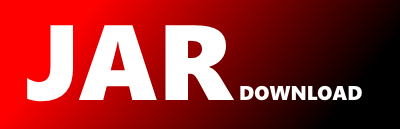
com.kenshoo.pl.entity.ChangeFlowConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of persistence-layer Show documentation
Show all versions of persistence-layer Show documentation
A Java persistence layer based on JOOQ for high performance and business flow support.
package com.kenshoo.pl.entity;
import com.google.common.annotations.VisibleForTesting;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableSet;
import com.kenshoo.pl.entity.internal.*;
import com.kenshoo.pl.entity.internal.audit.AuditedFieldsResolver;
import com.kenshoo.pl.entity.internal.audit.AuditRecordGenerator;
import com.kenshoo.pl.entity.spi.*;
import com.kenshoo.pl.entity.spi.helpers.EntityChangeCompositeValidator;
import com.kenshoo.pl.entity.spi.helpers.ImmutableFieldValidatorImpl;
import org.jooq.lambda.Seq;
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import static com.kenshoo.pl.entity.spi.PersistenceLayerRetryer.JUST_RUN_WITHOUT_CHECKING_DEADLOCKS;
import static java.util.stream.Collectors.toCollection;
public class ChangeFlowConfig> {
private final static Label NonExcludebale = new Label() {
};
private final E entityType;
private final List> postFetchCommandEnrichers;
private final List> outputGenerators;
private final List> validators;
private final Set> requiredRelationFields;
private final Set> requiredFields;
private final List>> childFlows;
private final List> postFetchFilters;
private final List> postSupplyFilters;
private final PersistenceLayerRetryer retryer;
private final AuditRecordGenerator auditRecordGenerator;
private final FeatureSet features;
private ChangeFlowConfig(E entityType,
List> postFetchCommandEnrichers,
List> validators,
List> outputGenerators,
Set> requiredRelationFields,
Set> requiredFields,
List>> childFlows,
PersistenceLayerRetryer retryer,
AuditRecordGenerator auditRecordGenerator,
FeatureSet features) {
this.entityType = entityType;
this.postFetchCommandEnrichers = postFetchCommandEnrichers;
this.outputGenerators = outputGenerators;
this.validators = validators;
this.requiredRelationFields = requiredRelationFields;
this.requiredFields = requiredFields;
this.childFlows = childFlows;
this.postFetchFilters = ImmutableList.of(new MissingParentEntitiesFilter<>(entityType.determineForeignKeys(requiredRelationFields)), new MissingEntitiesFilter<>(entityType));
this.postSupplyFilters = ImmutableList.of(new RequiredFieldsChangesFilter<>(requiredFields));
this.retryer = retryer;
this.auditRecordGenerator = auditRecordGenerator;
this.features = features;
}
public E getEntityType() {
return entityType;
}
public PersistenceLayerRetryer retryer() {
return retryer;
}
public Optional> auditRecordGenerator() {
return Optional.ofNullable(auditRecordGenerator);
}
public List> getPostFetchCommandEnrichers() {
return postFetchCommandEnrichers;
}
public List> getValidators() {
return validators;
}
public List> getOutputGenerators() {
return outputGenerators;
}
public Stream> currentStateConsumers() {
return Seq.concat(postFetchFilters,
postSupplyFilters,
postFetchCommandEnrichers,
validators,
outputGenerators)
.concat(Optional.ofNullable(auditRecordGenerator));
}
static > Builder builder(E entityType) {
return new Builder<>(entityType);
}
public Set> getRequiredRelationFields() {
return requiredRelationFields;
}
public Set> getRequiredFields() {
return requiredFields;
}
public List>> childFlows() {
return childFlows;
}
public List> getPostFetchFilters() {
return postFetchFilters;
}
public List> getPostSupplyFilters() {
return postSupplyFilters;
}
public Optional> getPrimaryIdentityField() {
return getEntityType().getPrimaryIdentityField();
}
public FeatureSet getFeatures() {
return this.features;
}
public static class Builder> {
private final E entityType;
private final List>> postFetchCommandEnrichers = new ArrayList<>();
private final List>> validators = new ArrayList<>();
private final List> outputGenerators = new ArrayList<>();
private final Set> requiredRelationFields = new HashSet<>();
private final Set> requiredFields = new HashSet<>();
private Optional> falseUpdatesPurger = Optional.empty();
private final List>> flowConfigBuilders = new ArrayList<>();
private PersistenceLayerRetryer retryer = JUST_RUN_WITHOUT_CHECKING_DEADLOCKS;
private final AuditedFieldsResolver auditedFieldsResolver;
private FeatureSet features = FeatureSet.EMPTY;
public Builder(E entityType) {
this(entityType,
AuditedFieldsResolver.INSTANCE);
}
@VisibleForTesting
Builder(final E entityType,
final AuditedFieldsResolver auditedFieldsResolver) {
this.entityType = entityType;
this.auditedFieldsResolver = auditedFieldsResolver;
}
public Builder with(FeatureSet features) {
this.features = features;
this.flowConfigBuilders.forEach(builder -> builder.with(features));
return this;
}
public Builder withLabeledPostFetchCommandEnricher(PostFetchCommandEnricher enricher, Label label) {
postFetchCommandEnrichers.add(new Labeled<>(enricher, label));
return this;
}
public Builder withPostFetchCommandEnricher(PostFetchCommandEnricher enricher) {
postFetchCommandEnrichers.add(new Labeled<>(enricher, NonExcludebale));
return this;
}
public Builder withLabeledPostFetchCommandEnrichers(Collection extends PostFetchCommandEnricher> enrichers, Label label) {
enrichers.forEach(e -> postFetchCommandEnrichers.add(new Labeled<>(e, label)));
return this;
}
public Builder withPostFetchCommandEnrichers(Collection extends PostFetchCommandEnricher> enrichers) {
enrichers.forEach(e -> postFetchCommandEnrichers.add(new Labeled<>(e, NonExcludebale)));
return this;
}
/* not public */ void withFalseUpdatesPurger(FalseUpdatesPurger falseUpdatesPurger) {
this.falseUpdatesPurger = Optional.of(falseUpdatesPurger);
}
public Builder withoutFalseUpdatesPurger() {
this.falseUpdatesPurger = Optional.empty();
this.flowConfigBuilders.forEach(Builder::withoutFalseUpdatesPurger);
return this;
}
public Builder withValidator(ChangesValidator validator) {
this.validators.add(new Labeled<>(validator, NonExcludebale));
return this;
}
public Builder withValidators(Collection> validators) {
validators.forEach(validator -> this.validators.add(new Labeled<>(validator, NonExcludebale)));
return this;
}
public Builder withLabeledValidator(ChangesValidator validator, Label label) {
this.validators.add(new Labeled<>(validator, label));
return this;
}
public Builder withLabeledValidators(Collection> validators, Label label) {
validators.forEach(validator -> this.validators.add(new Labeled<>(validator, label)));
return this;
}
public Builder withoutValidators() {
this.validators.clear();
this.flowConfigBuilders.forEach(Builder::withoutValidators);
return this;
}
public Builder withoutLabeledElements(Label label) {
this.withoutLabeledElements(ImmutableList.of(label));
return this;
}
public Builder withoutLabeledElements(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy