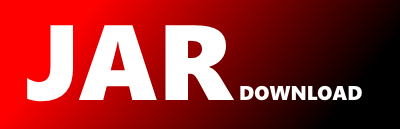
com.kibocommerce.sdk.fulfillment.model.Phone Maven / Gradle / Ivy
The newest version!
/*
* Kibo Fulfillment API - Production Profile
* REST API backing the Kibo Fulfiller User Interface
*
* OpenAPI spec version: 1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.kibocommerce.sdk.fulfillment.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Phone
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-12-08T12:42:53.880-06:00[America/Chicago]")
public class Phone {
public static final String SERIALIZED_NAME_ATTRIBUTES = "attributes";
@SerializedName(SERIALIZED_NAME_ATTRIBUTES)
private Map attributes = null;
public static final String SERIALIZED_NAME_HOME = "home";
@SerializedName(SERIALIZED_NAME_HOME)
private String home;
public static final String SERIALIZED_NAME_MOBILE = "mobile";
@SerializedName(SERIALIZED_NAME_MOBILE)
private String mobile;
public static final String SERIALIZED_NAME_WORK = "work";
@SerializedName(SERIALIZED_NAME_WORK)
private String work;
public Phone attributes(Map attributes) {
this.attributes = attributes;
return this;
}
public Phone putAttributesItem(String key, Object attributesItem) {
if (this.attributes == null) {
this.attributes = new HashMap();
}
this.attributes.put(key, attributesItem);
return this;
}
/**
* Get attributes
* @return attributes
**/
@ApiModelProperty(value = "")
public Map getAttributes() {
return attributes;
}
public void setAttributes(Map attributes) {
this.attributes = attributes;
}
public Phone home(String home) {
this.home = home;
return this;
}
/**
* Get home
* @return home
**/
@ApiModelProperty(value = "")
public String getHome() {
return home;
}
public void setHome(String home) {
this.home = home;
}
public Phone mobile(String mobile) {
this.mobile = mobile;
return this;
}
/**
* Get mobile
* @return mobile
**/
@ApiModelProperty(value = "")
public String getMobile() {
return mobile;
}
public void setMobile(String mobile) {
this.mobile = mobile;
}
public Phone work(String work) {
this.work = work;
return this;
}
/**
* Get work
* @return work
**/
@ApiModelProperty(value = "")
public String getWork() {
return work;
}
public void setWork(String work) {
this.work = work;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Phone phone = (Phone) o;
return Objects.equals(this.attributes, phone.attributes) &&
Objects.equals(this.home, phone.home) &&
Objects.equals(this.mobile, phone.mobile) &&
Objects.equals(this.work, phone.work);
}
@Override
public int hashCode() {
return Objects.hash(attributes, home, mobile, work);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Phone {\n");
sb.append(" attributes: ").append(toIndentedString(attributes)).append("\n");
sb.append(" home: ").append(toIndentedString(home)).append("\n");
sb.append(" mobile: ").append(toIndentedString(mobile)).append("\n");
sb.append(" work: ").append(toIndentedString(work)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy