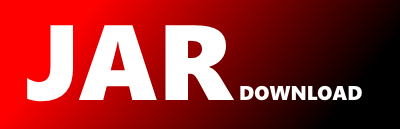
com.kingsoft.services.KWSWebServiceClient Maven / Gradle / Ivy
package com.kingsoft.services;
import java.net.URI;
import java.net.URISyntaxException;
import com.kingsoft.services.http.IKWSHttpClient;
import com.kingsoft.services.http.KWSHttpClient;
public class KWSWebServiceClient {
protected volatile URI endpoint;
protected ClientOptions clientConfiguration;
protected IKWSHttpClient client;
protected KWSWebServiceClient(String endpoint, ClientOptions clientConfiguration, IKWSHttpClient client) {
this.clientConfiguration = clientConfiguration;
this.client = client;
setEndpoint(endpoint);
}
public void shutdown() {
client.shutdown();
}
private void setEndpoint(String endpoint) throws IllegalArgumentException {
URI uri = toURI(endpoint);
synchronized (this) {
this.endpoint = uri;
}
}
public URI getEndPoint(){
return this.endpoint;
}
private URI toURI(String endpoint) throws IllegalArgumentException {
/*
* If the endpoint doesn't explicitly specify a protocol to use, then
* we'll defer to the default protocol specified in the client
* configuration.
*/
String[] endpoints = endpoint.split(",");
if (endpoints.length > 0) {
java.util.Random r = new java.util.Random();
endpoint = endpoints[r.nextInt(endpoints.length)];
if (endpoint.contains("://") == false) {
endpoint = clientConfiguration.getProtocol().toString() + "://" + endpoint.trim();
}
}
try {
return new URI(endpoint);
} catch (URISyntaxException e) {
throw new IllegalArgumentException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy