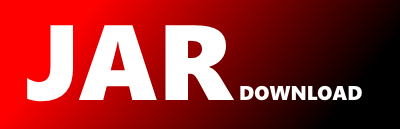
com.kibocommerce.sdk.fulfillment.model.Shipment Maven / Gradle / Ivy
The newest version!
/*
* Kibo Fulfillment API - Production Profile
* REST API backing the Kibo Fulfiller User Interface
*
* OpenAPI spec version: 1.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.kibocommerce.sdk.fulfillment.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.kibocommerce.sdk.fulfillment.model.AuditInfo;
import com.kibocommerce.sdk.fulfillment.model.CanceledItem;
import com.kibocommerce.sdk.fulfillment.model.ChangeMessage;
import com.kibocommerce.sdk.fulfillment.model.Customer;
import com.kibocommerce.sdk.fulfillment.model.Destination;
import com.kibocommerce.sdk.fulfillment.model.Item;
import com.kibocommerce.sdk.fulfillment.model.ModelPackage;
import com.kibocommerce.sdk.fulfillment.model.ReassignItem;
import com.kibocommerce.sdk.fulfillment.model.RejectedItem;
import com.kibocommerce.sdk.fulfillment.model.ShipmentStatusReason;
import com.kibocommerce.sdk.fulfillment.model.WorkflowState;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.threeten.bp.OffsetDateTime;
/**
* Shipment
*/
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2020-12-08T12:42:53.880-06:00[America/Chicago]")
public class Shipment {
public static final String SERIALIZED_NAME_ACCEPTED_DATE = "acceptedDate";
@SerializedName(SERIALIZED_NAME_ACCEPTED_DATE)
private OffsetDateTime acceptedDate;
public static final String SERIALIZED_NAME_ASSIGNED_LOCATION_CODE = "assignedLocationCode";
@SerializedName(SERIALIZED_NAME_ASSIGNED_LOCATION_CODE)
private String assignedLocationCode;
public static final String SERIALIZED_NAME_ATTRIBUTES = "attributes";
@SerializedName(SERIALIZED_NAME_ATTRIBUTES)
private Map attributes = null;
public static final String SERIALIZED_NAME_AUDIT_INFO = "auditInfo";
@SerializedName(SERIALIZED_NAME_AUDIT_INFO)
private AuditInfo auditInfo = null;
public static final String SERIALIZED_NAME_CANCELED_ITEMS = "canceledItems";
@SerializedName(SERIALIZED_NAME_CANCELED_ITEMS)
private List canceledItems = null;
public static final String SERIALIZED_NAME_CHANGE_MESSAGES = "changeMessages";
@SerializedName(SERIALIZED_NAME_CHANGE_MESSAGES)
private List changeMessages = null;
public static final String SERIALIZED_NAME_CHILD_SHIPMENT_NUMBERS = "childShipmentNumbers";
@SerializedName(SERIALIZED_NAME_CHILD_SHIPMENT_NUMBERS)
private List childShipmentNumbers = null;
public static final String SERIALIZED_NAME_CURRENCY_CODE = "currencyCode";
@SerializedName(SERIALIZED_NAME_CURRENCY_CODE)
private String currencyCode;
public static final String SERIALIZED_NAME_CUSTOMER = "customer";
@SerializedName(SERIALIZED_NAME_CUSTOMER)
private Customer customer = null;
public static final String SERIALIZED_NAME_CUSTOMER_ACCOUNT_ID = "customerAccountId";
@SerializedName(SERIALIZED_NAME_CUSTOMER_ACCOUNT_ID)
private Integer customerAccountId;
public static final String SERIALIZED_NAME_CUSTOMER_ADDRESS_ID = "customerAddressId";
@SerializedName(SERIALIZED_NAME_CUSTOMER_ADDRESS_ID)
private Integer customerAddressId;
public static final String SERIALIZED_NAME_CUSTOMER_TAX_ID = "customerTaxId";
@SerializedName(SERIALIZED_NAME_CUSTOMER_TAX_ID)
private String customerTaxId;
public static final String SERIALIZED_NAME_DATA = "data";
@SerializedName(SERIALIZED_NAME_DATA)
private Map data = null;
public static final String SERIALIZED_NAME_DESTINATION = "destination";
@SerializedName(SERIALIZED_NAME_DESTINATION)
private Destination destination = null;
public static final String SERIALIZED_NAME_DUTY_ADJUSTMENT = "dutyAdjustment";
@SerializedName(SERIALIZED_NAME_DUTY_ADJUSTMENT)
private BigDecimal dutyAdjustment;
public static final String SERIALIZED_NAME_DUTY_TOTAL = "dutyTotal";
@SerializedName(SERIALIZED_NAME_DUTY_TOTAL)
private BigDecimal dutyTotal;
public static final String SERIALIZED_NAME_EMAIL = "email";
@SerializedName(SERIALIZED_NAME_EMAIL)
private String email;
public static final String SERIALIZED_NAME_EXTERNAL_ORDER_ID = "externalOrderId";
@SerializedName(SERIALIZED_NAME_EXTERNAL_ORDER_ID)
private String externalOrderId;
public static final String SERIALIZED_NAME_FULFILLMENT_DATE = "fulfillmentDate";
@SerializedName(SERIALIZED_NAME_FULFILLMENT_DATE)
private OffsetDateTime fulfillmentDate;
public static final String SERIALIZED_NAME_FULFILLMENT_LOCATION_CODE = "fulfillmentLocationCode";
@SerializedName(SERIALIZED_NAME_FULFILLMENT_LOCATION_CODE)
private String fulfillmentLocationCode;
/**
* Gets or Sets fulfillmentStatus
*/
@JsonAdapter(FulfillmentStatusEnum.Adapter.class)
public enum FulfillmentStatusEnum {
NOTFULFILLED("NotFulfilled"),
FULFILLED("Fulfilled");
private String value;
FulfillmentStatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static FulfillmentStatusEnum fromValue(String text) {
for (FulfillmentStatusEnum b : FulfillmentStatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + text + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final FulfillmentStatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public FulfillmentStatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return FulfillmentStatusEnum.fromValue(String.valueOf(value));
}
}
}
public static final String SERIALIZED_NAME_FULFILLMENT_STATUS = "fulfillmentStatus";
@SerializedName(SERIALIZED_NAME_FULFILLMENT_STATUS)
private FulfillmentStatusEnum fulfillmentStatus;
public static final String SERIALIZED_NAME_HANDLING_ADJUSTMENT = "handlingAdjustment";
@SerializedName(SERIALIZED_NAME_HANDLING_ADJUSTMENT)
private BigDecimal handlingAdjustment;
public static final String SERIALIZED_NAME_HANDLING_SUBTOTAL = "handlingSubtotal";
@SerializedName(SERIALIZED_NAME_HANDLING_SUBTOTAL)
private BigDecimal handlingSubtotal;
public static final String SERIALIZED_NAME_HANDLING_TAX_ADJUSTMENT = "handlingTaxAdjustment";
@SerializedName(SERIALIZED_NAME_HANDLING_TAX_ADJUSTMENT)
private BigDecimal handlingTaxAdjustment;
public static final String SERIALIZED_NAME_HANDLING_TAX_TOTAL = "handlingTaxTotal";
@SerializedName(SERIALIZED_NAME_HANDLING_TAX_TOTAL)
private BigDecimal handlingTaxTotal;
public static final String SERIALIZED_NAME_HANDLING_TOTAL = "handlingTotal";
@SerializedName(SERIALIZED_NAME_HANDLING_TOTAL)
private BigDecimal handlingTotal;
public static final String SERIALIZED_NAME_IS_EXPRESS = "isExpress";
@SerializedName(SERIALIZED_NAME_IS_EXPRESS)
private Boolean isExpress;
public static final String SERIALIZED_NAME_IS_OPT_IN_FOR_SMS = "isOptInForSms";
@SerializedName(SERIALIZED_NAME_IS_OPT_IN_FOR_SMS)
private Boolean isOptInForSms;
public static final String SERIALIZED_NAME_ITEMS = "items";
@SerializedName(SERIALIZED_NAME_ITEMS)
private List- items = null;
public static final String SERIALIZED_NAME_LINE_ITEM_SUBTOTAL = "lineItemSubtotal";
@SerializedName(SERIALIZED_NAME_LINE_ITEM_SUBTOTAL)
private BigDecimal lineItemSubtotal;
public static final String SERIALIZED_NAME_LINE_ITEM_TAX_ADJUSTMENT = "lineItemTaxAdjustment";
@SerializedName(SERIALIZED_NAME_LINE_ITEM_TAX_ADJUSTMENT)
private BigDecimal lineItemTaxAdjustment;
public static final String SERIALIZED_NAME_LINE_ITEM_TAX_TOTAL = "lineItemTaxTotal";
@SerializedName(SERIALIZED_NAME_LINE_ITEM_TAX_TOTAL)
private BigDecimal lineItemTaxTotal;
public static final String SERIALIZED_NAME_LINE_ITEM_TOTAL = "lineItemTotal";
@SerializedName(SERIALIZED_NAME_LINE_ITEM_TOTAL)
private BigDecimal lineItemTotal;
public static final String SERIALIZED_NAME_ORDER_ID = "orderId";
@SerializedName(SERIALIZED_NAME_ORDER_ID)
private String orderId;
public static final String SERIALIZED_NAME_ORDER_NUMBER = "orderNumber";
@SerializedName(SERIALIZED_NAME_ORDER_NUMBER)
private Integer orderNumber;
public static final String SERIALIZED_NAME_ORDER_SUBMIT_DATE = "orderSubmitDate";
@SerializedName(SERIALIZED_NAME_ORDER_SUBMIT_DATE)
private OffsetDateTime orderSubmitDate;
public static final String SERIALIZED_NAME_ORIGINAL_SHIPMENT_NUMBER = "originalShipmentNumber";
@SerializedName(SERIALIZED_NAME_ORIGINAL_SHIPMENT_NUMBER)
private Integer originalShipmentNumber;
public static final String SERIALIZED_NAME_PACKAGES = "packages";
@SerializedName(SERIALIZED_NAME_PACKAGES)
private List
packages = null;
public static final String SERIALIZED_NAME_PARENT_SHIPMENT_NUMBER = "parentShipmentNumber";
@SerializedName(SERIALIZED_NAME_PARENT_SHIPMENT_NUMBER)
private Integer parentShipmentNumber;
/**
* Gets or Sets pickStatus
*/
@JsonAdapter(PickStatusEnum.Adapter.class)
public enum PickStatusEnum {
AVAILABLE("AVAILABLE"),
IN_WAVE("IN_WAVE"),
PICKED("PICKED"),
TRANSFER("TRANSFER"),
COMPLETE("COMPLETE");
private String value;
PickStatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PickStatusEnum fromValue(String text) {
for (PickStatusEnum b : PickStatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + text + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PickStatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PickStatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PickStatusEnum.fromValue(String.valueOf(value));
}
}
}
public static final String SERIALIZED_NAME_PICK_STATUS = "pickStatus";
@SerializedName(SERIALIZED_NAME_PICK_STATUS)
private PickStatusEnum pickStatus;
/**
* Gets or Sets pickType
*/
@JsonAdapter(PickTypeEnum.Adapter.class)
public enum PickTypeEnum {
NORMAL("NORMAL"),
SINGLE("SINGLE"),
MULTIPLE("MULTIPLE");
private String value;
PickTypeEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static PickTypeEnum fromValue(String text) {
for (PickTypeEnum b : PickTypeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + text + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final PickTypeEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public PickTypeEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return PickTypeEnum.fromValue(String.valueOf(value));
}
}
}
public static final String SERIALIZED_NAME_PICK_TYPE = "pickType";
@SerializedName(SERIALIZED_NAME_PICK_TYPE)
private PickTypeEnum pickType;
public static final String SERIALIZED_NAME_PICK_WAVE_NUMBER = "pickWaveNumber";
@SerializedName(SERIALIZED_NAME_PICK_WAVE_NUMBER)
private Integer pickWaveNumber;
public static final String SERIALIZED_NAME_PICKUP_INFO = "pickupInfo";
@SerializedName(SERIALIZED_NAME_PICKUP_INFO)
private Map pickupInfo = null;
public static final String SERIALIZED_NAME_READY_FOR_PICKUP = "readyForPickup";
@SerializedName(SERIALIZED_NAME_READY_FOR_PICKUP)
private Boolean readyForPickup;
public static final String SERIALIZED_NAME_READY_FOR_PICKUP_DATE = "readyForPickupDate";
@SerializedName(SERIALIZED_NAME_READY_FOR_PICKUP_DATE)
private OffsetDateTime readyForPickupDate;
public static final String SERIALIZED_NAME_READY_TO_CAPTURE = "readyToCapture";
@SerializedName(SERIALIZED_NAME_READY_TO_CAPTURE)
private Boolean readyToCapture;
public static final String SERIALIZED_NAME_REASSIGNED_ITEMS = "reassignedItems";
@SerializedName(SERIALIZED_NAME_REASSIGNED_ITEMS)
private List reassignedItems = null;
public static final String SERIALIZED_NAME_RECEIVED_DATE = "receivedDate";
@SerializedName(SERIALIZED_NAME_RECEIVED_DATE)
private OffsetDateTime receivedDate;
public static final String SERIALIZED_NAME_REJECTED_ITEMS = "rejectedItems";
@SerializedName(SERIALIZED_NAME_REJECTED_ITEMS)
private List rejectedItems = null;
public static final String SERIALIZED_NAME_SENT_CUSTOMER_AT_STORE_NOTIFICATION = "sentCustomerAtStoreNotification";
@SerializedName(SERIALIZED_NAME_SENT_CUSTOMER_AT_STORE_NOTIFICATION)
private Boolean sentCustomerAtStoreNotification;
public static final String SERIALIZED_NAME_SENT_CUSTOMER_IN_TRANSIT_NOTIFICATION = "sentCustomerInTransitNotification";
@SerializedName(SERIALIZED_NAME_SENT_CUSTOMER_IN_TRANSIT_NOTIFICATION)
private Boolean sentCustomerInTransitNotification;
public static final String SERIALIZED_NAME_SHIPMENT_ADJUSTMENT = "shipmentAdjustment";
@SerializedName(SERIALIZED_NAME_SHIPMENT_ADJUSTMENT)
private BigDecimal shipmentAdjustment;
public static final String SERIALIZED_NAME_SHIPMENT_NUMBER = "shipmentNumber";
@SerializedName(SERIALIZED_NAME_SHIPMENT_NUMBER)
private Integer shipmentNumber;
/**
* Gets or Sets shipmentStatus
*/
@JsonAdapter(ShipmentStatusEnum.Adapter.class)
public enum ShipmentStatusEnum {
READY("READY"),
REASSIGNED("REASSIGNED"),
BACKORDER("BACKORDER"),
CANCELED("CANCELED"),
FULFILLED("FULFILLED"),
CUSTOMER_CARE("CUSTOMER_CARE");
private String value;
ShipmentStatusEnum(String value) {
this.value = value;
}
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
public static ShipmentStatusEnum fromValue(String text) {
for (ShipmentStatusEnum b : ShipmentStatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + text + "'");
}
public static class Adapter extends TypeAdapter {
@Override
public void write(final JsonWriter jsonWriter, final ShipmentStatusEnum enumeration) throws IOException {
jsonWriter.value(enumeration.getValue());
}
@Override
public ShipmentStatusEnum read(final JsonReader jsonReader) throws IOException {
String value = jsonReader.nextString();
return ShipmentStatusEnum.fromValue(String.valueOf(value));
}
}
}
public static final String SERIALIZED_NAME_SHIPMENT_STATUS = "shipmentStatus";
@SerializedName(SERIALIZED_NAME_SHIPMENT_STATUS)
private ShipmentStatusEnum shipmentStatus;
public static final String SERIALIZED_NAME_SHIPMENT_STATUS_REASON = "shipmentStatusReason";
@SerializedName(SERIALIZED_NAME_SHIPMENT_STATUS_REASON)
private ShipmentStatusReason shipmentStatusReason = null;
public static final String SERIALIZED_NAME_SHIPMENT_TYPE = "shipmentType";
@SerializedName(SERIALIZED_NAME_SHIPMENT_TYPE)
private String shipmentType;
public static final String SERIALIZED_NAME_SHIPPING_ADJUSTMENT = "shippingAdjustment";
@SerializedName(SERIALIZED_NAME_SHIPPING_ADJUSTMENT)
private BigDecimal shippingAdjustment;
public static final String SERIALIZED_NAME_SHIPPING_METHOD_CODE = "shippingMethodCode";
@SerializedName(SERIALIZED_NAME_SHIPPING_METHOD_CODE)
private String shippingMethodCode;
public static final String SERIALIZED_NAME_SHIPPING_METHOD_NAME = "shippingMethodName";
@SerializedName(SERIALIZED_NAME_SHIPPING_METHOD_NAME)
private String shippingMethodName;
public static final String SERIALIZED_NAME_SHIPPING_SUBTOTAL = "shippingSubtotal";
@SerializedName(SERIALIZED_NAME_SHIPPING_SUBTOTAL)
private BigDecimal shippingSubtotal;
public static final String SERIALIZED_NAME_SHIPPING_TAX_ADJUSTMENT = "shippingTaxAdjustment";
@SerializedName(SERIALIZED_NAME_SHIPPING_TAX_ADJUSTMENT)
private BigDecimal shippingTaxAdjustment;
public static final String SERIALIZED_NAME_SHIPPING_TAX_TOTAL = "shippingTaxTotal";
@SerializedName(SERIALIZED_NAME_SHIPPING_TAX_TOTAL)
private BigDecimal shippingTaxTotal;
public static final String SERIALIZED_NAME_SHIPPING_TOTAL = "shippingTotal";
@SerializedName(SERIALIZED_NAME_SHIPPING_TOTAL)
private BigDecimal shippingTotal;
public static final String SERIALIZED_NAME_SITE_ID = "siteId";
@SerializedName(SERIALIZED_NAME_SITE_ID)
private Integer siteId;
public static final String SERIALIZED_NAME_TAX_DATA = "taxData";
@SerializedName(SERIALIZED_NAME_TAX_DATA)
private Object taxData = null;
public static final String SERIALIZED_NAME_TENANT_ID = "tenantId";
@SerializedName(SERIALIZED_NAME_TENANT_ID)
private Integer tenantId;
public static final String SERIALIZED_NAME_TOTAL = "total";
@SerializedName(SERIALIZED_NAME_TOTAL)
private BigDecimal total;
public static final String SERIALIZED_NAME_TRANSFER_SHIPMENT_NUMBERS = "transferShipmentNumbers";
@SerializedName(SERIALIZED_NAME_TRANSFER_SHIPMENT_NUMBERS)
private List transferShipmentNumbers = null;
public static final String SERIALIZED_NAME_TRANSIT_TIME = "transitTime";
@SerializedName(SERIALIZED_NAME_TRANSIT_TIME)
private String transitTime;
public static final String SERIALIZED_NAME_USER_ID = "userId";
@SerializedName(SERIALIZED_NAME_USER_ID)
private String userId;
public static final String SERIALIZED_NAME_WORKFLOW_PROCESS_CONTAINER_ID = "workflowProcessContainerId";
@SerializedName(SERIALIZED_NAME_WORKFLOW_PROCESS_CONTAINER_ID)
private String workflowProcessContainerId;
public static final String SERIALIZED_NAME_WORKFLOW_PROCESS_ID = "workflowProcessId";
@SerializedName(SERIALIZED_NAME_WORKFLOW_PROCESS_ID)
private String workflowProcessId;
public static final String SERIALIZED_NAME_WORKFLOW_STATE = "workflowState";
@SerializedName(SERIALIZED_NAME_WORKFLOW_STATE)
private WorkflowState workflowState = null;
public Shipment acceptedDate(OffsetDateTime acceptedDate) {
this.acceptedDate = acceptedDate;
return this;
}
/**
* Get acceptedDate
* @return acceptedDate
**/
@ApiModelProperty(value = "")
public OffsetDateTime getAcceptedDate() {
return acceptedDate;
}
public void setAcceptedDate(OffsetDateTime acceptedDate) {
this.acceptedDate = acceptedDate;
}
public Shipment assignedLocationCode(String assignedLocationCode) {
this.assignedLocationCode = assignedLocationCode;
return this;
}
/**
* Get assignedLocationCode
* @return assignedLocationCode
**/
@ApiModelProperty(value = "")
public String getAssignedLocationCode() {
return assignedLocationCode;
}
public void setAssignedLocationCode(String assignedLocationCode) {
this.assignedLocationCode = assignedLocationCode;
}
public Shipment attributes(Map attributes) {
this.attributes = attributes;
return this;
}
public Shipment putAttributesItem(String key, Object attributesItem) {
if (this.attributes == null) {
this.attributes = new HashMap();
}
this.attributes.put(key, attributesItem);
return this;
}
/**
* Get attributes
* @return attributes
**/
@ApiModelProperty(value = "")
public Map getAttributes() {
return attributes;
}
public void setAttributes(Map attributes) {
this.attributes = attributes;
}
public Shipment auditInfo(AuditInfo auditInfo) {
this.auditInfo = auditInfo;
return this;
}
/**
* Get auditInfo
* @return auditInfo
**/
@ApiModelProperty(value = "")
public AuditInfo getAuditInfo() {
return auditInfo;
}
public void setAuditInfo(AuditInfo auditInfo) {
this.auditInfo = auditInfo;
}
public Shipment canceledItems(List canceledItems) {
this.canceledItems = canceledItems;
return this;
}
public Shipment addCanceledItemsItem(CanceledItem canceledItemsItem) {
if (this.canceledItems == null) {
this.canceledItems = new ArrayList();
}
this.canceledItems.add(canceledItemsItem);
return this;
}
/**
* Get canceledItems
* @return canceledItems
**/
@ApiModelProperty(value = "")
public List getCanceledItems() {
return canceledItems;
}
public void setCanceledItems(List canceledItems) {
this.canceledItems = canceledItems;
}
public Shipment changeMessages(List changeMessages) {
this.changeMessages = changeMessages;
return this;
}
public Shipment addChangeMessagesItem(ChangeMessage changeMessagesItem) {
if (this.changeMessages == null) {
this.changeMessages = new ArrayList();
}
this.changeMessages.add(changeMessagesItem);
return this;
}
/**
* Get changeMessages
* @return changeMessages
**/
@ApiModelProperty(value = "")
public List getChangeMessages() {
return changeMessages;
}
public void setChangeMessages(List changeMessages) {
this.changeMessages = changeMessages;
}
public Shipment childShipmentNumbers(List childShipmentNumbers) {
this.childShipmentNumbers = childShipmentNumbers;
return this;
}
public Shipment addChildShipmentNumbersItem(Integer childShipmentNumbersItem) {
if (this.childShipmentNumbers == null) {
this.childShipmentNumbers = new ArrayList();
}
this.childShipmentNumbers.add(childShipmentNumbersItem);
return this;
}
/**
* Get childShipmentNumbers
* @return childShipmentNumbers
**/
@ApiModelProperty(value = "")
public List getChildShipmentNumbers() {
return childShipmentNumbers;
}
public void setChildShipmentNumbers(List childShipmentNumbers) {
this.childShipmentNumbers = childShipmentNumbers;
}
public Shipment currencyCode(String currencyCode) {
this.currencyCode = currencyCode;
return this;
}
/**
* Get currencyCode
* @return currencyCode
**/
@ApiModelProperty(value = "")
public String getCurrencyCode() {
return currencyCode;
}
public void setCurrencyCode(String currencyCode) {
this.currencyCode = currencyCode;
}
public Shipment customer(Customer customer) {
this.customer = customer;
return this;
}
/**
* Get customer
* @return customer
**/
@ApiModelProperty(value = "")
public Customer getCustomer() {
return customer;
}
public void setCustomer(Customer customer) {
this.customer = customer;
}
public Shipment customerAccountId(Integer customerAccountId) {
this.customerAccountId = customerAccountId;
return this;
}
/**
* Get customerAccountId
* @return customerAccountId
**/
@ApiModelProperty(value = "")
public Integer getCustomerAccountId() {
return customerAccountId;
}
public void setCustomerAccountId(Integer customerAccountId) {
this.customerAccountId = customerAccountId;
}
public Shipment customerAddressId(Integer customerAddressId) {
this.customerAddressId = customerAddressId;
return this;
}
/**
* Get customerAddressId
* @return customerAddressId
**/
@ApiModelProperty(value = "")
public Integer getCustomerAddressId() {
return customerAddressId;
}
public void setCustomerAddressId(Integer customerAddressId) {
this.customerAddressId = customerAddressId;
}
public Shipment customerTaxId(String customerTaxId) {
this.customerTaxId = customerTaxId;
return this;
}
/**
* Get customerTaxId
* @return customerTaxId
**/
@ApiModelProperty(value = "")
public String getCustomerTaxId() {
return customerTaxId;
}
public void setCustomerTaxId(String customerTaxId) {
this.customerTaxId = customerTaxId;
}
public Shipment data(Map data) {
this.data = data;
return this;
}
public Shipment putDataItem(String key, Object dataItem) {
if (this.data == null) {
this.data = new HashMap();
}
this.data.put(key, dataItem);
return this;
}
/**
* Get data
* @return data
**/
@ApiModelProperty(value = "")
public Map getData() {
return data;
}
public void setData(Map data) {
this.data = data;
}
public Shipment destination(Destination destination) {
this.destination = destination;
return this;
}
/**
* Get destination
* @return destination
**/
@ApiModelProperty(value = "")
public Destination getDestination() {
return destination;
}
public void setDestination(Destination destination) {
this.destination = destination;
}
public Shipment dutyAdjustment(BigDecimal dutyAdjustment) {
this.dutyAdjustment = dutyAdjustment;
return this;
}
/**
* Get dutyAdjustment
* @return dutyAdjustment
**/
@ApiModelProperty(value = "")
public BigDecimal getDutyAdjustment() {
return dutyAdjustment;
}
public void setDutyAdjustment(BigDecimal dutyAdjustment) {
this.dutyAdjustment = dutyAdjustment;
}
public Shipment dutyTotal(BigDecimal dutyTotal) {
this.dutyTotal = dutyTotal;
return this;
}
/**
* Get dutyTotal
* @return dutyTotal
**/
@ApiModelProperty(value = "")
public BigDecimal getDutyTotal() {
return dutyTotal;
}
public void setDutyTotal(BigDecimal dutyTotal) {
this.dutyTotal = dutyTotal;
}
public Shipment email(String email) {
this.email = email;
return this;
}
/**
* Get email
* @return email
**/
@ApiModelProperty(value = "")
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Shipment externalOrderId(String externalOrderId) {
this.externalOrderId = externalOrderId;
return this;
}
/**
* Get externalOrderId
* @return externalOrderId
**/
@ApiModelProperty(value = "")
public String getExternalOrderId() {
return externalOrderId;
}
public void setExternalOrderId(String externalOrderId) {
this.externalOrderId = externalOrderId;
}
public Shipment fulfillmentDate(OffsetDateTime fulfillmentDate) {
this.fulfillmentDate = fulfillmentDate;
return this;
}
/**
* Get fulfillmentDate
* @return fulfillmentDate
**/
@ApiModelProperty(value = "")
public OffsetDateTime getFulfillmentDate() {
return fulfillmentDate;
}
public void setFulfillmentDate(OffsetDateTime fulfillmentDate) {
this.fulfillmentDate = fulfillmentDate;
}
public Shipment fulfillmentLocationCode(String fulfillmentLocationCode) {
this.fulfillmentLocationCode = fulfillmentLocationCode;
return this;
}
/**
* Get fulfillmentLocationCode
* @return fulfillmentLocationCode
**/
@ApiModelProperty(value = "")
public String getFulfillmentLocationCode() {
return fulfillmentLocationCode;
}
public void setFulfillmentLocationCode(String fulfillmentLocationCode) {
this.fulfillmentLocationCode = fulfillmentLocationCode;
}
public Shipment fulfillmentStatus(FulfillmentStatusEnum fulfillmentStatus) {
this.fulfillmentStatus = fulfillmentStatus;
return this;
}
/**
* Get fulfillmentStatus
* @return fulfillmentStatus
**/
@ApiModelProperty(value = "")
public FulfillmentStatusEnum getFulfillmentStatus() {
return fulfillmentStatus;
}
public void setFulfillmentStatus(FulfillmentStatusEnum fulfillmentStatus) {
this.fulfillmentStatus = fulfillmentStatus;
}
public Shipment handlingAdjustment(BigDecimal handlingAdjustment) {
this.handlingAdjustment = handlingAdjustment;
return this;
}
/**
* Get handlingAdjustment
* @return handlingAdjustment
**/
@ApiModelProperty(value = "")
public BigDecimal getHandlingAdjustment() {
return handlingAdjustment;
}
public void setHandlingAdjustment(BigDecimal handlingAdjustment) {
this.handlingAdjustment = handlingAdjustment;
}
public Shipment handlingSubtotal(BigDecimal handlingSubtotal) {
this.handlingSubtotal = handlingSubtotal;
return this;
}
/**
* Get handlingSubtotal
* @return handlingSubtotal
**/
@ApiModelProperty(value = "")
public BigDecimal getHandlingSubtotal() {
return handlingSubtotal;
}
public void setHandlingSubtotal(BigDecimal handlingSubtotal) {
this.handlingSubtotal = handlingSubtotal;
}
public Shipment handlingTaxAdjustment(BigDecimal handlingTaxAdjustment) {
this.handlingTaxAdjustment = handlingTaxAdjustment;
return this;
}
/**
* Get handlingTaxAdjustment
* @return handlingTaxAdjustment
**/
@ApiModelProperty(value = "")
public BigDecimal getHandlingTaxAdjustment() {
return handlingTaxAdjustment;
}
public void setHandlingTaxAdjustment(BigDecimal handlingTaxAdjustment) {
this.handlingTaxAdjustment = handlingTaxAdjustment;
}
public Shipment handlingTaxTotal(BigDecimal handlingTaxTotal) {
this.handlingTaxTotal = handlingTaxTotal;
return this;
}
/**
* Get handlingTaxTotal
* @return handlingTaxTotal
**/
@ApiModelProperty(value = "")
public BigDecimal getHandlingTaxTotal() {
return handlingTaxTotal;
}
public void setHandlingTaxTotal(BigDecimal handlingTaxTotal) {
this.handlingTaxTotal = handlingTaxTotal;
}
public Shipment handlingTotal(BigDecimal handlingTotal) {
this.handlingTotal = handlingTotal;
return this;
}
/**
* Get handlingTotal
* @return handlingTotal
**/
@ApiModelProperty(value = "")
public BigDecimal getHandlingTotal() {
return handlingTotal;
}
public void setHandlingTotal(BigDecimal handlingTotal) {
this.handlingTotal = handlingTotal;
}
public Shipment isExpress(Boolean isExpress) {
this.isExpress = isExpress;
return this;
}
/**
* Get isExpress
* @return isExpress
**/
@ApiModelProperty(value = "")
public Boolean getIsExpress() {
return isExpress;
}
public void setIsExpress(Boolean isExpress) {
this.isExpress = isExpress;
}
public Shipment isOptInForSms(Boolean isOptInForSms) {
this.isOptInForSms = isOptInForSms;
return this;
}
/**
* Get isOptInForSms
* @return isOptInForSms
**/
@ApiModelProperty(value = "")
public Boolean getIsOptInForSms() {
return isOptInForSms;
}
public void setIsOptInForSms(Boolean isOptInForSms) {
this.isOptInForSms = isOptInForSms;
}
public Shipment items(List- items) {
this.items = items;
return this;
}
public Shipment addItemsItem(Item itemsItem) {
if (this.items == null) {
this.items = new ArrayList
- ();
}
this.items.add(itemsItem);
return this;
}
/**
* Get items
* @return items
**/
@ApiModelProperty(value = "")
public List
- getItems() {
return items;
}
public void setItems(List
- items) {
this.items = items;
}
public Shipment lineItemSubtotal(BigDecimal lineItemSubtotal) {
this.lineItemSubtotal = lineItemSubtotal;
return this;
}
/**
* Get lineItemSubtotal
* @return lineItemSubtotal
**/
@ApiModelProperty(value = "")
public BigDecimal getLineItemSubtotal() {
return lineItemSubtotal;
}
public void setLineItemSubtotal(BigDecimal lineItemSubtotal) {
this.lineItemSubtotal = lineItemSubtotal;
}
public Shipment lineItemTaxAdjustment(BigDecimal lineItemTaxAdjustment) {
this.lineItemTaxAdjustment = lineItemTaxAdjustment;
return this;
}
/**
* Get lineItemTaxAdjustment
* @return lineItemTaxAdjustment
**/
@ApiModelProperty(value = "")
public BigDecimal getLineItemTaxAdjustment() {
return lineItemTaxAdjustment;
}
public void setLineItemTaxAdjustment(BigDecimal lineItemTaxAdjustment) {
this.lineItemTaxAdjustment = lineItemTaxAdjustment;
}
public Shipment lineItemTaxTotal(BigDecimal lineItemTaxTotal) {
this.lineItemTaxTotal = lineItemTaxTotal;
return this;
}
/**
* Get lineItemTaxTotal
* @return lineItemTaxTotal
**/
@ApiModelProperty(value = "")
public BigDecimal getLineItemTaxTotal() {
return lineItemTaxTotal;
}
public void setLineItemTaxTotal(BigDecimal lineItemTaxTotal) {
this.lineItemTaxTotal = lineItemTaxTotal;
}
public Shipment lineItemTotal(BigDecimal lineItemTotal) {
this.lineItemTotal = lineItemTotal;
return this;
}
/**
* Get lineItemTotal
* @return lineItemTotal
**/
@ApiModelProperty(value = "")
public BigDecimal getLineItemTotal() {
return lineItemTotal;
}
public void setLineItemTotal(BigDecimal lineItemTotal) {
this.lineItemTotal = lineItemTotal;
}
public Shipment orderId(String orderId) {
this.orderId = orderId;
return this;
}
/**
* Get orderId
* @return orderId
**/
@ApiModelProperty(value = "")
public String getOrderId() {
return orderId;
}
public void setOrderId(String orderId) {
this.orderId = orderId;
}
public Shipment orderNumber(Integer orderNumber) {
this.orderNumber = orderNumber;
return this;
}
/**
* Get orderNumber
* @return orderNumber
**/
@ApiModelProperty(value = "")
public Integer getOrderNumber() {
return orderNumber;
}
public void setOrderNumber(Integer orderNumber) {
this.orderNumber = orderNumber;
}
public Shipment orderSubmitDate(OffsetDateTime orderSubmitDate) {
this.orderSubmitDate = orderSubmitDate;
return this;
}
/**
* Get orderSubmitDate
* @return orderSubmitDate
**/
@ApiModelProperty(value = "")
public OffsetDateTime getOrderSubmitDate() {
return orderSubmitDate;
}
public void setOrderSubmitDate(OffsetDateTime orderSubmitDate) {
this.orderSubmitDate = orderSubmitDate;
}
public Shipment originalShipmentNumber(Integer originalShipmentNumber) {
this.originalShipmentNumber = originalShipmentNumber;
return this;
}
/**
* Get originalShipmentNumber
* @return originalShipmentNumber
**/
@ApiModelProperty(value = "")
public Integer getOriginalShipmentNumber() {
return originalShipmentNumber;
}
public void setOriginalShipmentNumber(Integer originalShipmentNumber) {
this.originalShipmentNumber = originalShipmentNumber;
}
public Shipment packages(List
packages) {
this.packages = packages;
return this;
}
public Shipment addPackagesItem(ModelPackage packagesItem) {
if (this.packages == null) {
this.packages = new ArrayList();
}
this.packages.add(packagesItem);
return this;
}
/**
* Get packages
* @return packages
**/
@ApiModelProperty(value = "")
public List getPackages() {
return packages;
}
public void setPackages(List packages) {
this.packages = packages;
}
public Shipment parentShipmentNumber(Integer parentShipmentNumber) {
this.parentShipmentNumber = parentShipmentNumber;
return this;
}
/**
* Get parentShipmentNumber
* @return parentShipmentNumber
**/
@ApiModelProperty(value = "")
public Integer getParentShipmentNumber() {
return parentShipmentNumber;
}
public void setParentShipmentNumber(Integer parentShipmentNumber) {
this.parentShipmentNumber = parentShipmentNumber;
}
public Shipment pickStatus(PickStatusEnum pickStatus) {
this.pickStatus = pickStatus;
return this;
}
/**
* Get pickStatus
* @return pickStatus
**/
@ApiModelProperty(value = "")
public PickStatusEnum getPickStatus() {
return pickStatus;
}
public void setPickStatus(PickStatusEnum pickStatus) {
this.pickStatus = pickStatus;
}
public Shipment pickType(PickTypeEnum pickType) {
this.pickType = pickType;
return this;
}
/**
* Get pickType
* @return pickType
**/
@ApiModelProperty(value = "")
public PickTypeEnum getPickType() {
return pickType;
}
public void setPickType(PickTypeEnum pickType) {
this.pickType = pickType;
}
public Shipment pickWaveNumber(Integer pickWaveNumber) {
this.pickWaveNumber = pickWaveNumber;
return this;
}
/**
* Get pickWaveNumber
* @return pickWaveNumber
**/
@ApiModelProperty(value = "")
public Integer getPickWaveNumber() {
return pickWaveNumber;
}
public void setPickWaveNumber(Integer pickWaveNumber) {
this.pickWaveNumber = pickWaveNumber;
}
public Shipment pickupInfo(Map pickupInfo) {
this.pickupInfo = pickupInfo;
return this;
}
public Shipment putPickupInfoItem(String key, Object pickupInfoItem) {
if (this.pickupInfo == null) {
this.pickupInfo = new HashMap();
}
this.pickupInfo.put(key, pickupInfoItem);
return this;
}
/**
* Get pickupInfo
* @return pickupInfo
**/
@ApiModelProperty(value = "")
public Map getPickupInfo() {
return pickupInfo;
}
public void setPickupInfo(Map pickupInfo) {
this.pickupInfo = pickupInfo;
}
public Shipment readyForPickup(Boolean readyForPickup) {
this.readyForPickup = readyForPickup;
return this;
}
/**
* Get readyForPickup
* @return readyForPickup
**/
@ApiModelProperty(value = "")
public Boolean getReadyForPickup() {
return readyForPickup;
}
public void setReadyForPickup(Boolean readyForPickup) {
this.readyForPickup = readyForPickup;
}
public Shipment readyForPickupDate(OffsetDateTime readyForPickupDate) {
this.readyForPickupDate = readyForPickupDate;
return this;
}
/**
* Get readyForPickupDate
* @return readyForPickupDate
**/
@ApiModelProperty(value = "")
public OffsetDateTime getReadyForPickupDate() {
return readyForPickupDate;
}
public void setReadyForPickupDate(OffsetDateTime readyForPickupDate) {
this.readyForPickupDate = readyForPickupDate;
}
public Shipment readyToCapture(Boolean readyToCapture) {
this.readyToCapture = readyToCapture;
return this;
}
/**
* Get readyToCapture
* @return readyToCapture
**/
@ApiModelProperty(value = "")
public Boolean getReadyToCapture() {
return readyToCapture;
}
public void setReadyToCapture(Boolean readyToCapture) {
this.readyToCapture = readyToCapture;
}
public Shipment reassignedItems(List reassignedItems) {
this.reassignedItems = reassignedItems;
return this;
}
public Shipment addReassignedItemsItem(ReassignItem reassignedItemsItem) {
if (this.reassignedItems == null) {
this.reassignedItems = new ArrayList();
}
this.reassignedItems.add(reassignedItemsItem);
return this;
}
/**
* Get reassignedItems
* @return reassignedItems
**/
@ApiModelProperty(value = "")
public List getReassignedItems() {
return reassignedItems;
}
public void setReassignedItems(List reassignedItems) {
this.reassignedItems = reassignedItems;
}
public Shipment receivedDate(OffsetDateTime receivedDate) {
this.receivedDate = receivedDate;
return this;
}
/**
* Get receivedDate
* @return receivedDate
**/
@ApiModelProperty(value = "")
public OffsetDateTime getReceivedDate() {
return receivedDate;
}
public void setReceivedDate(OffsetDateTime receivedDate) {
this.receivedDate = receivedDate;
}
public Shipment rejectedItems(List rejectedItems) {
this.rejectedItems = rejectedItems;
return this;
}
public Shipment addRejectedItemsItem(RejectedItem rejectedItemsItem) {
if (this.rejectedItems == null) {
this.rejectedItems = new ArrayList();
}
this.rejectedItems.add(rejectedItemsItem);
return this;
}
/**
* Get rejectedItems
* @return rejectedItems
**/
@ApiModelProperty(value = "")
public List getRejectedItems() {
return rejectedItems;
}
public void setRejectedItems(List rejectedItems) {
this.rejectedItems = rejectedItems;
}
public Shipment sentCustomerAtStoreNotification(Boolean sentCustomerAtStoreNotification) {
this.sentCustomerAtStoreNotification = sentCustomerAtStoreNotification;
return this;
}
/**
* Get sentCustomerAtStoreNotification
* @return sentCustomerAtStoreNotification
**/
@ApiModelProperty(value = "")
public Boolean getSentCustomerAtStoreNotification() {
return sentCustomerAtStoreNotification;
}
public void setSentCustomerAtStoreNotification(Boolean sentCustomerAtStoreNotification) {
this.sentCustomerAtStoreNotification = sentCustomerAtStoreNotification;
}
public Shipment sentCustomerInTransitNotification(Boolean sentCustomerInTransitNotification) {
this.sentCustomerInTransitNotification = sentCustomerInTransitNotification;
return this;
}
/**
* Get sentCustomerInTransitNotification
* @return sentCustomerInTransitNotification
**/
@ApiModelProperty(value = "")
public Boolean getSentCustomerInTransitNotification() {
return sentCustomerInTransitNotification;
}
public void setSentCustomerInTransitNotification(Boolean sentCustomerInTransitNotification) {
this.sentCustomerInTransitNotification = sentCustomerInTransitNotification;
}
public Shipment shipmentAdjustment(BigDecimal shipmentAdjustment) {
this.shipmentAdjustment = shipmentAdjustment;
return this;
}
/**
* Get shipmentAdjustment
* @return shipmentAdjustment
**/
@ApiModelProperty(value = "")
public BigDecimal getShipmentAdjustment() {
return shipmentAdjustment;
}
public void setShipmentAdjustment(BigDecimal shipmentAdjustment) {
this.shipmentAdjustment = shipmentAdjustment;
}
public Shipment shipmentNumber(Integer shipmentNumber) {
this.shipmentNumber = shipmentNumber;
return this;
}
/**
* Get shipmentNumber
* @return shipmentNumber
**/
@ApiModelProperty(value = "")
public Integer getShipmentNumber() {
return shipmentNumber;
}
public void setShipmentNumber(Integer shipmentNumber) {
this.shipmentNumber = shipmentNumber;
}
public Shipment shipmentStatus(ShipmentStatusEnum shipmentStatus) {
this.shipmentStatus = shipmentStatus;
return this;
}
/**
* Get shipmentStatus
* @return shipmentStatus
**/
@ApiModelProperty(value = "")
public ShipmentStatusEnum getShipmentStatus() {
return shipmentStatus;
}
public void setShipmentStatus(ShipmentStatusEnum shipmentStatus) {
this.shipmentStatus = shipmentStatus;
}
public Shipment shipmentStatusReason(ShipmentStatusReason shipmentStatusReason) {
this.shipmentStatusReason = shipmentStatusReason;
return this;
}
/**
* Get shipmentStatusReason
* @return shipmentStatusReason
**/
@ApiModelProperty(value = "")
public ShipmentStatusReason getShipmentStatusReason() {
return shipmentStatusReason;
}
public void setShipmentStatusReason(ShipmentStatusReason shipmentStatusReason) {
this.shipmentStatusReason = shipmentStatusReason;
}
public Shipment shipmentType(String shipmentType) {
this.shipmentType = shipmentType;
return this;
}
/**
* Get shipmentType
* @return shipmentType
**/
@ApiModelProperty(value = "")
public String getShipmentType() {
return shipmentType;
}
public void setShipmentType(String shipmentType) {
this.shipmentType = shipmentType;
}
public Shipment shippingAdjustment(BigDecimal shippingAdjustment) {
this.shippingAdjustment = shippingAdjustment;
return this;
}
/**
* Get shippingAdjustment
* @return shippingAdjustment
**/
@ApiModelProperty(value = "")
public BigDecimal getShippingAdjustment() {
return shippingAdjustment;
}
public void setShippingAdjustment(BigDecimal shippingAdjustment) {
this.shippingAdjustment = shippingAdjustment;
}
public Shipment shippingMethodCode(String shippingMethodCode) {
this.shippingMethodCode = shippingMethodCode;
return this;
}
/**
* Get shippingMethodCode
* @return shippingMethodCode
**/
@ApiModelProperty(value = "")
public String getShippingMethodCode() {
return shippingMethodCode;
}
public void setShippingMethodCode(String shippingMethodCode) {
this.shippingMethodCode = shippingMethodCode;
}
public Shipment shippingMethodName(String shippingMethodName) {
this.shippingMethodName = shippingMethodName;
return this;
}
/**
* Get shippingMethodName
* @return shippingMethodName
**/
@ApiModelProperty(value = "")
public String getShippingMethodName() {
return shippingMethodName;
}
public void setShippingMethodName(String shippingMethodName) {
this.shippingMethodName = shippingMethodName;
}
public Shipment shippingSubtotal(BigDecimal shippingSubtotal) {
this.shippingSubtotal = shippingSubtotal;
return this;
}
/**
* Get shippingSubtotal
* @return shippingSubtotal
**/
@ApiModelProperty(value = "")
public BigDecimal getShippingSubtotal() {
return shippingSubtotal;
}
public void setShippingSubtotal(BigDecimal shippingSubtotal) {
this.shippingSubtotal = shippingSubtotal;
}
public Shipment shippingTaxAdjustment(BigDecimal shippingTaxAdjustment) {
this.shippingTaxAdjustment = shippingTaxAdjustment;
return this;
}
/**
* Get shippingTaxAdjustment
* @return shippingTaxAdjustment
**/
@ApiModelProperty(value = "")
public BigDecimal getShippingTaxAdjustment() {
return shippingTaxAdjustment;
}
public void setShippingTaxAdjustment(BigDecimal shippingTaxAdjustment) {
this.shippingTaxAdjustment = shippingTaxAdjustment;
}
public Shipment shippingTaxTotal(BigDecimal shippingTaxTotal) {
this.shippingTaxTotal = shippingTaxTotal;
return this;
}
/**
* Get shippingTaxTotal
* @return shippingTaxTotal
**/
@ApiModelProperty(value = "")
public BigDecimal getShippingTaxTotal() {
return shippingTaxTotal;
}
public void setShippingTaxTotal(BigDecimal shippingTaxTotal) {
this.shippingTaxTotal = shippingTaxTotal;
}
public Shipment shippingTotal(BigDecimal shippingTotal) {
this.shippingTotal = shippingTotal;
return this;
}
/**
* Get shippingTotal
* @return shippingTotal
**/
@ApiModelProperty(value = "")
public BigDecimal getShippingTotal() {
return shippingTotal;
}
public void setShippingTotal(BigDecimal shippingTotal) {
this.shippingTotal = shippingTotal;
}
public Shipment siteId(Integer siteId) {
this.siteId = siteId;
return this;
}
/**
* Get siteId
* @return siteId
**/
@ApiModelProperty(value = "")
public Integer getSiteId() {
return siteId;
}
public void setSiteId(Integer siteId) {
this.siteId = siteId;
}
public Shipment taxData(Object taxData) {
this.taxData = taxData;
return this;
}
/**
* Get taxData
* @return taxData
**/
@ApiModelProperty(value = "")
public Object getTaxData() {
return taxData;
}
public void setTaxData(Object taxData) {
this.taxData = taxData;
}
public Shipment tenantId(Integer tenantId) {
this.tenantId = tenantId;
return this;
}
/**
* Get tenantId
* @return tenantId
**/
@ApiModelProperty(value = "")
public Integer getTenantId() {
return tenantId;
}
public void setTenantId(Integer tenantId) {
this.tenantId = tenantId;
}
public Shipment total(BigDecimal total) {
this.total = total;
return this;
}
/**
* Get total
* @return total
**/
@ApiModelProperty(value = "")
public BigDecimal getTotal() {
return total;
}
public void setTotal(BigDecimal total) {
this.total = total;
}
public Shipment transferShipmentNumbers(List transferShipmentNumbers) {
this.transferShipmentNumbers = transferShipmentNumbers;
return this;
}
public Shipment addTransferShipmentNumbersItem(Integer transferShipmentNumbersItem) {
if (this.transferShipmentNumbers == null) {
this.transferShipmentNumbers = new ArrayList();
}
this.transferShipmentNumbers.add(transferShipmentNumbersItem);
return this;
}
/**
* Get transferShipmentNumbers
* @return transferShipmentNumbers
**/
@ApiModelProperty(value = "")
public List getTransferShipmentNumbers() {
return transferShipmentNumbers;
}
public void setTransferShipmentNumbers(List transferShipmentNumbers) {
this.transferShipmentNumbers = transferShipmentNumbers;
}
public Shipment transitTime(String transitTime) {
this.transitTime = transitTime;
return this;
}
/**
* Get transitTime
* @return transitTime
**/
@ApiModelProperty(value = "")
public String getTransitTime() {
return transitTime;
}
public void setTransitTime(String transitTime) {
this.transitTime = transitTime;
}
public Shipment userId(String userId) {
this.userId = userId;
return this;
}
/**
* Get userId
* @return userId
**/
@ApiModelProperty(value = "")
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public Shipment workflowProcessContainerId(String workflowProcessContainerId) {
this.workflowProcessContainerId = workflowProcessContainerId;
return this;
}
/**
* Get workflowProcessContainerId
* @return workflowProcessContainerId
**/
@ApiModelProperty(value = "")
public String getWorkflowProcessContainerId() {
return workflowProcessContainerId;
}
public void setWorkflowProcessContainerId(String workflowProcessContainerId) {
this.workflowProcessContainerId = workflowProcessContainerId;
}
public Shipment workflowProcessId(String workflowProcessId) {
this.workflowProcessId = workflowProcessId;
return this;
}
/**
* Get workflowProcessId
* @return workflowProcessId
**/
@ApiModelProperty(value = "")
public String getWorkflowProcessId() {
return workflowProcessId;
}
public void setWorkflowProcessId(String workflowProcessId) {
this.workflowProcessId = workflowProcessId;
}
public Shipment workflowState(WorkflowState workflowState) {
this.workflowState = workflowState;
return this;
}
/**
* Get workflowState
* @return workflowState
**/
@ApiModelProperty(value = "")
public WorkflowState getWorkflowState() {
return workflowState;
}
public void setWorkflowState(WorkflowState workflowState) {
this.workflowState = workflowState;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Shipment shipment = (Shipment) o;
return Objects.equals(this.acceptedDate, shipment.acceptedDate) &&
Objects.equals(this.assignedLocationCode, shipment.assignedLocationCode) &&
Objects.equals(this.attributes, shipment.attributes) &&
Objects.equals(this.auditInfo, shipment.auditInfo) &&
Objects.equals(this.canceledItems, shipment.canceledItems) &&
Objects.equals(this.changeMessages, shipment.changeMessages) &&
Objects.equals(this.childShipmentNumbers, shipment.childShipmentNumbers) &&
Objects.equals(this.currencyCode, shipment.currencyCode) &&
Objects.equals(this.customer, shipment.customer) &&
Objects.equals(this.customerAccountId, shipment.customerAccountId) &&
Objects.equals(this.customerAddressId, shipment.customerAddressId) &&
Objects.equals(this.customerTaxId, shipment.customerTaxId) &&
Objects.equals(this.data, shipment.data) &&
Objects.equals(this.destination, shipment.destination) &&
Objects.equals(this.dutyAdjustment, shipment.dutyAdjustment) &&
Objects.equals(this.dutyTotal, shipment.dutyTotal) &&
Objects.equals(this.email, shipment.email) &&
Objects.equals(this.externalOrderId, shipment.externalOrderId) &&
Objects.equals(this.fulfillmentDate, shipment.fulfillmentDate) &&
Objects.equals(this.fulfillmentLocationCode, shipment.fulfillmentLocationCode) &&
Objects.equals(this.fulfillmentStatus, shipment.fulfillmentStatus) &&
Objects.equals(this.handlingAdjustment, shipment.handlingAdjustment) &&
Objects.equals(this.handlingSubtotal, shipment.handlingSubtotal) &&
Objects.equals(this.handlingTaxAdjustment, shipment.handlingTaxAdjustment) &&
Objects.equals(this.handlingTaxTotal, shipment.handlingTaxTotal) &&
Objects.equals(this.handlingTotal, shipment.handlingTotal) &&
Objects.equals(this.isExpress, shipment.isExpress) &&
Objects.equals(this.isOptInForSms, shipment.isOptInForSms) &&
Objects.equals(this.items, shipment.items) &&
Objects.equals(this.lineItemSubtotal, shipment.lineItemSubtotal) &&
Objects.equals(this.lineItemTaxAdjustment, shipment.lineItemTaxAdjustment) &&
Objects.equals(this.lineItemTaxTotal, shipment.lineItemTaxTotal) &&
Objects.equals(this.lineItemTotal, shipment.lineItemTotal) &&
Objects.equals(this.orderId, shipment.orderId) &&
Objects.equals(this.orderNumber, shipment.orderNumber) &&
Objects.equals(this.orderSubmitDate, shipment.orderSubmitDate) &&
Objects.equals(this.originalShipmentNumber, shipment.originalShipmentNumber) &&
Objects.equals(this.packages, shipment.packages) &&
Objects.equals(this.parentShipmentNumber, shipment.parentShipmentNumber) &&
Objects.equals(this.pickStatus, shipment.pickStatus) &&
Objects.equals(this.pickType, shipment.pickType) &&
Objects.equals(this.pickWaveNumber, shipment.pickWaveNumber) &&
Objects.equals(this.pickupInfo, shipment.pickupInfo) &&
Objects.equals(this.readyForPickup, shipment.readyForPickup) &&
Objects.equals(this.readyForPickupDate, shipment.readyForPickupDate) &&
Objects.equals(this.readyToCapture, shipment.readyToCapture) &&
Objects.equals(this.reassignedItems, shipment.reassignedItems) &&
Objects.equals(this.receivedDate, shipment.receivedDate) &&
Objects.equals(this.rejectedItems, shipment.rejectedItems) &&
Objects.equals(this.sentCustomerAtStoreNotification, shipment.sentCustomerAtStoreNotification) &&
Objects.equals(this.sentCustomerInTransitNotification, shipment.sentCustomerInTransitNotification) &&
Objects.equals(this.shipmentAdjustment, shipment.shipmentAdjustment) &&
Objects.equals(this.shipmentNumber, shipment.shipmentNumber) &&
Objects.equals(this.shipmentStatus, shipment.shipmentStatus) &&
Objects.equals(this.shipmentStatusReason, shipment.shipmentStatusReason) &&
Objects.equals(this.shipmentType, shipment.shipmentType) &&
Objects.equals(this.shippingAdjustment, shipment.shippingAdjustment) &&
Objects.equals(this.shippingMethodCode, shipment.shippingMethodCode) &&
Objects.equals(this.shippingMethodName, shipment.shippingMethodName) &&
Objects.equals(this.shippingSubtotal, shipment.shippingSubtotal) &&
Objects.equals(this.shippingTaxAdjustment, shipment.shippingTaxAdjustment) &&
Objects.equals(this.shippingTaxTotal, shipment.shippingTaxTotal) &&
Objects.equals(this.shippingTotal, shipment.shippingTotal) &&
Objects.equals(this.siteId, shipment.siteId) &&
Objects.equals(this.taxData, shipment.taxData) &&
Objects.equals(this.tenantId, shipment.tenantId) &&
Objects.equals(this.total, shipment.total) &&
Objects.equals(this.transferShipmentNumbers, shipment.transferShipmentNumbers) &&
Objects.equals(this.transitTime, shipment.transitTime) &&
Objects.equals(this.userId, shipment.userId) &&
Objects.equals(this.workflowProcessContainerId, shipment.workflowProcessContainerId) &&
Objects.equals(this.workflowProcessId, shipment.workflowProcessId) &&
Objects.equals(this.workflowState, shipment.workflowState);
}
@Override
public int hashCode() {
return Objects.hash(acceptedDate, assignedLocationCode, attributes, auditInfo, canceledItems, changeMessages, childShipmentNumbers, currencyCode, customer, customerAccountId, customerAddressId, customerTaxId, data, destination, dutyAdjustment, dutyTotal, email, externalOrderId, fulfillmentDate, fulfillmentLocationCode, fulfillmentStatus, handlingAdjustment, handlingSubtotal, handlingTaxAdjustment, handlingTaxTotal, handlingTotal, isExpress, isOptInForSms, items, lineItemSubtotal, lineItemTaxAdjustment, lineItemTaxTotal, lineItemTotal, orderId, orderNumber, orderSubmitDate, originalShipmentNumber, packages, parentShipmentNumber, pickStatus, pickType, pickWaveNumber, pickupInfo, readyForPickup, readyForPickupDate, readyToCapture, reassignedItems, receivedDate, rejectedItems, sentCustomerAtStoreNotification, sentCustomerInTransitNotification, shipmentAdjustment, shipmentNumber, shipmentStatus, shipmentStatusReason, shipmentType, shippingAdjustment, shippingMethodCode, shippingMethodName, shippingSubtotal, shippingTaxAdjustment, shippingTaxTotal, shippingTotal, siteId, taxData, tenantId, total, transferShipmentNumbers, transitTime, userId, workflowProcessContainerId, workflowProcessId, workflowState);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Shipment {\n");
sb.append(" acceptedDate: ").append(toIndentedString(acceptedDate)).append("\n");
sb.append(" assignedLocationCode: ").append(toIndentedString(assignedLocationCode)).append("\n");
sb.append(" attributes: ").append(toIndentedString(attributes)).append("\n");
sb.append(" auditInfo: ").append(toIndentedString(auditInfo)).append("\n");
sb.append(" canceledItems: ").append(toIndentedString(canceledItems)).append("\n");
sb.append(" changeMessages: ").append(toIndentedString(changeMessages)).append("\n");
sb.append(" childShipmentNumbers: ").append(toIndentedString(childShipmentNumbers)).append("\n");
sb.append(" currencyCode: ").append(toIndentedString(currencyCode)).append("\n");
sb.append(" customer: ").append(toIndentedString(customer)).append("\n");
sb.append(" customerAccountId: ").append(toIndentedString(customerAccountId)).append("\n");
sb.append(" customerAddressId: ").append(toIndentedString(customerAddressId)).append("\n");
sb.append(" customerTaxId: ").append(toIndentedString(customerTaxId)).append("\n");
sb.append(" data: ").append(toIndentedString(data)).append("\n");
sb.append(" destination: ").append(toIndentedString(destination)).append("\n");
sb.append(" dutyAdjustment: ").append(toIndentedString(dutyAdjustment)).append("\n");
sb.append(" dutyTotal: ").append(toIndentedString(dutyTotal)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" externalOrderId: ").append(toIndentedString(externalOrderId)).append("\n");
sb.append(" fulfillmentDate: ").append(toIndentedString(fulfillmentDate)).append("\n");
sb.append(" fulfillmentLocationCode: ").append(toIndentedString(fulfillmentLocationCode)).append("\n");
sb.append(" fulfillmentStatus: ").append(toIndentedString(fulfillmentStatus)).append("\n");
sb.append(" handlingAdjustment: ").append(toIndentedString(handlingAdjustment)).append("\n");
sb.append(" handlingSubtotal: ").append(toIndentedString(handlingSubtotal)).append("\n");
sb.append(" handlingTaxAdjustment: ").append(toIndentedString(handlingTaxAdjustment)).append("\n");
sb.append(" handlingTaxTotal: ").append(toIndentedString(handlingTaxTotal)).append("\n");
sb.append(" handlingTotal: ").append(toIndentedString(handlingTotal)).append("\n");
sb.append(" isExpress: ").append(toIndentedString(isExpress)).append("\n");
sb.append(" isOptInForSms: ").append(toIndentedString(isOptInForSms)).append("\n");
sb.append(" items: ").append(toIndentedString(items)).append("\n");
sb.append(" lineItemSubtotal: ").append(toIndentedString(lineItemSubtotal)).append("\n");
sb.append(" lineItemTaxAdjustment: ").append(toIndentedString(lineItemTaxAdjustment)).append("\n");
sb.append(" lineItemTaxTotal: ").append(toIndentedString(lineItemTaxTotal)).append("\n");
sb.append(" lineItemTotal: ").append(toIndentedString(lineItemTotal)).append("\n");
sb.append(" orderId: ").append(toIndentedString(orderId)).append("\n");
sb.append(" orderNumber: ").append(toIndentedString(orderNumber)).append("\n");
sb.append(" orderSubmitDate: ").append(toIndentedString(orderSubmitDate)).append("\n");
sb.append(" originalShipmentNumber: ").append(toIndentedString(originalShipmentNumber)).append("\n");
sb.append(" packages: ").append(toIndentedString(packages)).append("\n");
sb.append(" parentShipmentNumber: ").append(toIndentedString(parentShipmentNumber)).append("\n");
sb.append(" pickStatus: ").append(toIndentedString(pickStatus)).append("\n");
sb.append(" pickType: ").append(toIndentedString(pickType)).append("\n");
sb.append(" pickWaveNumber: ").append(toIndentedString(pickWaveNumber)).append("\n");
sb.append(" pickupInfo: ").append(toIndentedString(pickupInfo)).append("\n");
sb.append(" readyForPickup: ").append(toIndentedString(readyForPickup)).append("\n");
sb.append(" readyForPickupDate: ").append(toIndentedString(readyForPickupDate)).append("\n");
sb.append(" readyToCapture: ").append(toIndentedString(readyToCapture)).append("\n");
sb.append(" reassignedItems: ").append(toIndentedString(reassignedItems)).append("\n");
sb.append(" receivedDate: ").append(toIndentedString(receivedDate)).append("\n");
sb.append(" rejectedItems: ").append(toIndentedString(rejectedItems)).append("\n");
sb.append(" sentCustomerAtStoreNotification: ").append(toIndentedString(sentCustomerAtStoreNotification)).append("\n");
sb.append(" sentCustomerInTransitNotification: ").append(toIndentedString(sentCustomerInTransitNotification)).append("\n");
sb.append(" shipmentAdjustment: ").append(toIndentedString(shipmentAdjustment)).append("\n");
sb.append(" shipmentNumber: ").append(toIndentedString(shipmentNumber)).append("\n");
sb.append(" shipmentStatus: ").append(toIndentedString(shipmentStatus)).append("\n");
sb.append(" shipmentStatusReason: ").append(toIndentedString(shipmentStatusReason)).append("\n");
sb.append(" shipmentType: ").append(toIndentedString(shipmentType)).append("\n");
sb.append(" shippingAdjustment: ").append(toIndentedString(shippingAdjustment)).append("\n");
sb.append(" shippingMethodCode: ").append(toIndentedString(shippingMethodCode)).append("\n");
sb.append(" shippingMethodName: ").append(toIndentedString(shippingMethodName)).append("\n");
sb.append(" shippingSubtotal: ").append(toIndentedString(shippingSubtotal)).append("\n");
sb.append(" shippingTaxAdjustment: ").append(toIndentedString(shippingTaxAdjustment)).append("\n");
sb.append(" shippingTaxTotal: ").append(toIndentedString(shippingTaxTotal)).append("\n");
sb.append(" shippingTotal: ").append(toIndentedString(shippingTotal)).append("\n");
sb.append(" siteId: ").append(toIndentedString(siteId)).append("\n");
sb.append(" taxData: ").append(toIndentedString(taxData)).append("\n");
sb.append(" tenantId: ").append(toIndentedString(tenantId)).append("\n");
sb.append(" total: ").append(toIndentedString(total)).append("\n");
sb.append(" transferShipmentNumbers: ").append(toIndentedString(transferShipmentNumbers)).append("\n");
sb.append(" transitTime: ").append(toIndentedString(transitTime)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" workflowProcessContainerId: ").append(toIndentedString(workflowProcessContainerId)).append("\n");
sb.append(" workflowProcessId: ").append(toIndentedString(workflowProcessId)).append("\n");
sb.append(" workflowState: ").append(toIndentedString(workflowState)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy