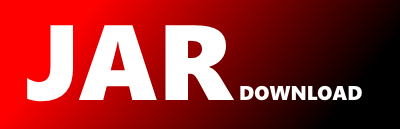
com.kendamasoft.dns.Buffer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dns-client Show documentation
Show all versions of dns-client Show documentation
Compact DNS client library intended primary for network utilities and testing applications
package com.kendamasoft.dns;
//import android.util.Log;
import java.util.ArrayList;
/**
* Byte buffer utility functions.
* Only for internal use.
*/
public class Buffer {
private byte[] data = new byte[DnsProtocol.MAX_MESSAGE_LENGTH];
private int length = 0;
private int mark = 0;
Buffer() {
data = new byte[DnsProtocol.MAX_MESSAGE_LENGTH];
}
Buffer(byte[] data) {
this.data = data;
this.length = data.length;
}
int getLength() {
return length;
}
byte[] getData() {
byte[] result = new byte[length];
System.arraycopy(data, 0, result, 0, length);
return result;
}
private void checkRange() {
if(mark >= length) {
throw new ArrayIndexOutOfBoundsException("Buffer overflow. Tried to read after it's end. Buffer length = " + length);
}
}
void write(DnsProtocol.Header header) {
write(header.transactionId);
write(header.flags);
write(header.questionResourceRecordCount);
write(header.answerResourceRecordsCount);
write(header.authorityResourceRecordsCount);
write(header.additionalResourceRecordsCount);
}
DnsProtocol.Header readHeader() {
DnsProtocol.Header header = new DnsProtocol.Header();
header.transactionId = readShort();
header.flags = readShort();
header.questionResourceRecordCount = readShort();
header.answerResourceRecordsCount = readShort();
header.authorityResourceRecordsCount = readShort();
header.additionalResourceRecordsCount = readShort();
return header;
}
void write(DnsProtocol.QuestionEntry questionEntry) {
write(questionEntry.name);
write(questionEntry.type);
write(questionEntry.questionClass);
}
DnsProtocol.QuestionEntry readQuestionEntry() {
DnsProtocol.QuestionEntry questionEntry = new DnsProtocol.QuestionEntry();
questionEntry.name = readString();
questionEntry.type = readShort();
questionEntry.questionClass = readShort();
return questionEntry;
}
DnsProtocol.ResourceRecord readResourceRecord() {
DnsProtocol.ResourceRecord record = new DnsProtocol.ResourceRecord();
record.name = readString();
record.recordTypeId = readShort();
record.recordType = DnsProtocol.RecordType.getById(record.recordTypeId);
record.recordClass = readShort();
record.ttl = readUint32();
record.dataLength = readShort();
record.readRecord(this);
return record;
}
void write(DnsProtocol.Message message) {
write(message.header);
write(message.questionEntry);
}
DnsProtocol.Message readMessage() {
DnsProtocol.Message message = new DnsProtocol.Message();
message.header = readHeader();
message.questionEntry = readQuestionEntry();
if(message.header.hasFlag(DnsProtocol.Header.FLAG_TRUNCATION)) {
return message;
}
if(message.header.answerResourceRecordsCount > 0) {
message.answerRecordList = new ArrayList(message.header.answerResourceRecordsCount);
for (int i = 0; i 0) {
message.authorityRecordList = new ArrayList(message.header.authorityResourceRecordsCount);
for (int i=0; i 0) {
message.additionalRecordList = new ArrayList(message.header.additionalResourceRecordsCount);
for (int i=0; i 0x7f) {
int next = readByte() & 0xff;
sb.append(readStringCompressed(((length ^ 192) << 8) | next));
break;
}
if(length == 0) {
break;
}
for (int i = 0; i < length; i++) {
sb.append((char)readByte());
}
sb.append('.');
}
return sb.toString();
}
private String readStringCompressed(int offset) {
int oldMark = mark;
mark = offset;
String result = readString();
mark = oldMark;
return result;
}
void write(byte b) {
data[length++] = b;
}
public byte readByte() {
checkRange();
return data[mark++];
}
void write(short s) {
data[length++] = (byte)(s >> 8);
data[length++] = (byte) s;
}
public short readShort() {
short b1 = (short)(readByte() & 0xff);
short b2 = (short)(readByte() & 0xff);
return (short)(b1 << 8 | b2);
}
public long readUint32() {
return ((readShort() & 0xFFFF) << 16) | (readShort() & 0xFFFF);
}
public void fill(byte[] data, int length) {
System.arraycopy(this.data, mark, data, 0, length);
advance(length);
}
void advance(int advance) {
mark += advance;
}
void dump() {
StringBuilder sb = new StringBuilder(">>>>>>>> DATA BUFFER DUMP <<<<<<<<\n");
for(int i = 0; i < data.length; i += 4) {
for(int j=i;j= data.length) {
break;
}
// ASCII printable [48,122]
if(data[j] >= 48 && data[j] <= 122) {
sb.append((char)data[j]);
} else {
sb.append(data[j] & 0xff);
}
sb.append(" ");
}
sb.append("\n");
}
sb.append(">>>>>>> DATA BUFFER DUMP END <<<<<<");
System.out.println(sb.toString());
//Log.d("DNS_DATA_BUFFER", sb.toString());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy