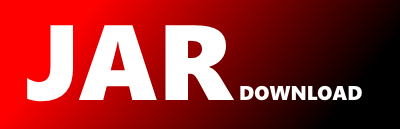
com.kibocommerce.sdk.fulfillment.model.Manifest Maven / Gradle / Ivy
The newest version!
/*
* Kibo Fulfillment API - Production Profile
* REST API backing the Kibo Fulfiller User Interface
*
* OpenAPI spec version: 1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.kibocommerce.sdk.fulfillment.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.kibocommerce.sdk.fulfillment.model.AuditInfo;
import com.kibocommerce.sdk.fulfillment.model.ManifestShipment;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Manifest
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-12-08T12:42:53.880-06:00[America/Chicago]")
public class Manifest {
public static final String SERIALIZED_NAME_ATTRIBUTES = "attributes";
@SerializedName(SERIALIZED_NAME_ATTRIBUTES)
private Map attributes = null;
public static final String SERIALIZED_NAME_AUDIT_INFO = "auditInfo";
@SerializedName(SERIALIZED_NAME_AUDIT_INFO)
private AuditInfo auditInfo = null;
public static final String SERIALIZED_NAME_CARRIER_ID = "carrierId";
@SerializedName(SERIALIZED_NAME_CARRIER_ID)
private String carrierId;
public static final String SERIALIZED_NAME_INTERNAL_ID = "internalId";
@SerializedName(SERIALIZED_NAME_INTERNAL_ID)
private String internalId;
public static final String SERIALIZED_NAME_LOCATION_CODE = "locationCode";
@SerializedName(SERIALIZED_NAME_LOCATION_CODE)
private String locationCode;
public static final String SERIALIZED_NAME_MANIFEST_ID = "manifestId";
@SerializedName(SERIALIZED_NAME_MANIFEST_ID)
private String manifestId;
public static final String SERIALIZED_NAME_MANIFEST_URL = "manifestUrl";
@SerializedName(SERIALIZED_NAME_MANIFEST_URL)
private String manifestUrl;
public static final String SERIALIZED_NAME_NUMBER_OF_PACKAGES = "numberOfPackages";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_PACKAGES)
private Integer numberOfPackages;
public static final String SERIALIZED_NAME_NUMBER_OF_SHIPMENTS = "numberOfShipments";
@SerializedName(SERIALIZED_NAME_NUMBER_OF_SHIPMENTS)
private Integer numberOfShipments;
public static final String SERIALIZED_NAME_SHIPMENTS = "shipments";
@SerializedName(SERIALIZED_NAME_SHIPMENTS)
private List shipments = null;
public static final String SERIALIZED_NAME_TENANT_ID = "tenantId";
@SerializedName(SERIALIZED_NAME_TENANT_ID)
private Integer tenantId;
public static final String SERIALIZED_NAME_USER_DISPLAY_NAME = "userDisplayName";
@SerializedName(SERIALIZED_NAME_USER_DISPLAY_NAME)
private String userDisplayName;
public static final String SERIALIZED_NAME_USER_ID = "userId";
@SerializedName(SERIALIZED_NAME_USER_ID)
private String userId;
public Manifest attributes(Map attributes) {
this.attributes = attributes;
return this;
}
public Manifest putAttributesItem(String key, Object attributesItem) {
if (this.attributes == null) {
this.attributes = new HashMap();
}
this.attributes.put(key, attributesItem);
return this;
}
/**
* Get attributes
* @return attributes
**/
@ApiModelProperty(value = "")
public Map getAttributes() {
return attributes;
}
public void setAttributes(Map attributes) {
this.attributes = attributes;
}
public Manifest auditInfo(AuditInfo auditInfo) {
this.auditInfo = auditInfo;
return this;
}
/**
* Get auditInfo
* @return auditInfo
**/
@ApiModelProperty(value = "")
public AuditInfo getAuditInfo() {
return auditInfo;
}
public void setAuditInfo(AuditInfo auditInfo) {
this.auditInfo = auditInfo;
}
public Manifest carrierId(String carrierId) {
this.carrierId = carrierId;
return this;
}
/**
* Get carrierId
* @return carrierId
**/
@ApiModelProperty(value = "")
public String getCarrierId() {
return carrierId;
}
public void setCarrierId(String carrierId) {
this.carrierId = carrierId;
}
public Manifest internalId(String internalId) {
this.internalId = internalId;
return this;
}
/**
* Get internalId
* @return internalId
**/
@ApiModelProperty(value = "")
public String getInternalId() {
return internalId;
}
public void setInternalId(String internalId) {
this.internalId = internalId;
}
public Manifest locationCode(String locationCode) {
this.locationCode = locationCode;
return this;
}
/**
* Get locationCode
* @return locationCode
**/
@ApiModelProperty(value = "")
public String getLocationCode() {
return locationCode;
}
public void setLocationCode(String locationCode) {
this.locationCode = locationCode;
}
public Manifest manifestId(String manifestId) {
this.manifestId = manifestId;
return this;
}
/**
* Get manifestId
* @return manifestId
**/
@ApiModelProperty(value = "")
public String getManifestId() {
return manifestId;
}
public void setManifestId(String manifestId) {
this.manifestId = manifestId;
}
public Manifest manifestUrl(String manifestUrl) {
this.manifestUrl = manifestUrl;
return this;
}
/**
* Get manifestUrl
* @return manifestUrl
**/
@ApiModelProperty(value = "")
public String getManifestUrl() {
return manifestUrl;
}
public void setManifestUrl(String manifestUrl) {
this.manifestUrl = manifestUrl;
}
public Manifest numberOfPackages(Integer numberOfPackages) {
this.numberOfPackages = numberOfPackages;
return this;
}
/**
* Get numberOfPackages
* @return numberOfPackages
**/
@ApiModelProperty(value = "")
public Integer getNumberOfPackages() {
return numberOfPackages;
}
public void setNumberOfPackages(Integer numberOfPackages) {
this.numberOfPackages = numberOfPackages;
}
public Manifest numberOfShipments(Integer numberOfShipments) {
this.numberOfShipments = numberOfShipments;
return this;
}
/**
* Get numberOfShipments
* @return numberOfShipments
**/
@ApiModelProperty(value = "")
public Integer getNumberOfShipments() {
return numberOfShipments;
}
public void setNumberOfShipments(Integer numberOfShipments) {
this.numberOfShipments = numberOfShipments;
}
public Manifest shipments(List shipments) {
this.shipments = shipments;
return this;
}
public Manifest addShipmentsItem(ManifestShipment shipmentsItem) {
if (this.shipments == null) {
this.shipments = new ArrayList();
}
this.shipments.add(shipmentsItem);
return this;
}
/**
* Get shipments
* @return shipments
**/
@ApiModelProperty(value = "")
public List getShipments() {
return shipments;
}
public void setShipments(List shipments) {
this.shipments = shipments;
}
public Manifest tenantId(Integer tenantId) {
this.tenantId = tenantId;
return this;
}
/**
* Get tenantId
* @return tenantId
**/
@ApiModelProperty(value = "")
public Integer getTenantId() {
return tenantId;
}
public void setTenantId(Integer tenantId) {
this.tenantId = tenantId;
}
public Manifest userDisplayName(String userDisplayName) {
this.userDisplayName = userDisplayName;
return this;
}
/**
* Get userDisplayName
* @return userDisplayName
**/
@ApiModelProperty(value = "")
public String getUserDisplayName() {
return userDisplayName;
}
public void setUserDisplayName(String userDisplayName) {
this.userDisplayName = userDisplayName;
}
public Manifest userId(String userId) {
this.userId = userId;
return this;
}
/**
* Get userId
* @return userId
**/
@ApiModelProperty(value = "")
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Manifest manifest = (Manifest) o;
return Objects.equals(this.attributes, manifest.attributes) &&
Objects.equals(this.auditInfo, manifest.auditInfo) &&
Objects.equals(this.carrierId, manifest.carrierId) &&
Objects.equals(this.internalId, manifest.internalId) &&
Objects.equals(this.locationCode, manifest.locationCode) &&
Objects.equals(this.manifestId, manifest.manifestId) &&
Objects.equals(this.manifestUrl, manifest.manifestUrl) &&
Objects.equals(this.numberOfPackages, manifest.numberOfPackages) &&
Objects.equals(this.numberOfShipments, manifest.numberOfShipments) &&
Objects.equals(this.shipments, manifest.shipments) &&
Objects.equals(this.tenantId, manifest.tenantId) &&
Objects.equals(this.userDisplayName, manifest.userDisplayName) &&
Objects.equals(this.userId, manifest.userId);
}
@Override
public int hashCode() {
return Objects.hash(attributes, auditInfo, carrierId, internalId, locationCode, manifestId, manifestUrl, numberOfPackages, numberOfShipments, shipments, tenantId, userDisplayName, userId);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Manifest {\n");
sb.append(" attributes: ").append(toIndentedString(attributes)).append("\n");
sb.append(" auditInfo: ").append(toIndentedString(auditInfo)).append("\n");
sb.append(" carrierId: ").append(toIndentedString(carrierId)).append("\n");
sb.append(" internalId: ").append(toIndentedString(internalId)).append("\n");
sb.append(" locationCode: ").append(toIndentedString(locationCode)).append("\n");
sb.append(" manifestId: ").append(toIndentedString(manifestId)).append("\n");
sb.append(" manifestUrl: ").append(toIndentedString(manifestUrl)).append("\n");
sb.append(" numberOfPackages: ").append(toIndentedString(numberOfPackages)).append("\n");
sb.append(" numberOfShipments: ").append(toIndentedString(numberOfShipments)).append("\n");
sb.append(" shipments: ").append(toIndentedString(shipments)).append("\n");
sb.append(" tenantId: ").append(toIndentedString(tenantId)).append("\n");
sb.append(" userDisplayName: ").append(toIndentedString(userDisplayName)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy