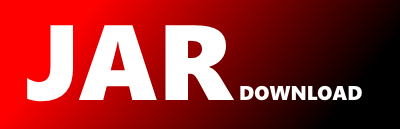
com.kibocommerce.sdk.fulfillment.api.ShipmentControllerApi Maven / Gradle / Ivy
The newest version!
/*
* Kibo Fulfillment API - Production Profile
* REST API backing the Kibo Fulfiller User Interface
*
* OpenAPI spec version: 1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.kibocommerce.sdk.fulfillment.api;
import com.kibocommerce.sdk.fulfillment.ApiCallback;
import com.kibocommerce.sdk.fulfillment.ApiClient;
import com.kibocommerce.sdk.fulfillment.ApiException;
import com.kibocommerce.sdk.fulfillment.ApiResponse;
import com.kibocommerce.sdk.fulfillment.Configuration;
import com.kibocommerce.sdk.fulfillment.Pair;
import com.kibocommerce.sdk.fulfillment.ProgressRequestBody;
import com.kibocommerce.sdk.fulfillment.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import com.kibocommerce.sdk.fulfillment.model.BackorderItemsRequest;
import com.kibocommerce.sdk.fulfillment.model.BackorderItemsUpdateRequest;
import com.kibocommerce.sdk.fulfillment.model.BackorderShipmentRequest;
import com.kibocommerce.sdk.fulfillment.model.CancelItemsRequest;
import com.kibocommerce.sdk.fulfillment.model.CancelShipment;
import com.kibocommerce.sdk.fulfillment.model.CollectionModelOfDashboardResponse;
import com.kibocommerce.sdk.fulfillment.model.CollectionModelOfEntityModelOfShipment;
import com.kibocommerce.sdk.fulfillment.model.CollectionModelOfLocationSummary;
import com.kibocommerce.sdk.fulfillment.model.CollectionModelOfShipment;
import com.kibocommerce.sdk.fulfillment.model.CollectionModelOfTask;
import com.kibocommerce.sdk.fulfillment.model.Destination;
import com.kibocommerce.sdk.fulfillment.model.EntityModelOfDashboardResponse;
import com.kibocommerce.sdk.fulfillment.model.EntityModelOfShipment;
import org.threeten.bp.OffsetDateTime;
import com.kibocommerce.sdk.fulfillment.model.PagedModelOfEntityModelOfShipment;
import com.kibocommerce.sdk.fulfillment.model.PickupItemsRequest;
import com.kibocommerce.sdk.fulfillment.model.ReassignItemsRequest;
import com.kibocommerce.sdk.fulfillment.model.ReassignShipment;
import com.kibocommerce.sdk.fulfillment.model.RejectItemsRequest;
import com.kibocommerce.sdk.fulfillment.model.RejectShipment;
import com.kibocommerce.sdk.fulfillment.model.Shipment;
import com.kibocommerce.sdk.fulfillment.model.TaskComplete;
import com.kibocommerce.sdk.fulfillment.model.TransferItemsRequest;
import com.kibocommerce.sdk.fulfillment.model.TransferShipment;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ShipmentControllerApi {
private ApiClient apiClient;
public ShipmentControllerApi() {
this(Configuration.getDefaultApiClient());
}
public ShipmentControllerApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for backorderItemsUpdateUsingPUT
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param backorderItemsUpdateRequest backorderItemsUpdateRequestDto (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call backorderItemsUpdateUsingPUTCall(Integer shipmentNumber, Integer xVolTenant, BackorderItemsUpdateRequest backorderItemsUpdateRequest, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = backorderItemsUpdateRequest;
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/backorderedItems"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call backorderItemsUpdateUsingPUTValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, BackorderItemsUpdateRequest backorderItemsUpdateRequest, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling backorderItemsUpdateUsingPUT(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling backorderItemsUpdateUsingPUT(Async)");
}
// verify the required parameter 'backorderItemsUpdateRequest' is set
if (backorderItemsUpdateRequest == null) {
throw new ApiException("Missing the required parameter 'backorderItemsUpdateRequest' when calling backorderItemsUpdateUsingPUT(Async)");
}
com.squareup.okhttp.Call call = backorderItemsUpdateUsingPUTCall(shipmentNumber, xVolTenant, backorderItemsUpdateRequest, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* backorderItemsUpdate
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param backorderItemsUpdateRequest backorderItemsUpdateRequestDto (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment backorderItemsUpdateUsingPUT(Integer shipmentNumber, Integer xVolTenant, BackorderItemsUpdateRequest backorderItemsUpdateRequest, Integer xVolSite) throws ApiException {
ApiResponse resp = backorderItemsUpdateUsingPUTWithHttpInfo(shipmentNumber, xVolTenant, backorderItemsUpdateRequest, xVolSite);
return resp.getData();
}
/**
* backorderItemsUpdate
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param backorderItemsUpdateRequest backorderItemsUpdateRequestDto (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse backorderItemsUpdateUsingPUTWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, BackorderItemsUpdateRequest backorderItemsUpdateRequest, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = backorderItemsUpdateUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, backorderItemsUpdateRequest, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* backorderItemsUpdate (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param backorderItemsUpdateRequest backorderItemsUpdateRequestDto (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call backorderItemsUpdateUsingPUTAsync(Integer shipmentNumber, Integer xVolTenant, BackorderItemsUpdateRequest backorderItemsUpdateRequest, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = backorderItemsUpdateUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, backorderItemsUpdateRequest, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for backorderItemsUsingPOST
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param backorderItemsRequest backorderItemsRequestDto (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call backorderItemsUsingPOSTCall(Integer shipmentNumber, Integer xVolTenant, BackorderItemsRequest backorderItemsRequest, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = backorderItemsRequest;
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/backorderedItems"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call backorderItemsUsingPOSTValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, BackorderItemsRequest backorderItemsRequest, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling backorderItemsUsingPOST(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling backorderItemsUsingPOST(Async)");
}
// verify the required parameter 'backorderItemsRequest' is set
if (backorderItemsRequest == null) {
throw new ApiException("Missing the required parameter 'backorderItemsRequest' when calling backorderItemsUsingPOST(Async)");
}
com.squareup.okhttp.Call call = backorderItemsUsingPOSTCall(shipmentNumber, xVolTenant, backorderItemsRequest, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* backorderItems
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param backorderItemsRequest backorderItemsRequestDto (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment backorderItemsUsingPOST(Integer shipmentNumber, Integer xVolTenant, BackorderItemsRequest backorderItemsRequest, Integer xVolSite) throws ApiException {
ApiResponse resp = backorderItemsUsingPOSTWithHttpInfo(shipmentNumber, xVolTenant, backorderItemsRequest, xVolSite);
return resp.getData();
}
/**
* backorderItems
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param backorderItemsRequest backorderItemsRequestDto (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse backorderItemsUsingPOSTWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, BackorderItemsRequest backorderItemsRequest, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = backorderItemsUsingPOSTValidateBeforeCall(shipmentNumber, xVolTenant, backorderItemsRequest, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* backorderItems (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param backorderItemsRequest backorderItemsRequestDto (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call backorderItemsUsingPOSTAsync(Integer shipmentNumber, Integer xVolTenant, BackorderItemsRequest backorderItemsRequest, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = backorderItemsUsingPOSTValidateBeforeCall(shipmentNumber, xVolTenant, backorderItemsRequest, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for backorderShipmentUsingPOST
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param backorderShipmentRequest backorderShipmentRequestDto (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call backorderShipmentUsingPOSTCall(Integer shipmentNumber, Integer xVolTenant, BackorderShipmentRequest backorderShipmentRequest, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = backorderShipmentRequest;
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/backordered"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call backorderShipmentUsingPOSTValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, BackorderShipmentRequest backorderShipmentRequest, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling backorderShipmentUsingPOST(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling backorderShipmentUsingPOST(Async)");
}
// verify the required parameter 'backorderShipmentRequest' is set
if (backorderShipmentRequest == null) {
throw new ApiException("Missing the required parameter 'backorderShipmentRequest' when calling backorderShipmentUsingPOST(Async)");
}
com.squareup.okhttp.Call call = backorderShipmentUsingPOSTCall(shipmentNumber, xVolTenant, backorderShipmentRequest, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* backorderShipment
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param backorderShipmentRequest backorderShipmentRequestDto (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment backorderShipmentUsingPOST(Integer shipmentNumber, Integer xVolTenant, BackorderShipmentRequest backorderShipmentRequest, Integer xVolSite) throws ApiException {
ApiResponse resp = backorderShipmentUsingPOSTWithHttpInfo(shipmentNumber, xVolTenant, backorderShipmentRequest, xVolSite);
return resp.getData();
}
/**
* backorderShipment
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param backorderShipmentRequest backorderShipmentRequestDto (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse backorderShipmentUsingPOSTWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, BackorderShipmentRequest backorderShipmentRequest, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = backorderShipmentUsingPOSTValidateBeforeCall(shipmentNumber, xVolTenant, backorderShipmentRequest, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* backorderShipment (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param backorderShipmentRequest backorderShipmentRequestDto (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call backorderShipmentUsingPOSTAsync(Integer shipmentNumber, Integer xVolTenant, BackorderShipmentRequest backorderShipmentRequest, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = backorderShipmentUsingPOSTValidateBeforeCall(shipmentNumber, xVolTenant, backorderShipmentRequest, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for cancelItemsUsingPUT
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param cancelItemsRequest cancelItemsRequestDto (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call cancelItemsUsingPUTCall(Integer shipmentNumber, Integer xVolTenant, CancelItemsRequest cancelItemsRequest, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = cancelItemsRequest;
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/canceledItems"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call cancelItemsUsingPUTValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, CancelItemsRequest cancelItemsRequest, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling cancelItemsUsingPUT(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling cancelItemsUsingPUT(Async)");
}
// verify the required parameter 'cancelItemsRequest' is set
if (cancelItemsRequest == null) {
throw new ApiException("Missing the required parameter 'cancelItemsRequest' when calling cancelItemsUsingPUT(Async)");
}
com.squareup.okhttp.Call call = cancelItemsUsingPUTCall(shipmentNumber, xVolTenant, cancelItemsRequest, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* cancelItems
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param cancelItemsRequest cancelItemsRequestDto (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment cancelItemsUsingPUT(Integer shipmentNumber, Integer xVolTenant, CancelItemsRequest cancelItemsRequest, Integer xVolSite) throws ApiException {
ApiResponse resp = cancelItemsUsingPUTWithHttpInfo(shipmentNumber, xVolTenant, cancelItemsRequest, xVolSite);
return resp.getData();
}
/**
* cancelItems
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param cancelItemsRequest cancelItemsRequestDto (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse cancelItemsUsingPUTWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, CancelItemsRequest cancelItemsRequest, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = cancelItemsUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, cancelItemsRequest, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* cancelItems (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param cancelItemsRequest cancelItemsRequestDto (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call cancelItemsUsingPUTAsync(Integer shipmentNumber, Integer xVolTenant, CancelItemsRequest cancelItemsRequest, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = cancelItemsUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, cancelItemsRequest, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for cancelShipmentUsingPUT
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param cancelShipment cancelShipmentRequestDto (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call cancelShipmentUsingPUTCall(Integer shipmentNumber, Integer xVolTenant, CancelShipment cancelShipment, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = cancelShipment;
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/canceled"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call cancelShipmentUsingPUTValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, CancelShipment cancelShipment, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling cancelShipmentUsingPUT(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling cancelShipmentUsingPUT(Async)");
}
// verify the required parameter 'cancelShipment' is set
if (cancelShipment == null) {
throw new ApiException("Missing the required parameter 'cancelShipment' when calling cancelShipmentUsingPUT(Async)");
}
com.squareup.okhttp.Call call = cancelShipmentUsingPUTCall(shipmentNumber, xVolTenant, cancelShipment, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* cancelShipment
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param cancelShipment cancelShipmentRequestDto (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment cancelShipmentUsingPUT(Integer shipmentNumber, Integer xVolTenant, CancelShipment cancelShipment, Integer xVolSite) throws ApiException {
ApiResponse resp = cancelShipmentUsingPUTWithHttpInfo(shipmentNumber, xVolTenant, cancelShipment, xVolSite);
return resp.getData();
}
/**
* cancelShipment
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param cancelShipment cancelShipmentRequestDto (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse cancelShipmentUsingPUTWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, CancelShipment cancelShipment, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = cancelShipmentUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, cancelShipment, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* cancelShipment (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param cancelShipment cancelShipmentRequestDto (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call cancelShipmentUsingPUTAsync(Integer shipmentNumber, Integer xVolTenant, CancelShipment cancelShipment, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = cancelShipmentUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, cancelShipment, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for cancelShipmentsUsingPUT
* @param orderId orderId (required)
* @param xVolTenant (required)
* @param cancelShipment cancelShipmentRequestDto (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call cancelShipmentsUsingPUTCall(String orderId, Integer xVolTenant, CancelShipment cancelShipment, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = cancelShipment;
// create path and map variables
String localVarPath = "/commerce/shipments/order/{orderId}/canceled"
.replaceAll("\\{" + "orderId" + "\\}", apiClient.escapeString(orderId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call cancelShipmentsUsingPUTValidateBeforeCall(String orderId, Integer xVolTenant, CancelShipment cancelShipment, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orderId' is set
if (orderId == null) {
throw new ApiException("Missing the required parameter 'orderId' when calling cancelShipmentsUsingPUT(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling cancelShipmentsUsingPUT(Async)");
}
// verify the required parameter 'cancelShipment' is set
if (cancelShipment == null) {
throw new ApiException("Missing the required parameter 'cancelShipment' when calling cancelShipmentsUsingPUT(Async)");
}
com.squareup.okhttp.Call call = cancelShipmentsUsingPUTCall(orderId, xVolTenant, cancelShipment, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* cancelShipments
*
* @param orderId orderId (required)
* @param xVolTenant (required)
* @param cancelShipment cancelShipmentRequestDto (required)
* @param xVolSite (optional)
* @return CollectionModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CollectionModelOfShipment cancelShipmentsUsingPUT(String orderId, Integer xVolTenant, CancelShipment cancelShipment, Integer xVolSite) throws ApiException {
ApiResponse resp = cancelShipmentsUsingPUTWithHttpInfo(orderId, xVolTenant, cancelShipment, xVolSite);
return resp.getData();
}
/**
* cancelShipments
*
* @param orderId orderId (required)
* @param xVolTenant (required)
* @param cancelShipment cancelShipmentRequestDto (required)
* @param xVolSite (optional)
* @return ApiResponse<CollectionModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse cancelShipmentsUsingPUTWithHttpInfo(String orderId, Integer xVolTenant, CancelShipment cancelShipment, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = cancelShipmentsUsingPUTValidateBeforeCall(orderId, xVolTenant, cancelShipment, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* cancelShipments (asynchronously)
*
* @param orderId orderId (required)
* @param xVolTenant (required)
* @param cancelShipment cancelShipmentRequestDto (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call cancelShipmentsUsingPUTAsync(String orderId, Integer xVolTenant, CancelShipment cancelShipment, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = cancelShipmentsUsingPUTValidateBeforeCall(orderId, xVolTenant, cancelShipment, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for customerAtCurbsideUsingPUT
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param requestBody pickupInfo (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call customerAtCurbsideUsingPUTCall(Integer shipmentNumber, Integer xVolTenant, Map requestBody, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = requestBody;
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/customerAtCurbside"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call customerAtCurbsideUsingPUTValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, Map requestBody, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling customerAtCurbsideUsingPUT(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling customerAtCurbsideUsingPUT(Async)");
}
// verify the required parameter 'requestBody' is set
if (requestBody == null) {
throw new ApiException("Missing the required parameter 'requestBody' when calling customerAtCurbsideUsingPUT(Async)");
}
com.squareup.okhttp.Call call = customerAtCurbsideUsingPUTCall(shipmentNumber, xVolTenant, requestBody, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* customerAtCurbside
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param requestBody pickupInfo (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment customerAtCurbsideUsingPUT(Integer shipmentNumber, Integer xVolTenant, Map requestBody, Integer xVolSite) throws ApiException {
ApiResponse resp = customerAtCurbsideUsingPUTWithHttpInfo(shipmentNumber, xVolTenant, requestBody, xVolSite);
return resp.getData();
}
/**
* customerAtCurbside
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param requestBody pickupInfo (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse customerAtCurbsideUsingPUTWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, Map requestBody, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = customerAtCurbsideUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, requestBody, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* customerAtCurbside (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param requestBody pickupInfo (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call customerAtCurbsideUsingPUTAsync(Integer shipmentNumber, Integer xVolTenant, Map requestBody, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = customerAtCurbsideUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, requestBody, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for customerAtStoreUsingPUT
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call customerAtStoreUsingPUTCall(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = new Object();
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/customerAtStore"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call customerAtStoreUsingPUTValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling customerAtStoreUsingPUT(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling customerAtStoreUsingPUT(Async)");
}
com.squareup.okhttp.Call call = customerAtStoreUsingPUTCall(shipmentNumber, xVolTenant, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* customerAtStore
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment customerAtStoreUsingPUT(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite) throws ApiException {
ApiResponse resp = customerAtStoreUsingPUTWithHttpInfo(shipmentNumber, xVolTenant, xVolSite);
return resp.getData();
}
/**
* customerAtStore
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse customerAtStoreUsingPUTWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = customerAtStoreUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* customerAtStore (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call customerAtStoreUsingPUTAsync(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = customerAtStoreUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for customerCareItemsUsingPOST
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param rejectItemsRequest rejectItemsRequestDto (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call customerCareItemsUsingPOSTCall(Integer shipmentNumber, Integer xVolTenant, RejectItemsRequest rejectItemsRequest, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = rejectItemsRequest;
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/customerCaredItems"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call customerCareItemsUsingPOSTValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, RejectItemsRequest rejectItemsRequest, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling customerCareItemsUsingPOST(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling customerCareItemsUsingPOST(Async)");
}
// verify the required parameter 'rejectItemsRequest' is set
if (rejectItemsRequest == null) {
throw new ApiException("Missing the required parameter 'rejectItemsRequest' when calling customerCareItemsUsingPOST(Async)");
}
com.squareup.okhttp.Call call = customerCareItemsUsingPOSTCall(shipmentNumber, xVolTenant, rejectItemsRequest, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* customerCareItems
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param rejectItemsRequest rejectItemsRequestDto (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment customerCareItemsUsingPOST(Integer shipmentNumber, Integer xVolTenant, RejectItemsRequest rejectItemsRequest, Integer xVolSite) throws ApiException {
ApiResponse resp = customerCareItemsUsingPOSTWithHttpInfo(shipmentNumber, xVolTenant, rejectItemsRequest, xVolSite);
return resp.getData();
}
/**
* customerCareItems
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param rejectItemsRequest rejectItemsRequestDto (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse customerCareItemsUsingPOSTWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, RejectItemsRequest rejectItemsRequest, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = customerCareItemsUsingPOSTValidateBeforeCall(shipmentNumber, xVolTenant, rejectItemsRequest, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* customerCareItems (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param rejectItemsRequest rejectItemsRequestDto (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call customerCareItemsUsingPOSTAsync(Integer shipmentNumber, Integer xVolTenant, RejectItemsRequest rejectItemsRequest, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = customerCareItemsUsingPOSTValidateBeforeCall(shipmentNumber, xVolTenant, rejectItemsRequest, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for customerCareShipmentUsingPUT
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param rejectShipment rejectShipmentRequestDto (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call customerCareShipmentUsingPUTCall(Integer shipmentNumber, Integer xVolTenant, RejectShipment rejectShipment, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = rejectShipment;
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/customerCared"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call customerCareShipmentUsingPUTValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, RejectShipment rejectShipment, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling customerCareShipmentUsingPUT(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling customerCareShipmentUsingPUT(Async)");
}
// verify the required parameter 'rejectShipment' is set
if (rejectShipment == null) {
throw new ApiException("Missing the required parameter 'rejectShipment' when calling customerCareShipmentUsingPUT(Async)");
}
com.squareup.okhttp.Call call = customerCareShipmentUsingPUTCall(shipmentNumber, xVolTenant, rejectShipment, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* customerCareShipment
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param rejectShipment rejectShipmentRequestDto (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment customerCareShipmentUsingPUT(Integer shipmentNumber, Integer xVolTenant, RejectShipment rejectShipment, Integer xVolSite) throws ApiException {
ApiResponse resp = customerCareShipmentUsingPUTWithHttpInfo(shipmentNumber, xVolTenant, rejectShipment, xVolSite);
return resp.getData();
}
/**
* customerCareShipment
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param rejectShipment rejectShipmentRequestDto (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse customerCareShipmentUsingPUTWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, RejectShipment rejectShipment, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = customerCareShipmentUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, rejectShipment, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* customerCareShipment (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param rejectShipment rejectShipmentRequestDto (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call customerCareShipmentUsingPUTAsync(Integer shipmentNumber, Integer xVolTenant, RejectShipment rejectShipment, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = customerCareShipmentUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, rejectShipment, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for customerInTransitUsingPUT
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call customerInTransitUsingPUTCall(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = new Object();
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/customerInTransit"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call customerInTransitUsingPUTValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling customerInTransitUsingPUT(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling customerInTransitUsingPUT(Async)");
}
com.squareup.okhttp.Call call = customerInTransitUsingPUTCall(shipmentNumber, xVolTenant, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* customerInTransit
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment customerInTransitUsingPUT(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite) throws ApiException {
ApiResponse resp = customerInTransitUsingPUTWithHttpInfo(shipmentNumber, xVolTenant, xVolSite);
return resp.getData();
}
/**
* customerInTransit
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse customerInTransitUsingPUTWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = customerInTransitUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* customerInTransit (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call customerInTransitUsingPUTAsync(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = customerInTransitUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for deleteShipmentUsingDELETE
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call deleteShipmentUsingDELETECall(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = new Object();
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call deleteShipmentUsingDELETEValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling deleteShipmentUsingDELETE(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling deleteShipmentUsingDELETE(Async)");
}
com.squareup.okhttp.Call call = deleteShipmentUsingDELETECall(shipmentNumber, xVolTenant, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* deleteShipment
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteShipmentUsingDELETE(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite) throws ApiException {
deleteShipmentUsingDELETEWithHttpInfo(shipmentNumber, xVolTenant, xVolSite);
}
/**
* deleteShipment
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteShipmentUsingDELETEWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = deleteShipmentUsingDELETEValidateBeforeCall(shipmentNumber, xVolTenant, xVolSite, null, null);
return apiClient.execute(call);
}
/**
* deleteShipment (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call deleteShipmentUsingDELETEAsync(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = deleteShipmentUsingDELETEValidateBeforeCall(shipmentNumber, xVolTenant, xVolSite, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for destinationUpdateUsingPUT
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param destination destinationDto (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call destinationUpdateUsingPUTCall(Integer shipmentNumber, Integer xVolTenant, Destination destination, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = destination;
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/destination"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call destinationUpdateUsingPUTValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, Destination destination, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling destinationUpdateUsingPUT(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling destinationUpdateUsingPUT(Async)");
}
// verify the required parameter 'destination' is set
if (destination == null) {
throw new ApiException("Missing the required parameter 'destination' when calling destinationUpdateUsingPUT(Async)");
}
com.squareup.okhttp.Call call = destinationUpdateUsingPUTCall(shipmentNumber, xVolTenant, destination, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* destinationUpdate
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param destination destinationDto (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment destinationUpdateUsingPUT(Integer shipmentNumber, Integer xVolTenant, Destination destination, Integer xVolSite) throws ApiException {
ApiResponse resp = destinationUpdateUsingPUTWithHttpInfo(shipmentNumber, xVolTenant, destination, xVolSite);
return resp.getData();
}
/**
* destinationUpdate
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param destination destinationDto (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse destinationUpdateUsingPUTWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, Destination destination, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = destinationUpdateUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, destination, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* destinationUpdate (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param destination destinationDto (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call destinationUpdateUsingPUTAsync(Integer shipmentNumber, Integer xVolTenant, Destination destination, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = destinationUpdateUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, destination, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for executeUsingPUT
* @param shipmentNumber shipmentNumber (required)
* @param taskName taskName (required)
* @param xVolTenant (required)
* @param taskComplete taskCompleteDto (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call executeUsingPUTCall(Integer shipmentNumber, String taskName, Integer xVolTenant, TaskComplete taskComplete, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = taskComplete;
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/tasks/{taskName}/completed"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()))
.replaceAll("\\{" + "taskName" + "\\}", apiClient.escapeString(taskName.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call executeUsingPUTValidateBeforeCall(Integer shipmentNumber, String taskName, Integer xVolTenant, TaskComplete taskComplete, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling executeUsingPUT(Async)");
}
// verify the required parameter 'taskName' is set
if (taskName == null) {
throw new ApiException("Missing the required parameter 'taskName' when calling executeUsingPUT(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling executeUsingPUT(Async)");
}
// verify the required parameter 'taskComplete' is set
if (taskComplete == null) {
throw new ApiException("Missing the required parameter 'taskComplete' when calling executeUsingPUT(Async)");
}
com.squareup.okhttp.Call call = executeUsingPUTCall(shipmentNumber, taskName, xVolTenant, taskComplete, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* execute
*
* @param shipmentNumber shipmentNumber (required)
* @param taskName taskName (required)
* @param xVolTenant (required)
* @param taskComplete taskCompleteDto (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment executeUsingPUT(Integer shipmentNumber, String taskName, Integer xVolTenant, TaskComplete taskComplete, Integer xVolSite) throws ApiException {
ApiResponse resp = executeUsingPUTWithHttpInfo(shipmentNumber, taskName, xVolTenant, taskComplete, xVolSite);
return resp.getData();
}
/**
* execute
*
* @param shipmentNumber shipmentNumber (required)
* @param taskName taskName (required)
* @param xVolTenant (required)
* @param taskComplete taskCompleteDto (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse executeUsingPUTWithHttpInfo(Integer shipmentNumber, String taskName, Integer xVolTenant, TaskComplete taskComplete, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = executeUsingPUTValidateBeforeCall(shipmentNumber, taskName, xVolTenant, taskComplete, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* execute (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param taskName taskName (required)
* @param xVolTenant (required)
* @param taskComplete taskCompleteDto (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call executeUsingPUTAsync(Integer shipmentNumber, String taskName, Integer xVolTenant, TaskComplete taskComplete, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = executeUsingPUTValidateBeforeCall(shipmentNumber, taskName, xVolTenant, taskComplete, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for fulfillShipmentUsingPUT
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call fulfillShipmentUsingPUTCall(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = new Object();
// create path and map variables
String localVarPath = "/commerce/shipments/{shipmentNumber}/fulfilled"
.replaceAll("\\{" + "shipmentNumber" + "\\}", apiClient.escapeString(shipmentNumber.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call fulfillShipmentUsingPUTValidateBeforeCall(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentNumber' is set
if (shipmentNumber == null) {
throw new ApiException("Missing the required parameter 'shipmentNumber' when calling fulfillShipmentUsingPUT(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling fulfillShipmentUsingPUT(Async)");
}
com.squareup.okhttp.Call call = fulfillShipmentUsingPUTCall(shipmentNumber, xVolTenant, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* fulfillShipment
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @return EntityModelOfShipment
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfShipment fulfillShipmentUsingPUT(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite) throws ApiException {
ApiResponse resp = fulfillShipmentUsingPUTWithHttpInfo(shipmentNumber, xVolTenant, xVolSite);
return resp.getData();
}
/**
* fulfillShipment
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfShipment>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse fulfillShipmentUsingPUTWithHttpInfo(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = fulfillShipmentUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* fulfillShipment (asynchronously)
*
* @param shipmentNumber shipmentNumber (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call fulfillShipmentUsingPUTAsync(Integer shipmentNumber, Integer xVolTenant, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = fulfillShipmentUsingPUTValidateBeforeCall(shipmentNumber, xVolTenant, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getDashboardUsingGET
* @param xVolTenant (required)
* @param fulfillmentLocationCodes fulfillmentLocationCodes (optional)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getDashboardUsingGETCall(Integer xVolTenant, List fulfillmentLocationCodes, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = new Object();
// create path and map variables
String localVarPath = "/commerce/shipments/dashboard";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (fulfillmentLocationCodes != null) {
localVarCollectionQueryParams.addAll(apiClient.parameterToPairs("multi", "fulfillmentLocationCodes", fulfillmentLocationCodes));
}
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getDashboardUsingGETValidateBeforeCall(Integer xVolTenant, List fulfillmentLocationCodes, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling getDashboardUsingGET(Async)");
}
com.squareup.okhttp.Call call = getDashboardUsingGETCall(xVolTenant, fulfillmentLocationCodes, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* getDashboard
*
* @param xVolTenant (required)
* @param fulfillmentLocationCodes fulfillmentLocationCodes (optional)
* @param xVolSite (optional)
* @return CollectionModelOfDashboardResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CollectionModelOfDashboardResponse getDashboardUsingGET(Integer xVolTenant, List fulfillmentLocationCodes, Integer xVolSite) throws ApiException {
ApiResponse resp = getDashboardUsingGETWithHttpInfo(xVolTenant, fulfillmentLocationCodes, xVolSite);
return resp.getData();
}
/**
* getDashboard
*
* @param xVolTenant (required)
* @param fulfillmentLocationCodes fulfillmentLocationCodes (optional)
* @param xVolSite (optional)
* @return ApiResponse<CollectionModelOfDashboardResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getDashboardUsingGETWithHttpInfo(Integer xVolTenant, List fulfillmentLocationCodes, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = getDashboardUsingGETValidateBeforeCall(xVolTenant, fulfillmentLocationCodes, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* getDashboard (asynchronously)
*
* @param xVolTenant (required)
* @param fulfillmentLocationCodes fulfillmentLocationCodes (optional)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getDashboardUsingGETAsync(Integer xVolTenant, List fulfillmentLocationCodes, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getDashboardUsingGETValidateBeforeCall(xVolTenant, fulfillmentLocationCodes, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getLocationSummaryReportUsingGET
* @param locationCodes locationCodes (required)
* @param startDateTime startDateTime (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getLocationSummaryReportUsingGETCall(List locationCodes, OffsetDateTime startDateTime, Integer xVolTenant, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = new Object();
// create path and map variables
String localVarPath = "/commerce/shipments/locationSummaryReport";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (locationCodes != null) {
localVarCollectionQueryParams.addAll(apiClient.parameterToPairs("multi", "locationCodes", locationCodes));
}
if (startDateTime != null) {
localVarQueryParams.addAll(apiClient.parameterToPair("startDateTime", startDateTime));
}
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getLocationSummaryReportUsingGETValidateBeforeCall(List locationCodes, OffsetDateTime startDateTime, Integer xVolTenant, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'locationCodes' is set
if (locationCodes == null) {
throw new ApiException("Missing the required parameter 'locationCodes' when calling getLocationSummaryReportUsingGET(Async)");
}
// verify the required parameter 'startDateTime' is set
if (startDateTime == null) {
throw new ApiException("Missing the required parameter 'startDateTime' when calling getLocationSummaryReportUsingGET(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling getLocationSummaryReportUsingGET(Async)");
}
com.squareup.okhttp.Call call = getLocationSummaryReportUsingGETCall(locationCodes, startDateTime, xVolTenant, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* getLocationSummaryReport
*
* @param locationCodes locationCodes (required)
* @param startDateTime startDateTime (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @return CollectionModelOfLocationSummary
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public CollectionModelOfLocationSummary getLocationSummaryReportUsingGET(List locationCodes, OffsetDateTime startDateTime, Integer xVolTenant, Integer xVolSite) throws ApiException {
ApiResponse resp = getLocationSummaryReportUsingGETWithHttpInfo(locationCodes, startDateTime, xVolTenant, xVolSite);
return resp.getData();
}
/**
* getLocationSummaryReport
*
* @param locationCodes locationCodes (required)
* @param startDateTime startDateTime (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @return ApiResponse<CollectionModelOfLocationSummary>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getLocationSummaryReportUsingGETWithHttpInfo(List locationCodes, OffsetDateTime startDateTime, Integer xVolTenant, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = getLocationSummaryReportUsingGETValidateBeforeCall(locationCodes, startDateTime, xVolTenant, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* getLocationSummaryReport (asynchronously)
*
* @param locationCodes locationCodes (required)
* @param startDateTime startDateTime (required)
* @param xVolTenant (required)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getLocationSummaryReportUsingGETAsync(List locationCodes, OffsetDateTime startDateTime, Integer xVolTenant, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getLocationSummaryReportUsingGETValidateBeforeCall(locationCodes, startDateTime, xVolTenant, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getShipmentStepCountByShipmentTypeUsingGET
* @param shipmentType shipmentType (required)
* @param xVolTenant (required)
* @param assignedLocations assignedLocations (optional)
* @param xVolSite (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getShipmentStepCountByShipmentTypeUsingGETCall(String shipmentType, Integer xVolTenant, List assignedLocations, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = new Object();
// create path and map variables
String localVarPath = "/commerce/shipments/countsByStep";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (assignedLocations != null) {
localVarCollectionQueryParams.addAll(apiClient.parameterToPairs("multi", "assignedLocations", assignedLocations));
}
if (shipmentType != null) {
localVarQueryParams.addAll(apiClient.parameterToPair("shipmentType", shipmentType));
}
Map localVarHeaderParams = new HashMap();
if (xVolSite != null) {
localVarHeaderParams.put("x-vol-site", apiClient.parameterToString(xVolSite));
}
if (xVolTenant != null) {
localVarHeaderParams.put("x-vol-tenant", apiClient.parameterToString(xVolTenant));
}
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/xml", "application/json", "application/hal+json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if (progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getShipmentStepCountByShipmentTypeUsingGETValidateBeforeCall(String shipmentType, Integer xVolTenant, List assignedLocations, Integer xVolSite, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'shipmentType' is set
if (shipmentType == null) {
throw new ApiException("Missing the required parameter 'shipmentType' when calling getShipmentStepCountByShipmentTypeUsingGET(Async)");
}
// verify the required parameter 'xVolTenant' is set
if (xVolTenant == null) {
throw new ApiException("Missing the required parameter 'xVolTenant' when calling getShipmentStepCountByShipmentTypeUsingGET(Async)");
}
com.squareup.okhttp.Call call = getShipmentStepCountByShipmentTypeUsingGETCall(shipmentType, xVolTenant, assignedLocations, xVolSite, progressListener, progressRequestListener);
return call;
}
/**
* getShipmentStepCountByShipmentType
*
* @param shipmentType shipmentType (required)
* @param xVolTenant (required)
* @param assignedLocations assignedLocations (optional)
* @param xVolSite (optional)
* @return EntityModelOfDashboardResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public EntityModelOfDashboardResponse getShipmentStepCountByShipmentTypeUsingGET(String shipmentType, Integer xVolTenant, List assignedLocations, Integer xVolSite) throws ApiException {
ApiResponse resp = getShipmentStepCountByShipmentTypeUsingGETWithHttpInfo(shipmentType, xVolTenant, assignedLocations, xVolSite);
return resp.getData();
}
/**
* getShipmentStepCountByShipmentType
*
* @param shipmentType shipmentType (required)
* @param xVolTenant (required)
* @param assignedLocations assignedLocations (optional)
* @param xVolSite (optional)
* @return ApiResponse<EntityModelOfDashboardResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getShipmentStepCountByShipmentTypeUsingGETWithHttpInfo(String shipmentType, Integer xVolTenant, List assignedLocations, Integer xVolSite) throws ApiException {
com.squareup.okhttp.Call call = getShipmentStepCountByShipmentTypeUsingGETValidateBeforeCall(shipmentType, xVolTenant, assignedLocations, xVolSite, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* getShipmentStepCountByShipmentType (asynchronously)
*
* @param shipmentType shipmentType (required)
* @param xVolTenant (required)
* @param assignedLocations assignedLocations (optional)
* @param xVolSite (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getShipmentStepCountByShipmentTypeUsingGETAsync(String shipmentType, Integer xVolTenant, List assignedLocations, Integer xVolSite, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getShipmentStepCountByShipmentTypeUsingGETValidateBeforeCall(shipmentType, xVolTenant, assignedLocations, xVolSite, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken