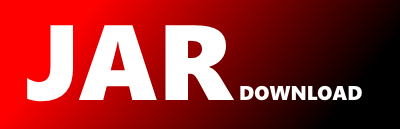
org.javabuilders.swing.mydoggy.MyDoggyContentWindow Maven / Gradle / Ivy
The newest version!
package org.javabuilders.swing.mydoggy;
import javax.swing.Icon;
/**
* Just a class to simplify the representation of a dock when you use Swing JavaBuilder and MyDoggy Docking
*
* @author anavarro
*
*/
public final class MyDoggyContentWindow
{
// mandatory
private String id;
private String title;
// optional
private Icon icon;
private Boolean closeable;
private Boolean detachable;
private Boolean minimizable;
private Boolean showAlwaysTab;
private String tabLayout;
private String tabPlacement;
/**
* @return the id
*/
public String getId()
{
return this.id;
}
/**
* @param aId
* the id to set
*/
public void setId(final String aId)
{
this.id = aId;
}
/**
* @return the title
*/
public String getTitle()
{
return this.title;
}
/**
* @param aTitle
* the title to set
*/
public void setTitle(final String aTitle)
{
this.title = aTitle;
}
/**
* Returns the icon.
*
* @return The icon to return.
*/
public Icon getIcon()
{
return this.icon;
}
/**
* Sets the icon.
*
* @param aIcon The icon to set.
*/
public void setIcon(final Icon aIcon)
{
this.icon = aIcon;
}
/**
* Returns the closeable.
*
* @return The closeable to return.
*/
public Boolean getCloseable()
{
return this.closeable;
}
/**
* Sets the closeable.
*
* @param aCloseable The closeable to set.
*/
public void setCloseable(final Boolean aCloseable)
{
this.closeable = aCloseable;
}
/**
* Returns the detachable.
*
* @return The detachable to return.
*/
public Boolean getDetachable()
{
return this.detachable;
}
/**
* Sets the detachable.
*
* @param aDetachable The detachable to set.
*/
public void setDetachable(final Boolean aDetachable)
{
this.detachable = aDetachable;
}
/**
* Returns the minimizable.
*
* @return The minimizable to return.
*/
public Boolean getMinimizable()
{
return this.minimizable;
}
/**
* Sets the minimizable.
*
* @param aMinimizable The minimizable to set.
*/
public void setMinimizable(final Boolean aMinimizable)
{
this.minimizable = aMinimizable;
}
/**
* Returns the showAlwaysTab.
*
* @return The showAlwaysTab to return.
*/
public Boolean getShowAlwaysTab()
{
return this.showAlwaysTab;
}
/**
* Sets the showAlwaysTab.
*
* @param aShowAlwaysTab The showAlwaysTab to set.
*/
public void setShowAlwaysTab(Boolean aShowAlwaysTab)
{
this.showAlwaysTab = aShowAlwaysTab;
}
/**
* Returns the tabLayout.
*
* @return The tabLayout to return.
*/
public String getTabLayout()
{
return this.tabLayout;
}
/**
* Sets the tabLayout.
*
* @param aTabLayout The tabLayout to set.
*/
public void setTabLayout(String aTabLayout)
{
this.tabLayout = aTabLayout;
}
/**
* Returns the tabPlacement.
*
* @return The tabPlacement to return.
*/
public String getTabPlacement()
{
return this.tabPlacement;
}
/**
* Sets the tabPlacement.
*
* @param aTabPlacement The tabPlacement to set.
*/
public void setTabPlacement(String aTabPlacement)
{
this.tabPlacement = aTabPlacement;
}
/**
* (non-Javadoc)
*
* @see java.lang.Object#toString()
*/
@Override
public String toString()
{
final StringBuilder stringBuilder = new StringBuilder(64);
stringBuilder.append("{id=").append(this.id).append(" ");
stringBuilder.append("title=").append(this.title).append("}");
return stringBuilder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy