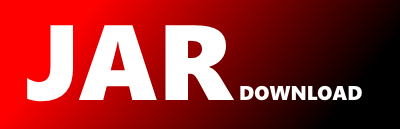
com.kenshoo.jooq.IdsTempTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of persistence-layer Show documentation
Show all versions of persistence-layer Show documentation
A Java persistence layer based on JOOQ for high performance and business flow support.
package com.kenshoo.jooq;
import org.jooq.DataType;
import org.jooq.Record;
import org.jooq.TableField;
import org.jooq.UniqueKey;
import org.jooq.impl.AbstractKeys;
import org.jooq.impl.SQLDataType;
import org.jooq.impl.TableImpl;
public class IdsTempTable extends TableImpl {
public static final IdsTempTable INT_TABLE = new IdsTempTable<>(SQLDataType.INTEGER);
public static final IdsTempTable LONG_TABLE = new IdsTempTable<>(SQLDataType.BIGINT);
public static final IdsTempTable STRING_SIZE_255_TABLE = new IdsTempTable<>(SQLDataType.VARCHAR.length(255));
public static final IdsTempTable STRING_SIZE_250_TABLE = new IdsTempTable<>(SQLDataType.VARCHAR.length(250));
public static final IdsTempTable STRING_SIZE_200_TABLE = new IdsTempTable<>(SQLDataType.VARCHAR.length(200));
public static final IdsTempTable STRING_SIZE_150_TABLE = new IdsTempTable<>(SQLDataType.VARCHAR.length(150));
public static final IdsTempTable STRING_IZE_100_TABLE = new IdsTempTable<>(SQLDataType.VARCHAR.length(100));
public static final IdsTempTable STRING_SIZE_50_TABLE = new IdsTempTable<>(SQLDataType.VARCHAR.length(50));
public static final IdsTempTable STRING_SIZE_40_TABLE = new IdsTempTable<>(SQLDataType.VARCHAR.length(40));
public static final IdsTempTable STRING_SIZE_30_TABLE = new IdsTempTable<>(SQLDataType.VARCHAR.length(30));
public static final IdsTempTable STRING_SIZE_20_TABLE = new IdsTempTable<>(SQLDataType.VARCHAR.length(20));
public static final IdsTempTable STRING_SIZE_10_TABLE = new IdsTempTable<>(SQLDataType.VARCHAR.length(10));
public final TableField id;
public IdsTempTable(DataType idType) {
super("tmp_ids");
id = createField("id", idType, this);
}
public IdsTempTable(String alias, IdsTempTable aliased) {
super(alias, null, aliased);
id = createField("id", aliased.id.getDataType(), this);
}
public IdsTempTable as(String alias) {
return new IdsTempTable<>(alias, this);
}
@Override
public UniqueKey getPrimaryKey() {
return new PrimaryKey().getPK();
}
private class PrimaryKey extends AbstractKeys {
@SuppressWarnings("unchecked")
UniqueKey getPK() {
return createUniqueKey(IdsTempTable.this, id);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy