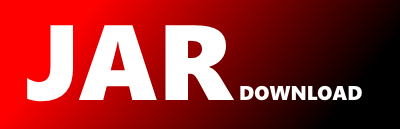
com.kibocommerce.sdk.fulfillment.model.ChangeMessage Maven / Gradle / Ivy
The newest version!
/*
* Kibo Fulfillment API - Production Profile
* REST API backing the Kibo Fulfiller User Interface
*
* OpenAPI spec version: 1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.kibocommerce.sdk.fulfillment.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.threeten.bp.OffsetDateTime;
/**
* ChangeMessage
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-12-08T12:42:53.880-06:00[America/Chicago]")
public class ChangeMessage {
public static final String SERIALIZED_NAME_AMOUNT = "amount";
@SerializedName(SERIALIZED_NAME_AMOUNT)
private BigDecimal amount;
public static final String SERIALIZED_NAME_APP_ID = "appId";
@SerializedName(SERIALIZED_NAME_APP_ID)
private String appId;
public static final String SERIALIZED_NAME_APP_KEY = "appKey";
@SerializedName(SERIALIZED_NAME_APP_KEY)
private String appKey;
public static final String SERIALIZED_NAME_APP_NAME = "appName";
@SerializedName(SERIALIZED_NAME_APP_NAME)
private String appName;
public static final String SERIALIZED_NAME_ATTRIBUTES = "attributes";
@SerializedName(SERIALIZED_NAME_ATTRIBUTES)
private Map attributes = null;
public static final String SERIALIZED_NAME_CHANGE_MESSAGE_ID = "changeMessageId";
@SerializedName(SERIALIZED_NAME_CHANGE_MESSAGE_ID)
private String changeMessageId;
public static final String SERIALIZED_NAME_CORRELATION_ID = "correlationId";
@SerializedName(SERIALIZED_NAME_CORRELATION_ID)
private String correlationId;
public static final String SERIALIZED_NAME_CREATED_DATE = "createdDate";
@SerializedName(SERIALIZED_NAME_CREATED_DATE)
private OffsetDateTime createdDate;
public static final String SERIALIZED_NAME_IDENTIFIER = "identifier";
@SerializedName(SERIALIZED_NAME_IDENTIFIER)
private String identifier;
public static final String SERIALIZED_NAME_MESSAGE = "message";
@SerializedName(SERIALIZED_NAME_MESSAGE)
private String message;
public static final String SERIALIZED_NAME_METADATA = "metadata";
@SerializedName(SERIALIZED_NAME_METADATA)
private String metadata;
public static final String SERIALIZED_NAME_NEW_VALUE = "newValue";
@SerializedName(SERIALIZED_NAME_NEW_VALUE)
private String newValue;
public static final String SERIALIZED_NAME_OLD_VALUE = "oldValue";
@SerializedName(SERIALIZED_NAME_OLD_VALUE)
private String oldValue;
public static final String SERIALIZED_NAME_SUBJECT = "subject";
@SerializedName(SERIALIZED_NAME_SUBJECT)
private String subject;
public static final String SERIALIZED_NAME_SUBJECT_TYPE = "subjectType";
@SerializedName(SERIALIZED_NAME_SUBJECT_TYPE)
private String subjectType;
public static final String SERIALIZED_NAME_SUCCESS = "success";
@SerializedName(SERIALIZED_NAME_SUCCESS)
private Boolean success;
public static final String SERIALIZED_NAME_USER_DISPLAY_NAME = "userDisplayName";
@SerializedName(SERIALIZED_NAME_USER_DISPLAY_NAME)
private String userDisplayName;
public static final String SERIALIZED_NAME_USER_FIRST_NAME = "userFirstName";
@SerializedName(SERIALIZED_NAME_USER_FIRST_NAME)
private String userFirstName;
public static final String SERIALIZED_NAME_USER_ID = "userId";
@SerializedName(SERIALIZED_NAME_USER_ID)
private String userId;
public static final String SERIALIZED_NAME_USER_LAST_NAME = "userLastName";
@SerializedName(SERIALIZED_NAME_USER_LAST_NAME)
private String userLastName;
public static final String SERIALIZED_NAME_VERB = "verb";
@SerializedName(SERIALIZED_NAME_VERB)
private String verb;
public ChangeMessage amount(BigDecimal amount) {
this.amount = amount;
return this;
}
/**
* Get amount
* @return amount
**/
@ApiModelProperty(value = "")
public BigDecimal getAmount() {
return amount;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public ChangeMessage appId(String appId) {
this.appId = appId;
return this;
}
/**
* Get appId
* @return appId
**/
@ApiModelProperty(value = "")
public String getAppId() {
return appId;
}
public void setAppId(String appId) {
this.appId = appId;
}
public ChangeMessage appKey(String appKey) {
this.appKey = appKey;
return this;
}
/**
* Get appKey
* @return appKey
**/
@ApiModelProperty(value = "")
public String getAppKey() {
return appKey;
}
public void setAppKey(String appKey) {
this.appKey = appKey;
}
public ChangeMessage appName(String appName) {
this.appName = appName;
return this;
}
/**
* Get appName
* @return appName
**/
@ApiModelProperty(value = "")
public String getAppName() {
return appName;
}
public void setAppName(String appName) {
this.appName = appName;
}
public ChangeMessage attributes(Map attributes) {
this.attributes = attributes;
return this;
}
public ChangeMessage putAttributesItem(String key, Object attributesItem) {
if (this.attributes == null) {
this.attributes = new HashMap();
}
this.attributes.put(key, attributesItem);
return this;
}
/**
* Get attributes
* @return attributes
**/
@ApiModelProperty(value = "")
public Map getAttributes() {
return attributes;
}
public void setAttributes(Map attributes) {
this.attributes = attributes;
}
public ChangeMessage changeMessageId(String changeMessageId) {
this.changeMessageId = changeMessageId;
return this;
}
/**
* Get changeMessageId
* @return changeMessageId
**/
@ApiModelProperty(value = "")
public String getChangeMessageId() {
return changeMessageId;
}
public void setChangeMessageId(String changeMessageId) {
this.changeMessageId = changeMessageId;
}
public ChangeMessage correlationId(String correlationId) {
this.correlationId = correlationId;
return this;
}
/**
* Get correlationId
* @return correlationId
**/
@ApiModelProperty(value = "")
public String getCorrelationId() {
return correlationId;
}
public void setCorrelationId(String correlationId) {
this.correlationId = correlationId;
}
public ChangeMessage createdDate(OffsetDateTime createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Get createdDate
* @return createdDate
**/
@ApiModelProperty(value = "")
public OffsetDateTime getCreatedDate() {
return createdDate;
}
public void setCreatedDate(OffsetDateTime createdDate) {
this.createdDate = createdDate;
}
public ChangeMessage identifier(String identifier) {
this.identifier = identifier;
return this;
}
/**
* Get identifier
* @return identifier
**/
@ApiModelProperty(value = "")
public String getIdentifier() {
return identifier;
}
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
public ChangeMessage message(String message) {
this.message = message;
return this;
}
/**
* Get message
* @return message
**/
@ApiModelProperty(value = "")
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public ChangeMessage metadata(String metadata) {
this.metadata = metadata;
return this;
}
/**
* Get metadata
* @return metadata
**/
@ApiModelProperty(value = "")
public String getMetadata() {
return metadata;
}
public void setMetadata(String metadata) {
this.metadata = metadata;
}
public ChangeMessage newValue(String newValue) {
this.newValue = newValue;
return this;
}
/**
* Get newValue
* @return newValue
**/
@ApiModelProperty(value = "")
public String getNewValue() {
return newValue;
}
public void setNewValue(String newValue) {
this.newValue = newValue;
}
public ChangeMessage oldValue(String oldValue) {
this.oldValue = oldValue;
return this;
}
/**
* Get oldValue
* @return oldValue
**/
@ApiModelProperty(value = "")
public String getOldValue() {
return oldValue;
}
public void setOldValue(String oldValue) {
this.oldValue = oldValue;
}
public ChangeMessage subject(String subject) {
this.subject = subject;
return this;
}
/**
* Get subject
* @return subject
**/
@ApiModelProperty(value = "")
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public ChangeMessage subjectType(String subjectType) {
this.subjectType = subjectType;
return this;
}
/**
* Get subjectType
* @return subjectType
**/
@ApiModelProperty(value = "")
public String getSubjectType() {
return subjectType;
}
public void setSubjectType(String subjectType) {
this.subjectType = subjectType;
}
public ChangeMessage success(Boolean success) {
this.success = success;
return this;
}
/**
* Get success
* @return success
**/
@ApiModelProperty(value = "")
public Boolean getSuccess() {
return success;
}
public void setSuccess(Boolean success) {
this.success = success;
}
public ChangeMessage userDisplayName(String userDisplayName) {
this.userDisplayName = userDisplayName;
return this;
}
/**
* Get userDisplayName
* @return userDisplayName
**/
@ApiModelProperty(value = "")
public String getUserDisplayName() {
return userDisplayName;
}
public void setUserDisplayName(String userDisplayName) {
this.userDisplayName = userDisplayName;
}
public ChangeMessage userFirstName(String userFirstName) {
this.userFirstName = userFirstName;
return this;
}
/**
* Get userFirstName
* @return userFirstName
**/
@ApiModelProperty(value = "")
public String getUserFirstName() {
return userFirstName;
}
public void setUserFirstName(String userFirstName) {
this.userFirstName = userFirstName;
}
public ChangeMessage userId(String userId) {
this.userId = userId;
return this;
}
/**
* Get userId
* @return userId
**/
@ApiModelProperty(value = "")
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public ChangeMessage userLastName(String userLastName) {
this.userLastName = userLastName;
return this;
}
/**
* Get userLastName
* @return userLastName
**/
@ApiModelProperty(value = "")
public String getUserLastName() {
return userLastName;
}
public void setUserLastName(String userLastName) {
this.userLastName = userLastName;
}
public ChangeMessage verb(String verb) {
this.verb = verb;
return this;
}
/**
* Get verb
* @return verb
**/
@ApiModelProperty(value = "")
public String getVerb() {
return verb;
}
public void setVerb(String verb) {
this.verb = verb;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ChangeMessage changeMessage = (ChangeMessage) o;
return Objects.equals(this.amount, changeMessage.amount) &&
Objects.equals(this.appId, changeMessage.appId) &&
Objects.equals(this.appKey, changeMessage.appKey) &&
Objects.equals(this.appName, changeMessage.appName) &&
Objects.equals(this.attributes, changeMessage.attributes) &&
Objects.equals(this.changeMessageId, changeMessage.changeMessageId) &&
Objects.equals(this.correlationId, changeMessage.correlationId) &&
Objects.equals(this.createdDate, changeMessage.createdDate) &&
Objects.equals(this.identifier, changeMessage.identifier) &&
Objects.equals(this.message, changeMessage.message) &&
Objects.equals(this.metadata, changeMessage.metadata) &&
Objects.equals(this.newValue, changeMessage.newValue) &&
Objects.equals(this.oldValue, changeMessage.oldValue) &&
Objects.equals(this.subject, changeMessage.subject) &&
Objects.equals(this.subjectType, changeMessage.subjectType) &&
Objects.equals(this.success, changeMessage.success) &&
Objects.equals(this.userDisplayName, changeMessage.userDisplayName) &&
Objects.equals(this.userFirstName, changeMessage.userFirstName) &&
Objects.equals(this.userId, changeMessage.userId) &&
Objects.equals(this.userLastName, changeMessage.userLastName) &&
Objects.equals(this.verb, changeMessage.verb);
}
@Override
public int hashCode() {
return Objects.hash(amount, appId, appKey, appName, attributes, changeMessageId, correlationId, createdDate, identifier, message, metadata, newValue, oldValue, subject, subjectType, success, userDisplayName, userFirstName, userId, userLastName, verb);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ChangeMessage {\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" appId: ").append(toIndentedString(appId)).append("\n");
sb.append(" appKey: ").append(toIndentedString(appKey)).append("\n");
sb.append(" appName: ").append(toIndentedString(appName)).append("\n");
sb.append(" attributes: ").append(toIndentedString(attributes)).append("\n");
sb.append(" changeMessageId: ").append(toIndentedString(changeMessageId)).append("\n");
sb.append(" correlationId: ").append(toIndentedString(correlationId)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" identifier: ").append(toIndentedString(identifier)).append("\n");
sb.append(" message: ").append(toIndentedString(message)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" newValue: ").append(toIndentedString(newValue)).append("\n");
sb.append(" oldValue: ").append(toIndentedString(oldValue)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" subjectType: ").append(toIndentedString(subjectType)).append("\n");
sb.append(" success: ").append(toIndentedString(success)).append("\n");
sb.append(" userDisplayName: ").append(toIndentedString(userDisplayName)).append("\n");
sb.append(" userFirstName: ").append(toIndentedString(userFirstName)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" userLastName: ").append(toIndentedString(userLastName)).append("\n");
sb.append(" verb: ").append(toIndentedString(verb)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy