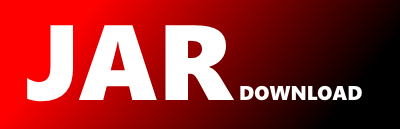
com.katalon.testops.api.model.ExecutionTestResultResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testops-api Show documentation
Show all versions of testops-api Show documentation
Katalon TestOps API Client generated by OpenAPI
The newest version!
/*
* Katalon TestOps API reference
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.katalon.testops.api.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.katalon.testops.api.model.ExecutionResource;
import com.katalon.testops.api.model.ExecutionTestResultIdentifyResource;
import com.katalon.testops.api.model.ExecutionTestSuiteResource;
import com.katalon.testops.api.model.ExternalIssueResource;
import com.katalon.testops.api.model.FileResource;
import com.katalon.testops.api.model.IncidentResource;
import com.katalon.testops.api.model.PlatformResource;
import com.katalon.testops.api.model.TestCaseResource;
import com.katalon.testops.api.model.TestSuiteResource;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import java.io.Serializable;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
/**
* ExecutionTestResultResource
*/
@JsonPropertyOrder({
ExecutionTestResultResource.JSON_PROPERTY_ID,
ExecutionTestResultResource.JSON_PROPERTY_TEST_CASE,
ExecutionTestResultResource.JSON_PROPERTY_EXECUTION,
ExecutionTestResultResource.JSON_PROPERTY_PLATFORM,
ExecutionTestResultResource.JSON_PROPERTY_STATUS,
ExecutionTestResultResource.JSON_PROPERTY_SAME_STATUS_PERIOD,
ExecutionTestResultResource.JSON_PROPERTY_ERROR_DETAILS_ID,
ExecutionTestResultResource.JSON_PROPERTY_STDOUT_ID,
ExecutionTestResultResource.JSON_PROPERTY_DESCRIPTION_ID,
ExecutionTestResultResource.JSON_PROPERTY_LOG_ID,
ExecutionTestResultResource.JSON_PROPERTY_ATTACHMENTS,
ExecutionTestResultResource.JSON_PROPERTY_START_TIME,
ExecutionTestResultResource.JSON_PROPERTY_END_TIME,
ExecutionTestResultResource.JSON_PROPERTY_DURATION,
ExecutionTestResultResource.JSON_PROPERTY_SAME_FAILURE_RESULTS,
ExecutionTestResultResource.JSON_PROPERTY_TEST_SUITE,
ExecutionTestResultResource.JSON_PROPERTY_EXECUTION_TEST_SUITE,
ExecutionTestResultResource.JSON_PROPERTY_INCIDENTS,
ExecutionTestResultResource.JSON_PROPERTY_PROFILE,
ExecutionTestResultResource.JSON_PROPERTY_HAS_COMMENT,
ExecutionTestResultResource.JSON_PROPERTY_ERROR_MESSAGE,
ExecutionTestResultResource.JSON_PROPERTY_ERROR_DETAIL,
ExecutionTestResultResource.JSON_PROPERTY_WEB_URL,
ExecutionTestResultResource.JSON_PROPERTY_EXTERNAL_ISSUES,
ExecutionTestResultResource.JSON_PROPERTY_FAILED_TEST_RESULT_CATEGORY,
ExecutionTestResultResource.JSON_PROPERTY_TOTAL_TEST_OBJECT,
ExecutionTestResultResource.JSON_PROPERTY_TOTAL_DEFECTS,
ExecutionTestResultResource.JSON_PROPERTY_URL_ID
})
@JsonTypeName("ExecutionTestResultResource")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public class ExecutionTestResultResource implements Serializable {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ID = "id";
private Long id;
public static final String JSON_PROPERTY_TEST_CASE = "testCase";
private TestCaseResource testCase;
public static final String JSON_PROPERTY_EXECUTION = "execution";
private ExecutionResource execution;
public static final String JSON_PROPERTY_PLATFORM = "platform";
private PlatformResource platform;
/**
* Gets or Sets status
*/
public enum StatusEnum {
PASSED("PASSED"),
FAILED("FAILED"),
ERROR("ERROR"),
INCOMPLETE("INCOMPLETE"),
RUNNING("RUNNING"),
SKIPPED("SKIPPED"),
NOT_RUN("NOT_RUN");
private String value;
StatusEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static StatusEnum fromValue(String value) {
for (StatusEnum b : StatusEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_STATUS = "status";
private StatusEnum status;
public static final String JSON_PROPERTY_SAME_STATUS_PERIOD = "sameStatusPeriod";
private Long sameStatusPeriod;
public static final String JSON_PROPERTY_ERROR_DETAILS_ID = "errorDetailsId";
private Long errorDetailsId;
public static final String JSON_PROPERTY_STDOUT_ID = "stdoutId";
private Long stdoutId;
public static final String JSON_PROPERTY_DESCRIPTION_ID = "descriptionId";
private Long descriptionId;
public static final String JSON_PROPERTY_LOG_ID = "logId";
private Long logId;
public static final String JSON_PROPERTY_ATTACHMENTS = "attachments";
private List attachments = null;
public static final String JSON_PROPERTY_START_TIME = "startTime";
private OffsetDateTime startTime;
public static final String JSON_PROPERTY_END_TIME = "endTime";
private OffsetDateTime endTime;
public static final String JSON_PROPERTY_DURATION = "duration";
private Long duration;
public static final String JSON_PROPERTY_SAME_FAILURE_RESULTS = "sameFailureResults";
private List sameFailureResults = null;
public static final String JSON_PROPERTY_TEST_SUITE = "testSuite";
private TestSuiteResource testSuite;
public static final String JSON_PROPERTY_EXECUTION_TEST_SUITE = "executionTestSuite";
private ExecutionTestSuiteResource executionTestSuite;
public static final String JSON_PROPERTY_INCIDENTS = "incidents";
private List incidents = null;
public static final String JSON_PROPERTY_PROFILE = "profile";
private String profile;
public static final String JSON_PROPERTY_HAS_COMMENT = "hasComment";
private Boolean hasComment;
public static final String JSON_PROPERTY_ERROR_MESSAGE = "errorMessage";
private String errorMessage;
public static final String JSON_PROPERTY_ERROR_DETAIL = "errorDetail";
private String errorDetail;
public static final String JSON_PROPERTY_WEB_URL = "webUrl";
private String webUrl;
public static final String JSON_PROPERTY_EXTERNAL_ISSUES = "externalIssues";
private List externalIssues = null;
/**
* Gets or Sets failedTestResultCategory
*/
public enum FailedTestResultCategoryEnum {
APPLICATION("APPLICATION"),
AUTOMATION("AUTOMATION"),
ENVIRONMENT("ENVIRONMENT"),
UNKNOWN("UNKNOWN");
private String value;
FailedTestResultCategoryEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static FailedTestResultCategoryEnum fromValue(String value) {
for (FailedTestResultCategoryEnum b : FailedTestResultCategoryEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_FAILED_TEST_RESULT_CATEGORY = "failedTestResultCategory";
private FailedTestResultCategoryEnum failedTestResultCategory;
public static final String JSON_PROPERTY_TOTAL_TEST_OBJECT = "totalTestObject";
private Long totalTestObject;
public static final String JSON_PROPERTY_TOTAL_DEFECTS = "totalDefects";
private Integer totalDefects;
public static final String JSON_PROPERTY_URL_ID = "urlId";
private String urlId;
public ExecutionTestResultResource id(Long id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public ExecutionTestResultResource testCase(TestCaseResource testCase) {
this.testCase = testCase;
return this;
}
/**
* Get testCase
* @return testCase
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TEST_CASE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public TestCaseResource getTestCase() {
return testCase;
}
public void setTestCase(TestCaseResource testCase) {
this.testCase = testCase;
}
public ExecutionTestResultResource execution(ExecutionResource execution) {
this.execution = execution;
return this;
}
/**
* Get execution
* @return execution
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_EXECUTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ExecutionResource getExecution() {
return execution;
}
public void setExecution(ExecutionResource execution) {
this.execution = execution;
}
public ExecutionTestResultResource platform(PlatformResource platform) {
this.platform = platform;
return this;
}
/**
* Get platform
* @return platform
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_PLATFORM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public PlatformResource getPlatform() {
return platform;
}
public void setPlatform(PlatformResource platform) {
this.platform = platform;
}
public ExecutionTestResultResource status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_STATUS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public ExecutionTestResultResource sameStatusPeriod(Long sameStatusPeriod) {
this.sameStatusPeriod = sameStatusPeriod;
return this;
}
/**
* Get sameStatusPeriod
* @return sameStatusPeriod
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SAME_STATUS_PERIOD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getSameStatusPeriod() {
return sameStatusPeriod;
}
public void setSameStatusPeriod(Long sameStatusPeriod) {
this.sameStatusPeriod = sameStatusPeriod;
}
public ExecutionTestResultResource errorDetailsId(Long errorDetailsId) {
this.errorDetailsId = errorDetailsId;
return this;
}
/**
* Get errorDetailsId
* @return errorDetailsId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ERROR_DETAILS_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getErrorDetailsId() {
return errorDetailsId;
}
public void setErrorDetailsId(Long errorDetailsId) {
this.errorDetailsId = errorDetailsId;
}
public ExecutionTestResultResource stdoutId(Long stdoutId) {
this.stdoutId = stdoutId;
return this;
}
/**
* Get stdoutId
* @return stdoutId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_STDOUT_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getStdoutId() {
return stdoutId;
}
public void setStdoutId(Long stdoutId) {
this.stdoutId = stdoutId;
}
public ExecutionTestResultResource descriptionId(Long descriptionId) {
this.descriptionId = descriptionId;
return this;
}
/**
* Get descriptionId
* @return descriptionId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DESCRIPTION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getDescriptionId() {
return descriptionId;
}
public void setDescriptionId(Long descriptionId) {
this.descriptionId = descriptionId;
}
public ExecutionTestResultResource logId(Long logId) {
this.logId = logId;
return this;
}
/**
* Get logId
* @return logId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_LOG_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getLogId() {
return logId;
}
public void setLogId(Long logId) {
this.logId = logId;
}
public ExecutionTestResultResource attachments(List attachments) {
this.attachments = attachments;
return this;
}
public ExecutionTestResultResource addAttachmentsItem(FileResource attachmentsItem) {
if (this.attachments == null) {
this.attachments = new ArrayList<>();
}
this.attachments.add(attachmentsItem);
return this;
}
/**
* Get attachments
* @return attachments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ATTACHMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAttachments() {
return attachments;
}
public void setAttachments(List attachments) {
this.attachments = attachments;
}
public ExecutionTestResultResource startTime(OffsetDateTime startTime) {
this.startTime = startTime;
return this;
}
/**
* Get startTime
* @return startTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_START_TIME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getStartTime() {
return startTime;
}
public void setStartTime(OffsetDateTime startTime) {
this.startTime = startTime;
}
public ExecutionTestResultResource endTime(OffsetDateTime endTime) {
this.endTime = endTime;
return this;
}
/**
* Get endTime
* @return endTime
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_END_TIME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getEndTime() {
return endTime;
}
public void setEndTime(OffsetDateTime endTime) {
this.endTime = endTime;
}
public ExecutionTestResultResource duration(Long duration) {
this.duration = duration;
return this;
}
/**
* Get duration
* @return duration
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DURATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getDuration() {
return duration;
}
public void setDuration(Long duration) {
this.duration = duration;
}
public ExecutionTestResultResource sameFailureResults(List sameFailureResults) {
this.sameFailureResults = sameFailureResults;
return this;
}
public ExecutionTestResultResource addSameFailureResultsItem(ExecutionTestResultIdentifyResource sameFailureResultsItem) {
if (this.sameFailureResults == null) {
this.sameFailureResults = new ArrayList<>();
}
this.sameFailureResults.add(sameFailureResultsItem);
return this;
}
/**
* Get sameFailureResults
* @return sameFailureResults
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_SAME_FAILURE_RESULTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getSameFailureResults() {
return sameFailureResults;
}
public void setSameFailureResults(List sameFailureResults) {
this.sameFailureResults = sameFailureResults;
}
public ExecutionTestResultResource testSuite(TestSuiteResource testSuite) {
this.testSuite = testSuite;
return this;
}
/**
* Get testSuite
* @return testSuite
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TEST_SUITE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public TestSuiteResource getTestSuite() {
return testSuite;
}
public void setTestSuite(TestSuiteResource testSuite) {
this.testSuite = testSuite;
}
public ExecutionTestResultResource executionTestSuite(ExecutionTestSuiteResource executionTestSuite) {
this.executionTestSuite = executionTestSuite;
return this;
}
/**
* Get executionTestSuite
* @return executionTestSuite
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_EXECUTION_TEST_SUITE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ExecutionTestSuiteResource getExecutionTestSuite() {
return executionTestSuite;
}
public void setExecutionTestSuite(ExecutionTestSuiteResource executionTestSuite) {
this.executionTestSuite = executionTestSuite;
}
public ExecutionTestResultResource incidents(List incidents) {
this.incidents = incidents;
return this;
}
public ExecutionTestResultResource addIncidentsItem(IncidentResource incidentsItem) {
if (this.incidents == null) {
this.incidents = new ArrayList<>();
}
this.incidents.add(incidentsItem);
return this;
}
/**
* Get incidents
* @return incidents
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_INCIDENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getIncidents() {
return incidents;
}
public void setIncidents(List incidents) {
this.incidents = incidents;
}
public ExecutionTestResultResource profile(String profile) {
this.profile = profile;
return this;
}
/**
* Get profile
* @return profile
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_PROFILE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getProfile() {
return profile;
}
public void setProfile(String profile) {
this.profile = profile;
}
public ExecutionTestResultResource hasComment(Boolean hasComment) {
this.hasComment = hasComment;
return this;
}
/**
* Get hasComment
* @return hasComment
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_HAS_COMMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getHasComment() {
return hasComment;
}
public void setHasComment(Boolean hasComment) {
this.hasComment = hasComment;
}
public ExecutionTestResultResource errorMessage(String errorMessage) {
this.errorMessage = errorMessage;
return this;
}
/**
* Get errorMessage
* @return errorMessage
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ERROR_MESSAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getErrorMessage() {
return errorMessage;
}
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
public ExecutionTestResultResource errorDetail(String errorDetail) {
this.errorDetail = errorDetail;
return this;
}
/**
* Get errorDetail
* @return errorDetail
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_ERROR_DETAIL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getErrorDetail() {
return errorDetail;
}
public void setErrorDetail(String errorDetail) {
this.errorDetail = errorDetail;
}
public ExecutionTestResultResource webUrl(String webUrl) {
this.webUrl = webUrl;
return this;
}
/**
* Get webUrl
* @return webUrl
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_WEB_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getWebUrl() {
return webUrl;
}
public void setWebUrl(String webUrl) {
this.webUrl = webUrl;
}
public ExecutionTestResultResource externalIssues(List externalIssues) {
this.externalIssues = externalIssues;
return this;
}
public ExecutionTestResultResource addExternalIssuesItem(ExternalIssueResource externalIssuesItem) {
if (this.externalIssues == null) {
this.externalIssues = new ArrayList<>();
}
this.externalIssues.add(externalIssuesItem);
return this;
}
/**
* Get externalIssues
* @return externalIssues
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_EXTERNAL_ISSUES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getExternalIssues() {
return externalIssues;
}
public void setExternalIssues(List externalIssues) {
this.externalIssues = externalIssues;
}
public ExecutionTestResultResource failedTestResultCategory(FailedTestResultCategoryEnum failedTestResultCategory) {
this.failedTestResultCategory = failedTestResultCategory;
return this;
}
/**
* Get failedTestResultCategory
* @return failedTestResultCategory
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_FAILED_TEST_RESULT_CATEGORY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public FailedTestResultCategoryEnum getFailedTestResultCategory() {
return failedTestResultCategory;
}
public void setFailedTestResultCategory(FailedTestResultCategoryEnum failedTestResultCategory) {
this.failedTestResultCategory = failedTestResultCategory;
}
public ExecutionTestResultResource totalTestObject(Long totalTestObject) {
this.totalTestObject = totalTestObject;
return this;
}
/**
* Get totalTestObject
* @return totalTestObject
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_TEST_OBJECT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getTotalTestObject() {
return totalTestObject;
}
public void setTotalTestObject(Long totalTestObject) {
this.totalTestObject = totalTestObject;
}
public ExecutionTestResultResource totalDefects(Integer totalDefects) {
this.totalDefects = totalDefects;
return this;
}
/**
* Get totalDefects
* @return totalDefects
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_DEFECTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getTotalDefects() {
return totalDefects;
}
public void setTotalDefects(Integer totalDefects) {
this.totalDefects = totalDefects;
}
public ExecutionTestResultResource urlId(String urlId) {
this.urlId = urlId;
return this;
}
/**
* Get urlId
* @return urlId
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_URL_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUrlId() {
return urlId;
}
public void setUrlId(String urlId) {
this.urlId = urlId;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExecutionTestResultResource executionTestResultResource = (ExecutionTestResultResource) o;
return Objects.equals(this.id, executionTestResultResource.id) &&
Objects.equals(this.testCase, executionTestResultResource.testCase) &&
Objects.equals(this.execution, executionTestResultResource.execution) &&
Objects.equals(this.platform, executionTestResultResource.platform) &&
Objects.equals(this.status, executionTestResultResource.status) &&
Objects.equals(this.sameStatusPeriod, executionTestResultResource.sameStatusPeriod) &&
Objects.equals(this.errorDetailsId, executionTestResultResource.errorDetailsId) &&
Objects.equals(this.stdoutId, executionTestResultResource.stdoutId) &&
Objects.equals(this.descriptionId, executionTestResultResource.descriptionId) &&
Objects.equals(this.logId, executionTestResultResource.logId) &&
Objects.equals(this.attachments, executionTestResultResource.attachments) &&
Objects.equals(this.startTime, executionTestResultResource.startTime) &&
Objects.equals(this.endTime, executionTestResultResource.endTime) &&
Objects.equals(this.duration, executionTestResultResource.duration) &&
Objects.equals(this.sameFailureResults, executionTestResultResource.sameFailureResults) &&
Objects.equals(this.testSuite, executionTestResultResource.testSuite) &&
Objects.equals(this.executionTestSuite, executionTestResultResource.executionTestSuite) &&
Objects.equals(this.incidents, executionTestResultResource.incidents) &&
Objects.equals(this.profile, executionTestResultResource.profile) &&
Objects.equals(this.hasComment, executionTestResultResource.hasComment) &&
Objects.equals(this.errorMessage, executionTestResultResource.errorMessage) &&
Objects.equals(this.errorDetail, executionTestResultResource.errorDetail) &&
Objects.equals(this.webUrl, executionTestResultResource.webUrl) &&
Objects.equals(this.externalIssues, executionTestResultResource.externalIssues) &&
Objects.equals(this.failedTestResultCategory, executionTestResultResource.failedTestResultCategory) &&
Objects.equals(this.totalTestObject, executionTestResultResource.totalTestObject) &&
Objects.equals(this.totalDefects, executionTestResultResource.totalDefects) &&
Objects.equals(this.urlId, executionTestResultResource.urlId);
}
@Override
public int hashCode() {
return Objects.hash(id, testCase, execution, platform, status, sameStatusPeriod, errorDetailsId, stdoutId, descriptionId, logId, attachments, startTime, endTime, duration, sameFailureResults, testSuite, executionTestSuite, incidents, profile, hasComment, errorMessage, errorDetail, webUrl, externalIssues, failedTestResultCategory, totalTestObject, totalDefects, urlId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExecutionTestResultResource {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" testCase: ").append(toIndentedString(testCase)).append("\n");
sb.append(" execution: ").append(toIndentedString(execution)).append("\n");
sb.append(" platform: ").append(toIndentedString(platform)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" sameStatusPeriod: ").append(toIndentedString(sameStatusPeriod)).append("\n");
sb.append(" errorDetailsId: ").append(toIndentedString(errorDetailsId)).append("\n");
sb.append(" stdoutId: ").append(toIndentedString(stdoutId)).append("\n");
sb.append(" descriptionId: ").append(toIndentedString(descriptionId)).append("\n");
sb.append(" logId: ").append(toIndentedString(logId)).append("\n");
sb.append(" attachments: ").append(toIndentedString(attachments)).append("\n");
sb.append(" startTime: ").append(toIndentedString(startTime)).append("\n");
sb.append(" endTime: ").append(toIndentedString(endTime)).append("\n");
sb.append(" duration: ").append(toIndentedString(duration)).append("\n");
sb.append(" sameFailureResults: ").append(toIndentedString(sameFailureResults)).append("\n");
sb.append(" testSuite: ").append(toIndentedString(testSuite)).append("\n");
sb.append(" executionTestSuite: ").append(toIndentedString(executionTestSuite)).append("\n");
sb.append(" incidents: ").append(toIndentedString(incidents)).append("\n");
sb.append(" profile: ").append(toIndentedString(profile)).append("\n");
sb.append(" hasComment: ").append(toIndentedString(hasComment)).append("\n");
sb.append(" errorMessage: ").append(toIndentedString(errorMessage)).append("\n");
sb.append(" errorDetail: ").append(toIndentedString(errorDetail)).append("\n");
sb.append(" webUrl: ").append(toIndentedString(webUrl)).append("\n");
sb.append(" externalIssues: ").append(toIndentedString(externalIssues)).append("\n");
sb.append(" failedTestResultCategory: ").append(toIndentedString(failedTestResultCategory)).append("\n");
sb.append(" totalTestObject: ").append(toIndentedString(totalTestObject)).append("\n");
sb.append(" totalDefects: ").append(toIndentedString(totalDefects)).append("\n");
sb.append(" urlId: ").append(toIndentedString(urlId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy