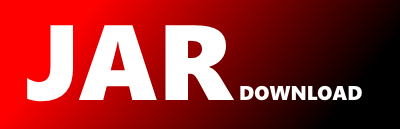
com.kenshoo.pl.data.ImpersonatorTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of persistence-layer Show documentation
Show all versions of persistence-layer Show documentation
A Java persistence layer based on JOOQ for high performance and business flow support.
package com.kenshoo.pl.data;
import com.google.common.base.Function;
import com.google.common.base.Predicate;
import com.google.common.base.Predicates;
import com.google.common.collect.Collections2;
import com.google.common.collect.Iterables;
import com.google.common.collect.Lists;
import com.kenshoo.jooq.DataTable;
import com.kenshoo.jooq.FieldAndValue;
import org.apache.commons.lang3.ArrayUtils;
import org.jooq.Field;
import org.jooq.ForeignKey;
import org.jooq.Record;
import org.jooq.TableField;
import org.jooq.UniqueKey;
import org.jooq.impl.AbstractKeys;
import org.jooq.impl.TableImpl;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
public class ImpersonatorTable extends TableImpl implements DataTable {
public static final String TEMP_TABLE_PREFIX = "tmp_";
private final Keys keys = new Keys();
private final DataTable realTable;
public ImpersonatorTable(DataTable realTable) {
super(TEMP_TABLE_PREFIX + realTable.getName());
this.realTable = realTable;
}
public TableField createField(Field realTableField) {
return createField(realTableField.getName(), realTableField.getDataType());
}
@Override
public Collection> getVirtualPartition() {
return Collections2.transform(realTable.getVirtualPartition(), new Function, FieldAndValue>>() {
@Override
public FieldAndValue> apply(FieldAndValue> input) {
return transform(input);
}
private FieldAndValue transform(FieldAndValue input) {
return new FieldAndValue<>(getField(input.getField()), input.getValue());
}
});
}
@Override
public UniqueKey getPrimaryKey() {
return keys.createPK();
}
@Override
public List> getReferences() {
// Transform is going to return nulls for foreign keys composed of fields not present in impersonator table,
// filtering them out
return Lists.newArrayList(Collections2.filter(Lists.transform(realTable.getReferences(), new Function, ForeignKey>() {
@Override
public ForeignKey apply(ForeignKey foreignKey) {
return keys.createFK(foreignKey);
}
}), Predicates.notNull()));
}
public TableField getField(final TableField realTableField) {
//noinspection unchecked
return (TableField) Iterables.tryFind(Arrays.asList(fields()), new Predicate>() {
@Override
public boolean apply(Field> field) {
return field.getName().equals(realTableField.getName());
}
}).orNull();
}
private class Keys extends AbstractKeys {
public UniqueKey createPK() {
UniqueKey primaryKey = realTable.getPrimaryKey();
if (primaryKey == null) {
return null;
}
TableField[] transformedFields = transformFields(primaryKey.getFieldsArray());
if (ArrayUtils.contains(transformedFields, null)) {
// We don't have some of the fields of realTable PK
return null;
}
//noinspection unchecked
return createUniqueKey(ImpersonatorTable.this, transformedFields);
}
public ForeignKey createFK(ForeignKey foreignKey) {
TableField[] fields = transformFields(foreignKey.getFieldsArray());
if (ArrayUtils.contains(fields, null)) {
return null;
}
//noinspection unchecked
return createForeignKey(foreignKey.getKey(), foreignKey.getTable(), fields);
}
private TableField[] transformFields(TableField[] originalFields) {
//noinspection unchecked
TableField[] fields = new TableField[originalFields.length];
for (int i = 0; i < originalFields.length; i++) {
TableField recordTableField = originalFields[i];
fields[i] = getField(recordTableField);
}
return fields;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy