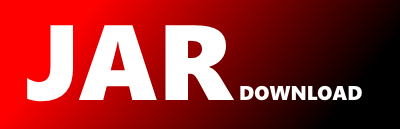
com.kintone.client.SchemaClient Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client;
import com.kintone.client.api.schema.GetApiListResponseBody;
import com.kintone.client.api.schema.GetApiSchemaResponseBody;
import java.util.Arrays;
import java.util.List;
/**
* A client that operates schema APIs.
*/
public class SchemaClient {
private final InternalClient client;
private final List handlers;
/**
* Gets the list of App/Record/Space APIs available to use on kintone.
*
* @return the response data. See {@link GetApiListResponseBody}
*/
public GetApiListResponseBody getApiList() {
return client.call(KintoneApi.GET_API_LIST, null, handlers);
}
/**
* Gets the API schema info of a kintone API.
*
* @param api the target api
* @return the response data. See {@link GetApiSchemaResponseBody}
*/
public GetApiSchemaResponseBody getApiSchema(KintoneApi api) {
if (api == KintoneApi.GET_API_LIST) {
return null;
}
String path = "/k/v1/" + toApiPath(api);
return client.call(KintoneHttpMethod.GET, path, null, GetApiSchemaResponseBody.class, handlers);
}
/**
* Gets the API schema info of a kintone API.
*
* @param link the path of target api
* @return the response data. See {@link GetApiSchemaResponseBody}
*/
public GetApiSchemaResponseBody getApiSchema(String link) {
boolean valid = Arrays.stream(KintoneApi.values()).anyMatch(api -> api != KintoneApi.GET_API_LIST && toApiPath(api).equals(link));
if (!valid) {
return null;
}
String path = "/k/v1/" + link;
return client.call(KintoneHttpMethod.GET, path, null, GetApiSchemaResponseBody.class, handlers);
}
private String toApiPath(KintoneApi api) {
return "apis/" + api.getEndpoint() + "/" + api.getMethod().toString().toLowerCase() + ".json";
}
@java.beans.ConstructorProperties({"client", "handlers"})
@java.lang.SuppressWarnings("all")
SchemaClient(final InternalClient client, final List handlers) {
this.client = client;
this.handlers = handlers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy