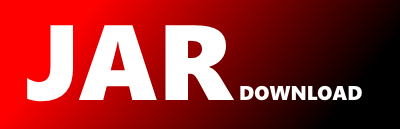
com.kintone.client.api.app.GetAppsRequest Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.api.app;
import com.kintone.client.api.KintoneRequest;
import java.util.List;
/**
* A request object for Get Apps API.
*/
public class GetAppsRequest implements KintoneRequest {
/**
* The App IDs.
*/
private List ids;
/**
* The App Code.
*/
private List codes;
/**
* The App Name.
*/
private String name;
/**
* The Space ID of where the App resides in.
*/
private List spaceIds;
/**
* The number of Apps to retrieve.
*/
private Long limit;
/**
* The number of retrievals that will be skipped.
*/
private Long offset;
@java.lang.SuppressWarnings("all")
public GetAppsRequest() {
}
/**
* The App IDs.
*/
@java.lang.SuppressWarnings("all")
public List getIds() {
return this.ids;
}
/**
* The App Code.
*/
@java.lang.SuppressWarnings("all")
public List getCodes() {
return this.codes;
}
/**
* The App Name.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* The Space ID of where the App resides in.
*/
@java.lang.SuppressWarnings("all")
public List getSpaceIds() {
return this.spaceIds;
}
/**
* The number of Apps to retrieve.
*/
@java.lang.SuppressWarnings("all")
public Long getLimit() {
return this.limit;
}
/**
* The number of retrievals that will be skipped.
*/
@java.lang.SuppressWarnings("all")
public Long getOffset() {
return this.offset;
}
/**
* The App IDs.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetAppsRequest setIds(final List ids) {
this.ids = ids;
return this;
}
/**
* The App Code.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetAppsRequest setCodes(final List codes) {
this.codes = codes;
return this;
}
/**
* The App Name.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetAppsRequest setName(final String name) {
this.name = name;
return this;
}
/**
* The Space ID of where the App resides in.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetAppsRequest setSpaceIds(final List spaceIds) {
this.spaceIds = spaceIds;
return this;
}
/**
* The number of Apps to retrieve.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetAppsRequest setLimit(final Long limit) {
this.limit = limit;
return this;
}
/**
* The number of retrievals that will be skipped.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetAppsRequest setOffset(final Long offset) {
this.offset = offset;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof GetAppsRequest)) return false;
final GetAppsRequest other = (GetAppsRequest) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$limit = this.getLimit();
final java.lang.Object other$limit = other.getLimit();
if (this$limit == null ? other$limit != null : !this$limit.equals(other$limit)) return false;
final java.lang.Object this$offset = this.getOffset();
final java.lang.Object other$offset = other.getOffset();
if (this$offset == null ? other$offset != null : !this$offset.equals(other$offset)) return false;
final java.lang.Object this$ids = this.getIds();
final java.lang.Object other$ids = other.getIds();
if (this$ids == null ? other$ids != null : !this$ids.equals(other$ids)) return false;
final java.lang.Object this$codes = this.getCodes();
final java.lang.Object other$codes = other.getCodes();
if (this$codes == null ? other$codes != null : !this$codes.equals(other$codes)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$spaceIds = this.getSpaceIds();
final java.lang.Object other$spaceIds = other.getSpaceIds();
if (this$spaceIds == null ? other$spaceIds != null : !this$spaceIds.equals(other$spaceIds)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof GetAppsRequest;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $limit = this.getLimit();
result = result * PRIME + ($limit == null ? 43 : $limit.hashCode());
final java.lang.Object $offset = this.getOffset();
result = result * PRIME + ($offset == null ? 43 : $offset.hashCode());
final java.lang.Object $ids = this.getIds();
result = result * PRIME + ($ids == null ? 43 : $ids.hashCode());
final java.lang.Object $codes = this.getCodes();
result = result * PRIME + ($codes == null ? 43 : $codes.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $spaceIds = this.getSpaceIds();
result = result * PRIME + ($spaceIds == null ? 43 : $spaceIds.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "GetAppsRequest(ids=" + this.getIds() + ", codes=" + this.getCodes() + ", name=" + this.getName() + ", spaceIds=" + this.getSpaceIds() + ", limit=" + this.getLimit() + ", offset=" + this.getOffset() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy