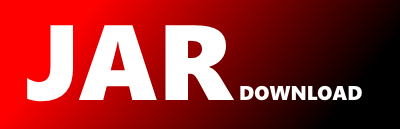
com.kenshoo.pl.entity.internal.ChangesContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of persistence-layer Show documentation
Show all versions of persistence-layer Show documentation
A Java persistence layer based on JOOQ for high performance and business flow support.
package com.kenshoo.pl.entity.internal;
import com.kenshoo.jooq.DataTable;
import com.kenshoo.pl.data.AbstractRecordCommand;
import com.kenshoo.pl.data.AffectedRows;
import com.kenshoo.pl.data.CommandsExecutor;
import com.kenshoo.pl.data.CreateRecordCommand;
import com.kenshoo.pl.data.DeleteRecordCommand;
import com.kenshoo.pl.data.UpdateRecordCommand;
import com.kenshoo.pl.entity.EntityChange;
import com.kenshoo.pl.entity.PersistentLayerStats;
import java.util.*;
import java.util.function.Supplier;
public class ChangesContainer {
private final CreateRecordCommand.OnDuplicateKey onDuplicateKey;
private final Map> deletes = new HashMap<>();
private final Map> updates = new HashMap<>();
private final Map> inserts = new HashMap<>();
private final Map> insertsOnDuplicateUpdate = new HashMap<>();
public ChangesContainer(CreateRecordCommand.OnDuplicateKey onDuplicateKey) {
this.onDuplicateKey = onDuplicateKey;
}
public AbstractRecordCommand getDelete(DataTable table, EntityChange entityChange, Supplier commandCreator) {
//noinspection RedundantTypeArguments IntelliJ fails to compile without specification
return getOrCreate(deletes, IdToCommandMap< DeleteRecordCommand>::new, commandCreator, table, entityChange);
}
public AbstractRecordCommand getUpdate(DataTable table, EntityChange entityChange, Supplier commandCreator) {
//noinspection RedundantTypeArguments IntelliJ fails to compile without specification
return getOrCreate(updates, IdToCommandMap< UpdateRecordCommand>::new, commandCreator, table, entityChange);
}
public AbstractRecordCommand getInsert(DataTable table, EntityChange entityChange, Supplier commandCreator) {
//noinspection RedundantTypeArguments IntelliJ fails to compile without specification
return getOrCreate(inserts, IdToCommandMap::new, commandCreator, table, entityChange);
}
public Optional getInsert(final DataTable table, final EntityChange entityChange) {
return Optional.ofNullable(inserts.get(table))
.map(idToCmdMap -> idToCmdMap.get(entityChange));
}
public AbstractRecordCommand getInsertOnDuplicateUpdate(DataTable table, EntityChange entityChange, Supplier commandCreator) {
//noinspection RedundantTypeArguments IntelliJ fails to compile without specification
return getOrCreate(insertsOnDuplicateUpdate, IdToCommandMap::new, commandCreator, table, entityChange);
}
public void commit(CommandsExecutor commandsExecutor, PersistentLayerStats stats) {
for (Map.Entry> entry : deletes.entrySet()) {
DataTable table = entry.getKey();
AffectedRows affectedRows = commandsExecutor.executeDeletes(table, entry.getValue().map.values());
stats.addAffectedRows(table.getName(), affectedRows);
}
for (Map.Entry> entry : inserts.entrySet()) {
DataTable table = entry.getKey();
Collection commands = entry.getValue().map.values();
AffectedRows affectedRows;
switch (onDuplicateKey) {
case IGNORE:
affectedRows = commandsExecutor.executeInsertsOnDuplicateKeyIgnore(table, commands);
break;
case UPDATE:
affectedRows = commandsExecutor.executeInsertsOnDuplicateKeyUpdate(table, commands);
break;
case FAIL:
default:
affectedRows = commandsExecutor.executeInserts(table, commands);
break;
}
stats.addAffectedRows(table.getName(), affectedRows);
}
for (Map.Entry> entry : updates.entrySet()) {
DataTable table = entry.getKey();
AffectedRows affectedRows = commandsExecutor.executeUpdates(table, entry.getValue().map.values());
stats.addAffectedRows(table.getName(), affectedRows);
}
for (Map.Entry> entry : insertsOnDuplicateUpdate.entrySet()) {
DataTable table = entry.getKey();
AffectedRows affectedRows = commandsExecutor.executeInsertsOnDuplicateKeyUpdate(table, entry.getValue().map.values());
stats.addAffectedRows(table.getName(), affectedRows);
}
}
private AbstractRecordCommand getOrCreate(Map> map, Supplier> creator, Supplier commandCreator, DataTable table, EntityChange entityChange) {
//noinspection unchecked
IdToCommandMap< RC> idToCommandMap = map.computeIfAbsent(table, k -> creator.get());
return idToCommandMap.getOrCreate(entityChange, commandCreator);
}
private static class IdToCommandMap {
private final Map map = new HashMap<>();
AbstractRecordCommand getOrCreate(EntityChange entityChange, Supplier commandCreator) {
return map.computeIfAbsent(entityChange, k -> commandCreator.get());
}
RC get(EntityChange entityChange) {
return map.get(entityChange);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy