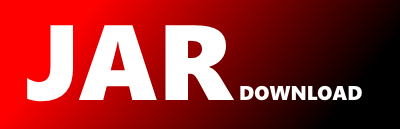
com.kintone.client.FileClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kintone-java-client Show documentation
Show all versions of kintone-java-client Show documentation
API client library for Kintone REST APIs on Java.
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client;
import com.kintone.client.api.common.DownloadFileRequest;
import com.kintone.client.api.common.DownloadFileResponseBody;
import com.kintone.client.api.common.UploadFileRequest;
import com.kintone.client.api.common.UploadFileResponseBody;
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.List;
/**
* A client that operates file APIs.
*/
public class FileClient {
private final InternalClient client;
private final List handlers;
/**
* Downloads files from an attachment field in an app.
*
* @param fileKey the value that is set on the Attachment field in the response data returned when
* using the Get Record API
* @return the content data stream
* @throws IOException if an I/O error occurs
*/
public InputStream downloadFile(String fileKey) throws IOException {
DownloadFileRequest req = new DownloadFileRequest();
req.setFileKey(fileKey);
return downloadFile(req).getContent();
}
/**
* Downloads files from an attachment field in an app.
*
* @param request the request parameters. See {@link DownloadFileRequest}
* @return the response data. See {@link DownloadFileResponseBody}
*/
public DownloadFileResponseBody downloadFile(DownloadFileRequest request) {
return client.download(request, handlers);
}
/**
* Uploads a file to Kintone.
*
* @param path the file to upload
* @param contentType the Content Type of the file.
* @return the fileKey of the uploaded file
* @throws IOException if an I/O error occurs
*/
public String uploadFile(Path path, String contentType) throws IOException {
UploadFileRequest req = new UploadFileRequest();
req.setContentType(contentType);
req.setFilename(path.getFileName().toString());
try (InputStream content = Files.newInputStream(path)) {
req.setContent(content);
return uploadFile(req).getFileKey();
}
}
/**
* Uploads a file to Kintone.
*
* @param request the request parameters. See {@link UploadFileRequest}
* @return the response data. See {@link UploadFileResponseBody}
*/
public UploadFileResponseBody uploadFile(UploadFileRequest request) {
return client.upload(request.getFilename(), request.getContentType(), request.getContent(), handlers).getBody();
}
@java.beans.ConstructorProperties({"client", "handlers"})
@java.lang.SuppressWarnings("all")
FileClient(final InternalClient client, final List handlers) {
this.client = client;
this.handlers = handlers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy