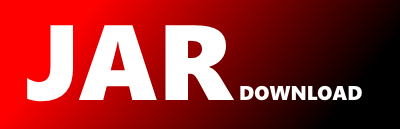
com.kauridev.lunarbase.GameSystemManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lunar-base Show documentation
Show all versions of lunar-base Show documentation
Lunar Base is a simple game framework written in Java. It is written using an entity-component-system architecture.
The newest version!
/*
* This file is part of the lunar-base package.
*
* Copyright (c) 2014 Eric Fritz
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software
* and associated documentation files (the "Software"), to deal in the Software without
* restriction, including without limitation the rights to use, copy, modify, merge, publish,
* distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the
* Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all copies or
* substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING
* BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
* DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.kauridev.lunarbase;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
/**
* A manager that handles a collection of systems.
*
* @author Eric Fritz
*/
public class GameSystemManager
{
/**
* Whether the manager has been initialized.
*/
private boolean isInitialized = false;
/**
* A map of systems by layer.
*/
private Map> systems = new TreeMap<>();
/**
* Adds a system to the list. If the manager has already been initialized, this system will be
* initialized immediately.
*
* @param system The system.
* @param layer The order this system should be processed in relation to other systems.
*/
public void addSystem(GameSystem system, int layer) {
if (isInitialized) {
system.init();
}
if (!systems.containsKey(layer)) {
systems.put(layer, new ArrayList());
}
systems.get(layer).add(system);
}
/**
* Initializes all the systems.
*/
public void init() {
for (List s : systems.values()) {
for (GameSystem system : s) {
system.init();
}
}
isInitialized = true;
}
/**
* Calls {@link GameSystem#exit()} on all the systems.
*/
public void exit() {
for (List s : systems.values()) {
for (GameSystem system : s) {
system.exit();
}
}
systems.clear();
}
/**
* Called when it has been determined that game logic needs to be processed.
*
* All systems will be processed in relation to their relative order (lower layers are processed
* first). Systems at the same layer will be processed in the order they were added to the
* manager.
*
* @param elapsed The number of milliseconds elapsed since the last call to process.
*/
public void process(double elapsed) {
for (List s : systems.values()) {
for (GameSystem system : s) {
system.process(elapsed);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy