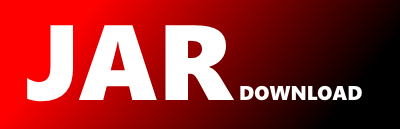
com.kenshoo.pl.entity.internal.SecondaryTableRelationExtractor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of persistence-layer Show documentation
Show all versions of persistence-layer Show documentation
A Java persistence layer based on JOOQ for high performance and business flow support.
package com.kenshoo.pl.entity.internal;
import com.kenshoo.jooq.DataTable;
import com.kenshoo.pl.entity.EntityField;
import com.kenshoo.pl.entity.EntityType;
import com.kenshoo.pl.entity.converters.IdentityValueConverter;
import org.jooq.Record;
import org.jooq.TableField;
import java.util.Objects;
import java.util.stream.Stream;
import static org.jooq.lambda.Seq.seq;
class SecondaryTableRelationExtractor {
/**
* This usually results with creating "fake" temporary EntityField because it is not likely that the Entity
* defines such an entity field.
* For example:
*
* ----- Table fields -----
*
* table campaign {
* int id;
* }
*
* table campaign_secondary {
* int campaign_id; // FK to campaign
* }
*
* ----- Entity fields -----
*
* class CampaignEntity {
* //
* // This is obvious:
* //
* static final EntityField ID = field(campaign.id);
* //
* // This is very unlikely:
* //
* static final EntityField ID_OF_PRIMARY_LOCATED_ON_SECONDARY_TABLE = field(campaign_secondary.campaign_id);
* }
*
* This method will create the unlikely EntityField assuming we can use it for fetching.
*/
static > Stream> relationUsingTableFieldsOfSecondary(DataTable secondaryTable, E entityType) {
var foreignKey = secondaryTable.getForeignKey(entityType.getPrimaryTable());
var primaryAndSecondaryFieldPairs = seq(foreignKey.getKey().getFields()).zip(foreignKey.getFields());
return primaryAndSecondaryFieldPairs
.map(ps -> entityType.findField(ps.v2).orElseGet(() -> createTemporaryEntityField(entityType, ps.v2, ps.v1)));
}
private static > EntityField createTemporaryEntityField(E entityType, TableField secondaryField, TableField, ?> primaryTableField) {
var primaryField = entityType.findField(primaryTableField).get();
var converter = IdentityValueConverter.getInstance(secondaryField.getType());
return new EntityFieldImpl(entityType, new SimpleEntityFieldDbAdapter<>(secondaryField, converter), primaryField.getStringValueConverter(), Objects::equals);
}
static > Stream extends EntityField> relationUsingTableFieldsOfPrimary(DataTable secondaryTable, E entityType) {
var foreignKey = secondaryTable.getForeignKey(entityType.getPrimaryTable());
var primaryFields = foreignKey.getKey().getFields();
return seq(primaryFields)
.map(field -> entityType.findField(field)
.orElseThrow(() -> new IllegalStateException(String.format("field %s is a FK from table %s to %s but is not defined on entity type %s.", field, secondaryTable.getName(), entityType.getPrimaryTable().getName(), entityType.getName()))));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy