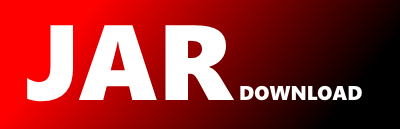
com.kenticocloud.delivery.RichTextElementConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of delivery-sdk-java Show documentation
Show all versions of delivery-sdk-java Show documentation
Kentico Cloud Delivery Java SDK https://kenticocloud.com/
/*
* MIT License
*
* Copyright (c) 2017
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package com.kenticocloud.delivery;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.JsonDeserializer;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.deser.ResolvableDeserializer;
import com.fasterxml.jackson.databind.deser.std.StdDeserializer;
import java.io.IOException;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class RichTextElementConverter extends StdDeserializer implements ResolvableDeserializer {
transient ContentLinkUrlResolver contentLinkUrlResolver;
transient BrokenLinkUrlResolver brokenLinkUrlResolver;
transient StronglyTypedContentItemConverter stronglyTypedContentItemConverter;
private final transient JsonDeserializer> defaultDeserializer;
/*
Regex prior to the Java \ escapes:
]+?data-item-id=\"(?[^\"]+)\"[^>]*>
Regex explanation:
]+?
+? Quantifier — Matches between one and unlimited times, as few times as possible, expanding as needed (lazy)
> matches the character > literally (case sensitive)
data-item-id= matches the characters data-item-id= literally (case sensitive)
\" matches the character " literally (case sensitive)
Named Capture Group id (?[^\"]+)
Match a single character not present in the list below [^\"]+
+ Quantifier — Matches between one and unlimited times, as many times as possible, giving back as needed (greedy)
\" matches the character " literally (case sensitive)
\" matches the character " literally (case sensitive)
Match a single character not present in the list below [^>]*
* Quantifier — Matches between zero and unlimited times, as many times as possible, giving back as needed (greedy)
> matches the character > literally (case sensitive)
> matches the character > literally (case sensitive)
Global pattern flags
g modifier: global. All matches (don't return after first match)
*/
private Pattern linkPattern = Pattern.compile("]+?data-item-id=\\\"(?[^\\\"]+)\\\"[^>]*>");
/*
Regex prior to the Java \ escapes:
© 2015 - 2024 Weber Informatics LLC | Privacy Policy