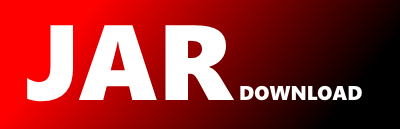
com.king.platform.net.http.WebSocketConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of king-http-client Show documentation
Show all versions of king-http-client Show documentation
A asyncronous http client built ontop of netty.
package com.king.platform.net.http;
import java.util.concurrent.CompletableFuture;
public interface WebSocketConnection {
/**
* Return the current headers for this connection
* @return headers
*/
Headers headers();
/**
* The current negotiated sub protocols the server has decided on.
* @return the protocol
*/
String getNegotiatedSubProtocol();
/**
* Is the current web socket connected to the server
* @return true if connected
*/
boolean isConnected();
/**
* Send an text frame to the server
* @param text the text
* @return the resulting future
*/
CompletableFuture sendTextFrame(String text);
/**
* Send an close frame to the server. This is to inform the server about wanting to close the connection.
* @return the resulting future
* @param statusCode the status code
* @param reason the reason to close
*/
CompletableFuture sendCloseFrame(int statusCode, String reason);
/**
* Send an close frame to the server. This is to inform the server about wanting to close the connection.
* StatusCode 1000 (Normal close) will be used.
* @return the resulting future
*
*/
CompletableFuture sendCloseFrame();
/**
* Send an binary frame to the server
* @param payload the bytes
* @return the resulting future
*/
CompletableFuture sendBinaryFrame(byte[] payload);
/**
* Send a ping frame to the server
*
* @return the resulting future
*/
CompletableFuture sendPingFrame();
/**
* Send a ping frame with a payload to the server
*
* @param payload the payload
* @return the resulting future
*/
CompletableFuture sendPingFrame(byte[] payload);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy