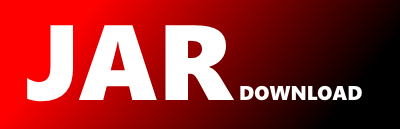
com.kenshoo.pl.entity.EntityField Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of persistence-layer Show documentation
Show all versions of persistence-layer Show documentation
A Java persistence layer based on JOOQ for high performance and business flow support.
package com.kenshoo.pl.entity;
import org.jooq.Record;
import org.jooq.TableField;
import org.jooq.lambda.Seq;
import java.util.Arrays;
import java.util.Objects;
import java.util.stream.Collectors;
public interface EntityField, T> {
EntityFieldDbAdapter getDbAdapter();
ValueConverter getStringValueConverter();
boolean valuesEqual(T v1, T v2);
default Class getValueClass() {
return getStringValueConverter().getValueClass();
}
default boolean isVirtual() {
return false;
}
EntityType getEntityType();
default PLCondition eq(T value) {
if (isVirtual()) {
throw new UnsupportedOperationException("The equals operation is unsupported for virtual fields");
}
final Object tableValue = getDbAdapter().getFirstDbValue(value);
@SuppressWarnings("unchecked")
final TableField tableField = (TableField)getDbAdapter().getFirstTableField();
return new PLCondition(tableField.eq(tableValue), entity -> entity.safeGet(this).equalsValue(value), this);
}
default PLCondition in(T ...values) {
if (isVirtual()) {
throw new UnsupportedOperationException("The in operation is unsupported for virtual fields");
}
final Object[] tableValues = Arrays.stream(values).map(value -> getDbAdapter().getFirstDbValue(value)).toArray(Object[]::new);
@SuppressWarnings("unchecked")
final TableField tableField = (TableField)getDbAdapter().getFirstTableField();
final var setOfValues = Seq.of(values).collect(Collectors.toUnmodifiableSet());
return new PLCondition(tableField.in(tableValues),
entity -> entity.safeGet(this).filter(Objects::nonNull).matches(setOfValues::contains), this);
}
default PLCondition isNull() {
if (isVirtual()) {
throw new UnsupportedOperationException("The equals operation is unsupported for virtual fields");
}
@SuppressWarnings("unchecked")
final TableField tableField = (TableField)getDbAdapter().getFirstTableField();
return new PLCondition(tableField.isNull(), entity -> entity.safeGet(this).isNull(), this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy