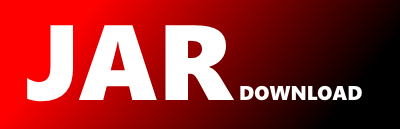
org.javabuilders.swing.mydoggy.MyDoggyToolWindow Maven / Gradle / Ivy
The newest version!
package org.javabuilders.swing.mydoggy;
import java.awt.Dimension;
import javax.swing.Icon;
/**
* Just a class to simplify the representation of a dock when you use Swing JavaBuilder and MyDoggy Docking
*
* @author anavarro
*
*/
public final class MyDoggyToolWindow
{
// Mandatory
private String id;
private String title;
private String anchor;
// Optional
private Icon icon;
private String tip;
private Boolean active;
private Boolean aggregateMode;
private Boolean autohide;
private Boolean available;
private Boolean flashing;
private Boolean maximized;
private Boolean representativeAnchorButtonVisible;
private Boolean selected;
private Boolean visible;
// Dock
private Long dockLength;
private Boolean dockHideRepresentativeButtonOnVisible;
private Long minimumDockLength;
private Boolean dockPopupMenuEnabled;
private Long dockPreviewDelay;
private Boolean dockPreviewEnabled;
private Double dockPreviewTransparentRatio;
// Sliding
private Long slidingTransparentDelay;
private Boolean slidingTransparentMode;
private Double slidingTransparentRatio;
// Floating
private Boolean floatingAddToTaskBar;
private Boolean floatingModal;
private Dimension floatingSize;
private Long floatingTransparentDelay;
private Boolean floatingTransparentMode;
private Double floatingTransparentRatio;
// Floating Free
private Boolean floatingFreeAddToTaskBar;
private Boolean floatingFreeModal;
private Dimension floatingFreeSize;
private Long floatingFreeTransparentDelay;
private Boolean floatingFreeTransparentMode;
private Double floatingFreeTransparentRatio;
// Floating Live
private Dimension floatingLiveSize;
private Long floatingLiveTransparentDelay;
private Boolean floatingLiveTransparentMode;
private Double floatingLiveTransparentRatio;
/**
* @return the id
*/
public String getId()
{
return this.id;
}
/**
* @param aId
* the id to set
*/
public void setId(final String aId)
{
this.id = aId;
}
/**
* @return the title
*/
public String getTitle()
{
return this.title;
}
/**
* @param aTitle
* the title to set
*/
public void setTitle(final String aTitle)
{
this.title = aTitle;
}
/**
* @return the icon
*/
public Icon getIcon()
{
return this.icon;
}
/**
* @param aIcon
* the icon to set
*/
public void setIcon(final Icon aIcon)
{
this.icon = aIcon;
}
/**
* @return the tip
*/
public String getTip()
{
return this.tip;
}
/**
* @param aTip
* the tip to set
*/
public void setTip(final String aTip)
{
this.tip = aTip;
}
/**
* @return the anchor
*/
public String getAnchor()
{
return this.anchor;
}
/**
* @param aAnchor
* the anchor to set
*/
public void setAnchor(final String aAnchor)
{
this.anchor = aAnchor;
}
/**
* Returns the active.
*
* @return The active to return.
*/
public Boolean getActive()
{
return this.active;
}
/**
* Sets the active.
*
* @param aActive
* The active to set.
*/
public void setActive(final Boolean aActive)
{
this.active = aActive;
}
/**
* Returns the aggregateMode.
*
* @return The aggregateMode to return.
*/
public Boolean getAggregateMode()
{
return this.aggregateMode;
}
/**
* Sets the aggregateMode.
*
* @param aAggregateMode
* The aggregateMode to set.
*/
public void setAggregateMode(final Boolean aAggregateMode)
{
this.aggregateMode = aAggregateMode;
}
/**
* Returns the autohide.
*
* @return The autohide to return.
*/
public Boolean getAutohide()
{
return this.autohide;
}
/**
* Sets the autohide.
*
* @param aAutohide
* The autohide to set.
*/
public void setAutohide(final Boolean aAutohide)
{
this.autohide = aAutohide;
}
/**
* Returns the available.
*
* @return The available to return.
*/
public Boolean getAvailable()
{
return this.available;
}
/**
* Sets the available.
*
* @param aAvailable
* The available to set.
*/
public void setAvailable(final Boolean aAvailable)
{
this.available = aAvailable;
}
/**
* Returns the flashing.
*
* @return The flashing to return.
*/
public Boolean getFlashing()
{
return this.flashing;
}
/**
* Sets the flashing.
*
* @param aFlashing
* The flashing to set.
*/
public void setFlashing(final Boolean aFlashing)
{
this.flashing = aFlashing;
}
/**
* Returns the maximized.
*
* @return The maximized to return.
*/
public Boolean getMaximized()
{
return this.maximized;
}
/**
* Sets the maximized.
*
* @param aMaximized
* The maximized to set.
*/
public void setMaximized(final Boolean aMaximized)
{
this.maximized = aMaximized;
}
/**
* Returns the representativeAnchorButtonVisible.
*
* @return The representativeAnchorButtonVisible to return.
*/
public Boolean getRepresentativeAnchorButtonVisible()
{
return this.representativeAnchorButtonVisible;
}
/**
* Sets the representativeAnchorButtonVisible.
*
* @param aRepresentativeAnchorButtonVisible
* The representativeAnchorButtonVisible to set.
*/
public void setRepresentativeAnchorButtonVisible(final Boolean aRepresentativeAnchorButtonVisible)
{
this.representativeAnchorButtonVisible = aRepresentativeAnchorButtonVisible;
}
/**
* Returns the selected.
*
* @return The selected to return.
*/
public Boolean getSelected()
{
return this.selected;
}
/**
* Sets the selected.
*
* @param aSelected
* The selected to set.
*/
public void setSelected(final Boolean aSelected)
{
this.selected = aSelected;
}
/**
* Returns the visible.
*
* @return The visible to return.
*/
public Boolean getVisible()
{
return this.visible;
}
/**
* Sets the visible.
*
* @param aVisible
* The visible to set.
*/
public void setVisible(final Boolean aVisible)
{
this.visible = aVisible;
}
/**
* Returns the dockLength.
*
* @return The dockLength to return.
*/
public Long getDockLength()
{
return this.dockLength;
}
/**
* Sets the dockLength.
*
* @param aDockLength The dockLength to set.
*/
public void setDockLength(final Long aDockLength)
{
this.dockLength = aDockLength;
}
/**
* Returns the dockHideRepresentativeButtonOnVisible.
*
* @return The dockHideRepresentativeButtonOnVisible to return.
*/
public Boolean getDockHideRepresentativeButtonOnVisible()
{
return this.dockHideRepresentativeButtonOnVisible;
}
/**
* Sets the dockHideRepresentativeButtonOnVisible.
*
* @param aDockHideRepresentativeButtonOnVisible The dockHideRepresentativeButtonOnVisible to set.
*/
public void setDockHideRepresentativeButtonOnVisible(final Boolean aDockHideRepresentativeButtonOnVisible)
{
this.dockHideRepresentativeButtonOnVisible = aDockHideRepresentativeButtonOnVisible;
}
/**
* Returns the minimumDockLength.
*
* @return The minimumDockLength to return.
*/
public Long getMinimumDockLength()
{
return this.minimumDockLength;
}
/**
* Sets the minimumDockLength.
*
* @param aMinimumDockLength The minimumDockLength to set.
*/
public void setMinimumDockLength(final Long aMinimumDockLength)
{
this.minimumDockLength = aMinimumDockLength;
}
/**
* Returns the dockPopupMenuEnabled.
*
* @return The dockPopupMenuEnabled to return.
*/
public Boolean getDockPopupMenuEnabled()
{
return this.dockPopupMenuEnabled;
}
/**
* Sets the dockPopupMenuEnabled.
*
* @param aDockPopupMenuEnabled The dockPopupMenuEnabled to set.
*/
public void setDockPopupMenuEnabled(final Boolean aDockPopupMenuEnabled)
{
this.dockPopupMenuEnabled = aDockPopupMenuEnabled;
}
/**
* Returns the dockPreviewDelay.
*
* @return The dockPreviewDelay to return.
*/
public Long getDockPreviewDelay()
{
return this.dockPreviewDelay;
}
/**
* Sets the dockPreviewDelay.
*
* @param aDockPreviewDelay The dockPreviewDelay to set.
*/
public void setDockPreviewDelay(final Long aDockPreviewDelay)
{
this.dockPreviewDelay = aDockPreviewDelay;
}
/**
* Returns the dockPreviewEnabled.
*
* @return The dockPreviewEnabled to return.
*/
public Boolean getDockPreviewEnabled()
{
return this.dockPreviewEnabled;
}
/**
* Sets the dockPreviewEnabled.
*
* @param aDockPreviewEnabled The dockPreviewEnabled to set.
*/
public void setDockPreviewEnabled(final Boolean aDockPreviewEnabled)
{
this.dockPreviewEnabled = aDockPreviewEnabled;
}
/**
* Returns the slidingTransparentDelay.
*
* @return The slidingTransparentDelay to return.
*/
public Long getSlidingTransparentDelay()
{
return this.slidingTransparentDelay;
}
/**
* Sets the slidingTransparentDelay.
*
* @param aSlidingTransparentDelay The slidingTransparentDelay to set.
*/
public void setSlidingTransparentDelay(final Long aSlidingTransparentDelay)
{
this.slidingTransparentDelay = aSlidingTransparentDelay;
}
/**
* Returns the slidingTransparentMode.
*
* @return The slidingTransparentMode to return.
*/
public Boolean getSlidingTransparentMode()
{
return this.slidingTransparentMode;
}
/**
* Sets the slidingTransparentMode.
*
* @param aSlidingTransparentMode The slidingTransparentMode to set.
*/
public void setSlidingTransparentMode(final Boolean aSlidingTransparentMode)
{
this.slidingTransparentMode = aSlidingTransparentMode;
}
/**
* Returns the floatingAddToTaskBar.
*
* @return The floatingAddToTaskBar to return.
*/
public Boolean getFloatingAddToTaskBar()
{
return this.floatingAddToTaskBar;
}
/**
* Sets the floatingAddToTaskBar.
*
* @param aFloatingAddToTaskBar The floatingAddToTaskBar to set.
*/
public void setFloatingAddToTaskBar(final Boolean aFloatingAddToTaskBar)
{
this.floatingAddToTaskBar = aFloatingAddToTaskBar;
}
/**
* Returns the floatingModal.
*
* @return The floatingModal to return.
*/
public Boolean getFloatingModal()
{
return this.floatingModal;
}
/**
* Sets the floatingModal.
*
* @param aFloatingModal The floatingModal to set.
*/
public void setFloatingModal(final Boolean aFloatingModal)
{
this.floatingModal = aFloatingModal;
}
/**
* Returns the floatingSize.
*
* @return The floatingSize to return.
*/
public Dimension getFloatingSize()
{
return this.floatingSize;
}
/**
* Sets the floatingSize.
*
* @param aFloatingSize The floatingSize to set.
*/
public void setFloatingSize(final Dimension aFloatingSize)
{
this.floatingSize = aFloatingSize;
}
/**
* Returns the floatingTransparentDelay.
*
* @return The floatingTransparentDelay to return.
*/
public Long getFloatingTransparentDelay()
{
return this.floatingTransparentDelay;
}
/**
* Sets the floatingTransparentDelay.
*
* @param aFloatingTransparentDelay The floatingTransparentDelay to set.
*/
public void setFloatingTransparentDelay(final Long aFloatingTransparentDelay)
{
this.floatingTransparentDelay = aFloatingTransparentDelay;
}
/**
* Returns the floatingTransparentMode.
*
* @return The floatingTransparentMode to return.
*/
public Boolean getFloatingTransparentMode()
{
return this.floatingTransparentMode;
}
/**
* Sets the floatingTransparentMode.
*
* @param aFloatingTransparentMode The floatingTransparentMode to set.
*/
public void setFloatingTransparentMode(final Boolean aFloatingTransparentMode)
{
this.floatingTransparentMode = aFloatingTransparentMode;
}
/**
* Returns the floatingFreeAddToTaskBar.
*
* @return The floatingFreeAddToTaskBar to return.
*/
public Boolean getFloatingFreeAddToTaskBar()
{
return this.floatingFreeAddToTaskBar;
}
/**
* Sets the floatingFreeAddToTaskBar.
*
* @param aFloatingFreeAddToTaskBar The floatingFreeAddToTaskBar to set.
*/
public void setFloatingFreeAddToTaskBar(final Boolean aFloatingFreeAddToTaskBar)
{
this.floatingFreeAddToTaskBar = aFloatingFreeAddToTaskBar;
}
/**
* Returns the floatingFreeModal.
*
* @return The floatingFreeModal to return.
*/
public Boolean getFloatingFreeModal()
{
return this.floatingFreeModal;
}
/**
* Sets the floatingFreeModal.
*
* @param aFloatingFreeModal The floatingFreeModal to set.
*/
public void setFloatingFreeModal(final Boolean aFloatingFreeModal)
{
this.floatingFreeModal = aFloatingFreeModal;
}
/**
* Returns the floatingFreeSize.
*
* @return The floatingFreeSize to return.
*/
public Dimension getFloatingFreeSize()
{
return this.floatingFreeSize;
}
/**
* Sets the floatingFreeSize.
*
* @param aFloatingFreeSize The floatingFreeSize to set.
*/
public void setFloatingFreeSize(final Dimension aFloatingFreeSize)
{
this.floatingFreeSize = aFloatingFreeSize;
}
/**
* Returns the floatingFreeTransparentDelay.
*
* @return The floatingFreeTransparentDelay to return.
*/
public Long getFloatingFreeTransparentDelay()
{
return this.floatingFreeTransparentDelay;
}
/**
* Sets the floatingFreeTransparentDelay.
*
* @param aFloatingFreeTransparentDelay The floatingFreeTransparentDelay to set.
*/
public void setFloatingFreeTransparentDelay(final Long aFloatingFreeTransparentDelay)
{
this.floatingFreeTransparentDelay = aFloatingFreeTransparentDelay;
}
/**
* Returns the floatingFreeTransparentMode.
*
* @return The floatingFreeTransparentMode to return.
*/
public Boolean getFloatingFreeTransparentMode()
{
return this.floatingFreeTransparentMode;
}
/**
* Sets the floatingFreeTransparentMode.
*
* @param aFloatingFreeTransparentMode The floatingFreeTransparentMode to set.
*/
public void setFloatingFreeTransparentMode(final Boolean aFloatingFreeTransparentMode)
{
this.floatingFreeTransparentMode = aFloatingFreeTransparentMode;
}
/**
* Returns the floatingLiveSize.
*
* @return The floatingLiveSize to return.
*/
public Dimension getFloatingLiveSize()
{
return this.floatingLiveSize;
}
/**
* Sets the floatingLiveSize.
*
* @param aFloatingLiveSize The floatingLiveSize to set.
*/
public void setFloatingLiveSize(final Dimension aFloatingLiveSize)
{
this.floatingLiveSize = aFloatingLiveSize;
}
/**
* Returns the floatingLiveTransparentDelay.
*
* @return The floatingLiveTransparentDelay to return.
*/
public Long getFloatingLiveTransparentDelay()
{
return this.floatingLiveTransparentDelay;
}
/**
* Sets the floatingLiveTransparentDelay.
*
* @param aFloatingLiveTransparentDelay The floatingLiveTransparentDelay to set.
*/
public void setFloatingLiveTransparentDelay(final Long aFloatingLiveTransparentDelay)
{
this.floatingLiveTransparentDelay = aFloatingLiveTransparentDelay;
}
/**
* Returns the floatingLiveTransparentMode.
*
* @return The floatingLiveTransparentMode to return.
*/
public Boolean getFloatingLiveTransparentMode()
{
return this.floatingLiveTransparentMode;
}
/**
* Sets the floatingLiveTransparentMode.
*
* @param aFloatingLiveTransparentMode The floatingLiveTransparentMode to set.
*/
public void setFloatingLiveTransparentMode(final Boolean aFloatingLiveTransparentMode)
{
this.floatingLiveTransparentMode = aFloatingLiveTransparentMode;
}
/**
* Returns the dockPreviewTransparentRatio.
*
* @return The dockPreviewTransparentRatio to return.
*/
public Double getDockPreviewTransparentRatio()
{
return this.dockPreviewTransparentRatio;
}
/**
* Sets the dockPreviewTransparentRatio.
*
* @param aDockPreviewTransparentRatio The dockPreviewTransparentRatio to set.
*/
public void setDockPreviewTransparentRatio(final Double aDockPreviewTransparentRatio)
{
this.dockPreviewTransparentRatio = aDockPreviewTransparentRatio;
}
/**
* Returns the slidingTransparentRatio.
*
* @return The slidingTransparentRatio to return.
*/
public Double getSlidingTransparentRatio()
{
return this.slidingTransparentRatio;
}
/**
* Sets the slidingTransparentRatio.
*
* @param aSlidingTransparentRatio The slidingTransparentRatio to set.
*/
public void setSlidingTransparentRatio(final Double aSlidingTransparentRatio)
{
this.slidingTransparentRatio = aSlidingTransparentRatio;
}
/**
* Returns the floatingTransparentRatio.
*
* @return The floatingTransparentRatio to return.
*/
public Double getFloatingTransparentRatio()
{
return this.floatingTransparentRatio;
}
/**
* Sets the floatingTransparentRatio.
*
* @param aFloatingTransparentRatio The floatingTransparentRatio to set.
*/
public void setFloatingTransparentRatio(final Double aFloatingTransparentRatio)
{
this.floatingTransparentRatio = aFloatingTransparentRatio;
}
/**
* Returns the floatingFreeTransparentRatio.
*
* @return The floatingFreeTransparentRatio to return.
*/
public Double getFloatingFreeTransparentRatio()
{
return this.floatingFreeTransparentRatio;
}
/**
* Sets the floatingFreeTransparentRatio.
*
* @param aFloatingFreeTransparentRatio The floatingFreeTransparentRatio to set.
*/
public void setFloatingFreeTransparentRatio(final Double aFloatingFreeTransparentRatio)
{
this.floatingFreeTransparentRatio = aFloatingFreeTransparentRatio;
}
/**
* Returns the floatingLiveTransparentRatio.
*
* @return The floatingLiveTransparentRatio to return.
*/
public Double getFloatingLiveTransparentRatio()
{
return this.floatingLiveTransparentRatio;
}
/**
* Sets the floatingLiveTransparentRatio.
*
* @param aFloatingLiveTransparentRatio The floatingLiveTransparentRatio to set.
*/
public void setFloatingLiveTransparentRatio(final Double aFloatingLiveTransparentRatio)
{
this.floatingLiveTransparentRatio = aFloatingLiveTransparentRatio;
}
/**
* (non-Javadoc)
*
* @see java.lang.Object#toString()
*/
@Override
public String toString()
{
final StringBuilder stringBuilder = new StringBuilder(64);
stringBuilder.append("{id=").append(this.id).append(" ");
stringBuilder.append("title=").append(this.title).append(" ");
stringBuilder.append("anchor=").append(this.anchor).append("}");
return stringBuilder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy