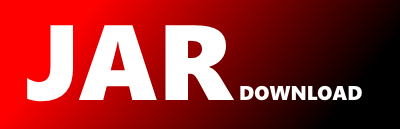
com.kenshoo.pl.entity.Identifier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of persistence-layer Show documentation
Show all versions of persistence-layer Show documentation
A Java persistence layer based on JOOQ for high performance and business flow support.
package com.kenshoo.pl.entity;
import com.kenshoo.jooq.FieldAndValues;
import org.jooq.Record;
import org.jooq.TableField;
import org.jooq.lambda.Seq;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.stream.Stream;
import static java.util.stream.Collectors.toList;
public interface Identifier> extends FieldsValueMap {
IdentifierType getUniqueKey();
default Stream
© 2015 - 2025 Weber Informatics LLC | Privacy Policy