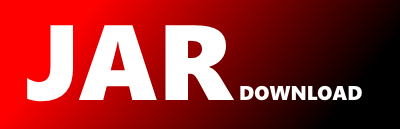
com.king.platform.net.http.BuiltClientRequest Maven / Gradle / Ivy
// Copyright (C) king.com Ltd 2015
// https://github.com/king/king-http-client
// Author: Magnus Gustafsson
// License: Apache 2.0, https://raw.github.com/king/king-http-client/LICENSE-APACHE
package com.king.platform.net.http;
import com.king.platform.net.http.netty.eventbus.ExternalEventTrigger;
import java.util.concurrent.Future;
public interface BuiltClientRequest {
/**
* Execute the built request, consume the returned data as String
*
* @return a future with the result of the request
*/
Future> execute();
/**
* Execute the built request, consume the returned data as String
*
* @param httpCallback the callback object, executed on the HttpCallbackExecutor
* @return a future with the result of the request
*/
Future> execute(HttpCallback httpCallback);
/**
* Execute the built request, consume the returned data as String
*
* @param httpCallback the callback object, executed on the HttpCallbackExecutor
* @param nioCallback the NioCallback object, executed on the io thread for this request.
* @return a future with the result of the request
*/
Future> execute(HttpCallback httpCallback, NioCallback nioCallback);
/**
* Execute the built request, consume the returned data as the type defined by responseBodyConsumer
*
* @param httpCallback the callback object, executed on the HttpCallbackExecutor
* @param responseBodyConsumer the consumer of the read http body
* @param the type defined by the responseBodyConsumer
* @return a future with the result of the request
*/
Future> execute(HttpCallback httpCallback, ResponseBodyConsumer responseBodyConsumer);
/**
* Execute the built request, consume the returned data as the type defined by the responseBodyConsumer parameter
*
* @param responseBodyConsumer the consumer of the response body
* @param the type of the response body
* @return a future with the result of the request
*/
Future> execute(ResponseBodyConsumer responseBodyConsumer);
/**
* Execute the built request, consume the returned data as String
*
* @param nioCallback the NioCallback object, executed on the io thread for this request.
* @return a future with the result of the request
*/
Future> execute(NioCallback nioCallback);
/**
* Execute the built request, consume the returned data as the type defined by responseBodyConsumer
*
* @param httpCallback the callback object, executed on the HttpCallbackExecutor
* @param responseBodyConsumer the consumer of the read http body
* @param nioCallback the NioCallback object, executed on the io thread for this request.
* @param the type defined by the responseBodyConsumer
* @return a future with the result of the request
*/
Future> execute(HttpCallback httpCallback, ResponseBodyConsumer responseBodyConsumer, NioCallback nioCallback);
/**
* Execute the built request, consume the returned data as the type defined by responseBodyConsumer
* @param httpCallback the callback object, executed on the HttpCallbackExecutor
* @param responseBodyConsumer the consumer of the read http body
* @param nioCallback the NioCallback object, executed on the io thread for this request.
* @param externalEventTrigger the trigger for external events to control the client
* @param the type defined by the responseBodyConsumer
* @return a future with the result of the request
*/
Future> execute(HttpCallback httpCallback, ResponseBodyConsumer responseBodyConsumer, NioCallback nioCallback, ExternalEventTrigger externalEventTrigger);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy