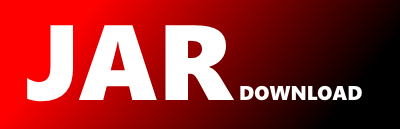
com.kenshoo.pl.entity.UniqueKey Maven / Gradle / Ivy
Show all versions of persistence-layer Show documentation
package com.kenshoo.pl.entity;
import com.google.common.collect.ImmutableList;
import org.jooq.Record;
import org.jooq.TableField;
import java.util.Arrays;
import java.util.List;
import static org.jooq.lambda.Seq.seq;
/**
* Specifies UniqueKey of entry.
* Since the key is used in maps, it's highly recommended to reuse the value of this class.
*
* The instances of this class are immutable.
*/
public class UniqueKey> {
private final EntityField[] fields;
private final TableField[] tableFields;
private int hashCode;
public UniqueKey(EntityField[] fields) {
this.fields = fields;
//noinspection unchecked
this.tableFields = Arrays.asList(fields).stream()
.flatMap(field -> field.getDbAdapter().getTableFields())
.toArray(TableField[]::new);
}
public UniqueKey(Iterable extends EntityField> fields) {
this(seq(fields).toArray(EntityField[]::new));
}
public EntityField[] getFields() {
return fields;
}
public List> getTableFields() {
//noinspection unchecked
return ImmutableList.copyOf(tableFields);
}
public Identifier createValue(FieldsValueMap fieldsValueMap) {
Object[] values = new Object[fields.length];
for (int i = 0; i < fields.length; i++) {
values[i] = fieldsValueMap.get(fields[i]);
}
return new UniqueKeyValue<>(this, values);
}
public E getEntityType() {
if(this.fields.length == 0) {
throw new IllegalStateException("unique key does not contain any fields.");
}
return (E) this.fields[0].getEntityType();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof UniqueKey)) return false;
UniqueKey uniqueKey = (UniqueKey) o;
return Arrays.deepEquals(fields, uniqueKey.fields);
}
@Override
public int hashCode() {
if (hashCode == 0) {
hashCode = Arrays.deepHashCode(fields);
}
return hashCode;
}
@Override
public String toString() {
return "UniqueKey{" +
"fields=" + Arrays.toString(fields) +
'}';
}
}