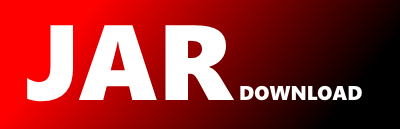
com.kenshoo.pl.entity.internal.fetch.QueryBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of persistence-layer Show documentation
Show all versions of persistence-layer Show documentation
A Java persistence layer based on JOOQ for high performance and business flow support.
package com.kenshoo.pl.entity.internal.fetch;
import com.google.common.collect.Lists;
import com.google.common.collect.Sets;
import com.kenshoo.jooq.*;
import com.kenshoo.pl.data.ImpersonatorTable;
import com.kenshoo.pl.entity.*;
import com.kenshoo.pl.entity.UniqueKey;
import org.jooq.*;
import org.jooq.impl.DSL;
import java.util.*;
import java.util.function.Consumer;
import java.util.function.Predicate;
import static org.jooq.lambda.function.Functions.not;
public class QueryBuilder> {
private DSLContext dslContext;
private List> selectedFields;
private DataTable startingTable;
private List paths;
private Set oneToOneTableRelations;
private Collection extends Identifier> ids;
public QueryBuilder(DSLContext dslContext) {
this.dslContext = dslContext;
}
public QueryBuilder selecting(List> selectedFields) {
this.selectedFields = selectedFields;
return this;
}
public QueryBuilder from(DataTable primaryTable) {
this.startingTable = primaryTable;
return this;
}
public QueryBuilder innerJoin(List paths) {
this.paths = paths;
return this;
}
public QueryBuilder innerJoin(TreeEdge path) {
this.paths = Lists.newArrayList(path);
return this;
}
public QueryBuilder leftJoin(Set oneToOneTableRelations) {
this.oneToOneTableRelations = oneToOneTableRelations;
return this;
}
public QueryBuilder whereIdsIn(Collection extends Identifier> ids) {
this.ids = ids;
return this;
}
public QueryExtension> build() {
final SelectJoinStep query = dslContext.select(selectedFields).from(startingTable);
final Set joinedTables = Sets.newHashSet(startingTable);
paths.forEach(edge -> joinTables(query, joinedTables, edge));
if (oneToOneTableRelations != null) {
joinSecondaryTables(query, joinedTables, oneToOneTableRelations);
}
if (ids != null) {
final UniqueKey uniqueKey = ids.iterator().next().getUniqueKey();
return addIdsCondition(query, startingTable, uniqueKey, ids);
}
return new QueryExtension>() {
@Override
public SelectJoinStep getQuery() {
return query;
}
@Override
public void close() {
}
};
}
public QueryExtension addIdsCondition(Q query, DataTable primaryTable, UniqueKey uniqueKey, Collection extends Identifier> identifiers) {
List> conditions = new ArrayList<>();
for (EntityField field : uniqueKey.getFields()) {
addToConditions(field, identifiers, conditions);
}
primaryTable.getVirtualPartition().forEach(fieldAndValue -> {
Object[] values = new Object[identifiers.size()];
Arrays.fill(values, fieldAndValue.getValue());
conditions.add(new FieldAndValues<>((Field
© 2015 - 2025 Weber Informatics LLC | Privacy Policy