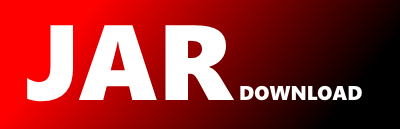
com.kenshoo.pl.data.CommandsExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of persistence-layer Show documentation
Show all versions of persistence-layer Show documentation
A Java persistence layer based on JOOQ for high performance and business flow support.
package com.kenshoo.pl.data;
import com.kenshoo.jooq.DataTable;
import com.kenshoo.jooq.FieldAndValue;
import com.kenshoo.pl.data.CreateRecordCommand.OnDuplicateKey;
import org.jooq.*;
import org.jooq.impl.DSL;
import java.util.*;
import java.util.stream.IntStream;
import java.util.stream.Stream;
import static java.util.stream.Collectors.toList;
import static java.util.stream.Collectors.toSet;
import static org.jooq.lambda.Seq.seq;
public class CommandsExecutor {
final private DSLContext dslContext;
public CommandsExecutor(DSLContext dslContext) {
this.dslContext = dslContext;
}
public static CommandsExecutor of(DSLContext dslContext) {
return new CommandsExecutor(dslContext);
}
public AffectedRows executeInserts(final DataTable table, Collection extends CreateRecordCommand> commands) {
return executeCommands(commands, homogeneousCommands -> executeInsertCommands(table, homogeneousCommands, OnDuplicateKey.FAIL));
}
public AffectedRows executeInsertsOnDuplicateKeyIgnore(final DataTable table, Collection extends CreateRecordCommand> commands) {
return executeCommands(commands, homogeneousCommands -> executeInsertCommands(table, homogeneousCommands, OnDuplicateKey.IGNORE));
}
public AffectedRows executeInsertsOnDuplicateKeyUpdate(final DataTable table, Collection extends CreateRecordCommand> commands) {
return executeCommands(commands, homogeneousCommands -> executeInsertCommands(table, homogeneousCommands, OnDuplicateKey.UPDATE));
}
public AffectedRows executeUpdates(final DataTable table, Collection extends UpdateRecordCommand> commands) {
return executeCommands(commands, homogeneousCommands -> executeUpdateCommands(table, homogeneousCommands));
}
public AffectedRows executeDeletes(final DataTable table, Collection extends DeleteRecordCommand> commands) {
if (commands.isEmpty()) {
return AffectedRows.empty();
}
return executeDeleteCommands(table, commands);
}
private AffectedRows executeCommands(Collection extends C> commands, HomogeneousChunkExecutor homogeneousChunkExecutor) {
AffectedRows updated = AffectedRows.empty();
List commandsLeft = new LinkedList<>(commands);
while (!commandsLeft.isEmpty()) {
C firstCommand = commandsLeft.remove(0);
List commandsToExecute = new ArrayList<>(Collections.singletonList(firstCommand));
Set firstCommandFields = getFieldsNames(firstCommand);
Iterator iterator = commandsLeft.iterator();
while (iterator.hasNext()) {
C command = iterator.next();
if (getFieldsNames(command).equals(firstCommandFields)) {
commandsToExecute.add(command);
iterator.remove();
}
}
updated = updated.plus(homogeneousChunkExecutor.execute(commandsToExecute));
}
return updated;
}
private AffectedRows executeDeleteCommands(DataTable table, Collection extends DeleteRecordCommand> commandsToExecute) {
DeleteWhereStep delete = dslContext.delete(table);
Iterator extends DeleteRecordCommand> commandIt = commandsToExecute.iterator();
DeleteRecordCommand command = commandIt.next();
Condition condition = DSL.trueCondition();
TableField[] tableFields = command.getId().getTableFields();
for (TableField id : tableFields) {
//noinspection unchecked
condition = condition.and(id.eq((Object) null));
}
for (FieldAndValue> partitionFieldAndValue : table.getVirtualPartition()) {
//noinspection unchecked
condition = condition.and(((Field) partitionFieldAndValue.getField()).eq((Object) null));
}
delete.where(condition);
BatchBindStep batch = dslContext.batch(delete);
while (command != null) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy