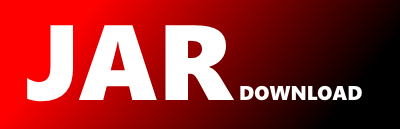
com.kintone.client.api.app.GetAppResponseBody Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.api.app;
import com.kintone.client.api.KintoneResponseBody;
import com.kintone.client.model.User;
import java.time.ZonedDateTime;
/**
* A response object for Get App API.
*/
public final class GetAppResponseBody implements KintoneResponseBody {
/**
* The App ID.
*/
private final long appId;
/**
* The App Code of the App.
*/
private final String code;
/**
* The name of the App.
*/
private final String name;
/**
* The description of the App.
*/
private final String description;
/**
* If the App was created inside a Space, it will return the Space ID.
*/
private final Long spaceId;
/**
* If the App was created inside a Space, it will return the Thread ID of the Thread of the space
* it belongs to.
*/
private final Long threadId;
/**
* The date of when the App was created.
*/
private final ZonedDateTime createdAt;
/**
* The information of the user who created the App.
*/
private final User creator;
/**
* The date of when the App was last modified.
*/
private final ZonedDateTime modifiedAt;
/**
* The information of the user who last updated the App.
*/
private final User modifier;
@java.beans.ConstructorProperties({"appId", "code", "name", "description", "spaceId", "threadId", "createdAt", "creator", "modifiedAt", "modifier"})
@java.lang.SuppressWarnings("all")
public GetAppResponseBody(final long appId, final String code, final String name, final String description, final Long spaceId, final Long threadId, final ZonedDateTime createdAt, final User creator, final ZonedDateTime modifiedAt, final User modifier) {
this.appId = appId;
this.code = code;
this.name = name;
this.description = description;
this.spaceId = spaceId;
this.threadId = threadId;
this.createdAt = createdAt;
this.creator = creator;
this.modifiedAt = modifiedAt;
this.modifier = modifier;
}
/**
* The App ID.
*/
@java.lang.SuppressWarnings("all")
public long getAppId() {
return this.appId;
}
/**
* The App Code of the App.
*/
@java.lang.SuppressWarnings("all")
public String getCode() {
return this.code;
}
/**
* The name of the App.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* The description of the App.
*/
@java.lang.SuppressWarnings("all")
public String getDescription() {
return this.description;
}
/**
* If the App was created inside a Space, it will return the Space ID.
*/
@java.lang.SuppressWarnings("all")
public Long getSpaceId() {
return this.spaceId;
}
/**
* If the App was created inside a Space, it will return the Thread ID of the Thread of the space
* it belongs to.
*/
@java.lang.SuppressWarnings("all")
public Long getThreadId() {
return this.threadId;
}
/**
* The date of when the App was created.
*/
@java.lang.SuppressWarnings("all")
public ZonedDateTime getCreatedAt() {
return this.createdAt;
}
/**
* The information of the user who created the App.
*/
@java.lang.SuppressWarnings("all")
public User getCreator() {
return this.creator;
}
/**
* The date of when the App was last modified.
*/
@java.lang.SuppressWarnings("all")
public ZonedDateTime getModifiedAt() {
return this.modifiedAt;
}
/**
* The information of the user who last updated the App.
*/
@java.lang.SuppressWarnings("all")
public User getModifier() {
return this.modifier;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof GetAppResponseBody)) return false;
final GetAppResponseBody other = (GetAppResponseBody) o;
if (this.getAppId() != other.getAppId()) return false;
final java.lang.Object this$spaceId = this.getSpaceId();
final java.lang.Object other$spaceId = other.getSpaceId();
if (this$spaceId == null ? other$spaceId != null : !this$spaceId.equals(other$spaceId)) return false;
final java.lang.Object this$threadId = this.getThreadId();
final java.lang.Object other$threadId = other.getThreadId();
if (this$threadId == null ? other$threadId != null : !this$threadId.equals(other$threadId)) return false;
final java.lang.Object this$code = this.getCode();
final java.lang.Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$createdAt = this.getCreatedAt();
final java.lang.Object other$createdAt = other.getCreatedAt();
if (this$createdAt == null ? other$createdAt != null : !this$createdAt.equals(other$createdAt)) return false;
final java.lang.Object this$creator = this.getCreator();
final java.lang.Object other$creator = other.getCreator();
if (this$creator == null ? other$creator != null : !this$creator.equals(other$creator)) return false;
final java.lang.Object this$modifiedAt = this.getModifiedAt();
final java.lang.Object other$modifiedAt = other.getModifiedAt();
if (this$modifiedAt == null ? other$modifiedAt != null : !this$modifiedAt.equals(other$modifiedAt)) return false;
final java.lang.Object this$modifier = this.getModifier();
final java.lang.Object other$modifier = other.getModifier();
if (this$modifier == null ? other$modifier != null : !this$modifier.equals(other$modifier)) return false;
return true;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final long $appId = this.getAppId();
result = result * PRIME + (int) ($appId >>> 32 ^ $appId);
final java.lang.Object $spaceId = this.getSpaceId();
result = result * PRIME + ($spaceId == null ? 43 : $spaceId.hashCode());
final java.lang.Object $threadId = this.getThreadId();
result = result * PRIME + ($threadId == null ? 43 : $threadId.hashCode());
final java.lang.Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $createdAt = this.getCreatedAt();
result = result * PRIME + ($createdAt == null ? 43 : $createdAt.hashCode());
final java.lang.Object $creator = this.getCreator();
result = result * PRIME + ($creator == null ? 43 : $creator.hashCode());
final java.lang.Object $modifiedAt = this.getModifiedAt();
result = result * PRIME + ($modifiedAt == null ? 43 : $modifiedAt.hashCode());
final java.lang.Object $modifier = this.getModifier();
result = result * PRIME + ($modifier == null ? 43 : $modifier.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "GetAppResponseBody(appId=" + this.getAppId() + ", code=" + this.getCode() + ", name=" + this.getName() + ", description=" + this.getDescription() + ", spaceId=" + this.getSpaceId() + ", threadId=" + this.getThreadId() + ", createdAt=" + this.getCreatedAt() + ", creator=" + this.getCreator() + ", modifiedAt=" + this.getModifiedAt() + ", modifier=" + this.getModifier() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy