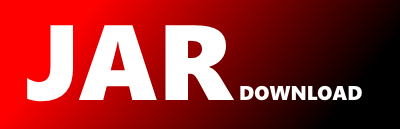
com.kenshoo.pl.entity.spi.helpers.CopyFieldsOnCreateEnricher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of persistence-layer Show documentation
Show all versions of persistence-layer Show documentation
A Java persistence layer based on JOOQ for high performance and business flow support.
package com.kenshoo.pl.entity.spi.helpers;
import com.kenshoo.pl.entity.*;
import com.kenshoo.pl.entity.spi.PostFetchCommandEnricher;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.stream.Stream;
public class CopyFieldsOnCreateEnricher> implements PostFetchCommandEnricher {
final List> fields2Copy = new ArrayList<>();
protected final Set> requiredFields = new HashSet<>();
public CopyFieldsOnCreateEnricher(EntityField, T> sourceField, EntityField targetField) {
//noinspection unchecked
this(new Field2Copy<>(sourceField, targetField));
}
public CopyFieldsOnCreateEnricher(EntityField, T1> sourceField1, EntityField targetField1,
EntityField, T2> sourceField2, EntityField targetField2) {
//noinspection unchecked
this(new Field2Copy<>(sourceField1, targetField1), new Field2Copy<>(sourceField2, targetField2));
}
public CopyFieldsOnCreateEnricher(EntityField, T1> sourceField1, EntityField targetField1,
EntityField, T2> sourceField2, EntityField targetField2,
EntityField, T3> sourceField3, EntityField targetField3) {
//noinspection unchecked
this(new Field2Copy<>(sourceField1, targetField1),
new Field2Copy<>(sourceField2, targetField2),
new Field2Copy<>(sourceField3, targetField3));
}
public CopyFieldsOnCreateEnricher(EntityField, T1> sourceField1, EntityField targetField1,
EntityField, T2> sourceField2, EntityField targetField2,
EntityField, T3> sourceField3, EntityField targetField3,
EntityField, T4> sourceField4, EntityField targetField4) {
//noinspection unchecked
this(new Field2Copy<>(sourceField1, targetField1),
new Field2Copy<>(sourceField2, targetField2),
new Field2Copy<>(sourceField3, targetField3),
new Field2Copy<>(sourceField4, targetField4));
}
@SuppressWarnings("unchecked")
private CopyFieldsOnCreateEnricher(Field2Copy... fieldsToCopy) {
this.fields2Copy.addAll(Arrays.asList(fieldsToCopy));
addRequiredFields();
}
protected void addRequiredFields() {
this.fields2Copy.stream()
.map(input -> input.source)
.forEach(requiredFields::add);
}
@Override
public SupportedChangeOperation getSupportedChangeOperation() {
return SupportedChangeOperation.CREATE;
}
@Override
public void enrich(Collection extends ChangeEntityCommand> commands, ChangeOperation changeOperation, ChangeContext changeContext) {
for (ChangeEntityCommand command : commands) {
Entity entity = changeContext.getEntity(command);
for (Field2Copy field2Copy : fields2Copy) {
copyField(field2Copy, entity, command);
}
}
}
@Override
public Stream> fieldsToEnrich() {
return fields2Copy.stream().map(pair -> pair.target);
}
private void copyField(Field2Copy field2Copy, Entity entity, ChangeEntityCommand command) {
command.set(field2Copy.target, entity.get(field2Copy.source));
}
@Override
public Stream extends EntityField, ?>> requiredFields(Collection extends EntityField> fieldsToUpdate, ChangeOperation changeOperation) {
return requiredFields.stream();
}
public static class Field2Copy, T> {
private final EntityField, T> source;
private final EntityField target;
Field2Copy(EntityField, T> source, EntityField target) {
this.source = source;
this.target = target;
}
public EntityField getTarget() {
return target;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy