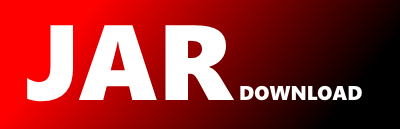
com.kintone.client.api.schema.GetApiSchemaResponseBody Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.api.schema;
import com.kintone.client.api.KintoneResponseBody;
import com.kintone.client.model.schema.RequestSchema;
import com.kintone.client.model.schema.ResponseSchema;
import com.kintone.client.model.schema.Schema;
import java.util.Map;
/**
* A response object for Get API Schema API.
*/
public final class GetApiSchemaResponseBody implements KintoneResponseBody {
/**
* The ID of the API.
*/
private final String id;
/**
* The base URL starting with "https://" that will be used with the API.
*/
private final String baseUrl;
/**
* The API path, such as "records.json".
*/
private final String path;
/**
* The HTTP method for the API
*/
private final String httpMethod;
/**
* The schema information for the API request, in a JSON Schema format.
*/
private final RequestSchema request;
/**
* The schema information for the API response, in a JSON Schema format.
*/
private final ResponseSchema response;
/**
* The schema information common within all APIs.
*/
private final Map schemas;
@java.beans.ConstructorProperties({"id", "baseUrl", "path", "httpMethod", "request", "response", "schemas"})
@java.lang.SuppressWarnings("all")
public GetApiSchemaResponseBody(final String id, final String baseUrl, final String path, final String httpMethod, final RequestSchema request, final ResponseSchema response, final Map schemas) {
this.id = id;
this.baseUrl = baseUrl;
this.path = path;
this.httpMethod = httpMethod;
this.request = request;
this.response = response;
this.schemas = schemas;
}
/**
* The ID of the API.
*/
@java.lang.SuppressWarnings("all")
public String getId() {
return this.id;
}
/**
* The base URL starting with "https://" that will be used with the API.
*/
@java.lang.SuppressWarnings("all")
public String getBaseUrl() {
return this.baseUrl;
}
/**
* The API path, such as "records.json".
*/
@java.lang.SuppressWarnings("all")
public String getPath() {
return this.path;
}
/**
* The HTTP method for the API
*/
@java.lang.SuppressWarnings("all")
public String getHttpMethod() {
return this.httpMethod;
}
/**
* The schema information for the API request, in a JSON Schema format.
*/
@java.lang.SuppressWarnings("all")
public RequestSchema getRequest() {
return this.request;
}
/**
* The schema information for the API response, in a JSON Schema format.
*/
@java.lang.SuppressWarnings("all")
public ResponseSchema getResponse() {
return this.response;
}
/**
* The schema information common within all APIs.
*/
@java.lang.SuppressWarnings("all")
public Map getSchemas() {
return this.schemas;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof GetApiSchemaResponseBody)) return false;
final GetApiSchemaResponseBody other = (GetApiSchemaResponseBody) o;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$baseUrl = this.getBaseUrl();
final java.lang.Object other$baseUrl = other.getBaseUrl();
if (this$baseUrl == null ? other$baseUrl != null : !this$baseUrl.equals(other$baseUrl)) return false;
final java.lang.Object this$path = this.getPath();
final java.lang.Object other$path = other.getPath();
if (this$path == null ? other$path != null : !this$path.equals(other$path)) return false;
final java.lang.Object this$httpMethod = this.getHttpMethod();
final java.lang.Object other$httpMethod = other.getHttpMethod();
if (this$httpMethod == null ? other$httpMethod != null : !this$httpMethod.equals(other$httpMethod)) return false;
final java.lang.Object this$request = this.getRequest();
final java.lang.Object other$request = other.getRequest();
if (this$request == null ? other$request != null : !this$request.equals(other$request)) return false;
final java.lang.Object this$response = this.getResponse();
final java.lang.Object other$response = other.getResponse();
if (this$response == null ? other$response != null : !this$response.equals(other$response)) return false;
final java.lang.Object this$schemas = this.getSchemas();
final java.lang.Object other$schemas = other.getSchemas();
if (this$schemas == null ? other$schemas != null : !this$schemas.equals(other$schemas)) return false;
return true;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $baseUrl = this.getBaseUrl();
result = result * PRIME + ($baseUrl == null ? 43 : $baseUrl.hashCode());
final java.lang.Object $path = this.getPath();
result = result * PRIME + ($path == null ? 43 : $path.hashCode());
final java.lang.Object $httpMethod = this.getHttpMethod();
result = result * PRIME + ($httpMethod == null ? 43 : $httpMethod.hashCode());
final java.lang.Object $request = this.getRequest();
result = result * PRIME + ($request == null ? 43 : $request.hashCode());
final java.lang.Object $response = this.getResponse();
result = result * PRIME + ($response == null ? 43 : $response.hashCode());
final java.lang.Object $schemas = this.getSchemas();
result = result * PRIME + ($schemas == null ? 43 : $schemas.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "GetApiSchemaResponseBody(id=" + this.getId() + ", baseUrl=" + this.getBaseUrl() + ", path=" + this.getPath() + ", httpMethod=" + this.getHttpMethod() + ", request=" + this.getRequest() + ", response=" + this.getResponse() + ", schemas=" + this.getSchemas() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy