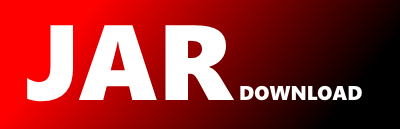
com.katujo.web.utils.JsonUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of katujo-web-utils Show documentation
Show all versions of katujo-web-utils Show documentation
Util classes for a Java web application with a SQL database back end that communicates with clients using JSON.
The newest version!
//Namespace
package com.katujo.web.utils;
//Java imports
import java.math.BigDecimal;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.util.concurrent.ConcurrentHashMap;
//Google imports
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonPrimitive;
/**
* JSON util methods that makes it easier to work with GSON/JSON in java.
* @author Johan Hertz
*
*/
public class JsonUtils
{
//The map that holds the translation from SQL column format to JSON field name
//
private static final ConcurrentHashMap createJsonObjectColumnTranslator = new ConcurrentHashMap();
/**
* Get the member value as an object.
* @param element
* @param member
* @return
* @throws Exception
*/
public static Object get(JsonElement element, String member) throws Exception
{
//Check if object
if(!element.isJsonObject())
throw new Exception("Element is not a JSON object");
//Get the object value for the member
return get(element.getAsJsonObject(), member);
}
/**
* Get the member value as an object.
* @param obj
* @param member
* @return
* @throws Exception
*/
public static Object get(JsonObject obj, String member) throws Exception
{
//The value is null
if(obj.get(member) == null || obj.get(member).isJsonNull())
return null;
//Get the primitive
JsonPrimitive primitive = obj.get(member).getAsJsonPrimitive();
//Boolean
if(primitive.isBoolean())
return primitive.getAsBoolean();
//Number/Double
if(primitive.isNumber())
return primitive.getAsDouble();
//String
if(primitive.isString())
return primitive.getAsString();
//Could not find a matching type
throw new Exception("The member type is not regonised");
}
/**
* Create a JSON array of JSON objects to hold the data in
* the result set.
* @param result
* @return
* @throws Exception
*/
public static JsonArray createJsonArray(ResultSet result) throws Exception
{
//Try to create the data
try
{
//Create the JSON array to hold the data
JsonArray data = new JsonArray();
//Read the result into the data
while(result.next())
data.add(createJsonObject(result));
//Return the data
return data;
}
//Failed
catch(Exception ex)
{
throw new Exception("Failed to create JSON array from result set", ex);
}
}
/**
* Create a JSON object from a result set row.
* @param result
* @return
* @throws Exception
*/
public static JsonObject createJsonObject(ResultSet result) throws Exception
{
//Try to create the JSON object
try
{
//Create the JSON object
JsonObject obj = new JsonObject();
//Get the meta data
ResultSetMetaData meta = result.getMetaData();
//Read the data into the object
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy