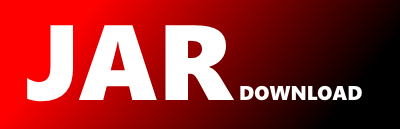
com.kingsoft.services.RequestMessage Maven / Gradle / Ivy
package com.kingsoft.services;
import java.io.InputStream;
import java.net.URI;
import java.util.HashMap;
import java.util.Map;
import org.apache.http.annotation.NotThreadSafe;
import com.kingsoft.services.auth.KWSCredentials;
import com.kingsoft.services.http.HttpMethodName;
@NotThreadSafe
public class RequestMessage {
/** The resource path being requested */
private String resourcePath;
/** Map of the headers included in this request */
private Map headers = new HashMap();
/** The service endpoint to which this request should be sent */
private URI endpoint;
private long requestId;
private KWSCredentials credentials;
private Class> responseMessageType;
/** The name of the service to which this request is being sent */
private String serviceName;
/** The HTTP method to use when sending this request. */
private HttpMethodName httpMethod = HttpMethodName.POST;
private InputStream content;
private long startTime;
public RequestMessage() {
startTime = System.currentTimeMillis();
}
public Class> getResponseMessageType() {
return responseMessageType;
}
public void setResponseMessageType(Class> responseMessageType) {
this.responseMessageType = responseMessageType;
}
public KWSCredentials getCredentials() {
return credentials;
}
public void setCredentials(KWSCredentials credentials) {
this.credentials = credentials;
}
public void setServiceName(String serviceName) {
this.serviceName = serviceName;
}
public void addHeader(String name, String value) {
headers.put(name, value);
}
public Map getHeaders() {
return headers;
}
public void setHeaders(Map headers) {
this.headers.clear();
this.headers.putAll(headers);
}
public String getResourcePath() {
return resourcePath;
}
public void setResourcePath(String resourcePath) {
this.resourcePath = resourcePath;
}
public HttpMethodName getHttpMethod() {
return httpMethod;
}
public void setHttpMethod(HttpMethodName httpMethod) {
this.httpMethod = httpMethod;
}
public URI getEndpoint() {
return endpoint;
}
public void setEndpoint(URI endpoint) {
this.endpoint = endpoint;
}
public String getServiceName() {
return serviceName;
}
public InputStream getContent() {
return content;
}
public void setContent(InputStream content) {
this.content = content;
}
public long getRequestId() {
return requestId;
}
public void setRequestId(long requestId) {
this.requestId = requestId;
}
public long getStartTime() {
return startTime;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy