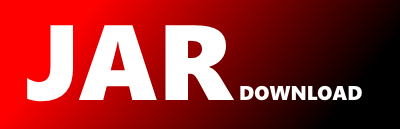
com.kintone.client.api.space.AddSpaceFromTemplateRequest Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.api.space;
import com.kintone.client.api.KintoneRequest;
import com.kintone.client.model.space.SpaceMember;
import java.util.List;
/**
* A request object for Add Space From Template API.
*/
public class AddSpaceFromTemplateRequest implements KintoneRequest {
/**
* The Space Template ID (required).
*/
private Long id;
/**
* The name of the Space (required).
*/
private String name;
/**
* A list of members of the Space (required).
*/
private List members;
/**
* The Space is a Private Space or not (optional).
*/
private Boolean isPrivate;
/**
* true to make the Space as a Guest Space (optional).
*/
private Boolean isGuest;
/**
* If true, users will not be able to join/leave the Space or follow/unfollow threads (optional).
*/
private Boolean fixedMember;
@java.lang.SuppressWarnings("all")
public AddSpaceFromTemplateRequest() {
}
/**
* The Space Template ID (required).
*/
@java.lang.SuppressWarnings("all")
public Long getId() {
return this.id;
}
/**
* The name of the Space (required).
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* A list of members of the Space (required).
*/
@java.lang.SuppressWarnings("all")
public List getMembers() {
return this.members;
}
/**
* The Space is a Private Space or not (optional).
*/
@java.lang.SuppressWarnings("all")
public Boolean getIsPrivate() {
return this.isPrivate;
}
/**
* true to make the Space as a Guest Space (optional).
*/
@java.lang.SuppressWarnings("all")
public Boolean getIsGuest() {
return this.isGuest;
}
/**
* If true, users will not be able to join/leave the Space or follow/unfollow threads (optional).
*/
@java.lang.SuppressWarnings("all")
public Boolean getFixedMember() {
return this.fixedMember;
}
/**
* The Space Template ID (required).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public AddSpaceFromTemplateRequest setId(final Long id) {
this.id = id;
return this;
}
/**
* The name of the Space (required).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public AddSpaceFromTemplateRequest setName(final String name) {
this.name = name;
return this;
}
/**
* A list of members of the Space (required).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public AddSpaceFromTemplateRequest setMembers(final List members) {
this.members = members;
return this;
}
/**
* The Space is a Private Space or not (optional).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public AddSpaceFromTemplateRequest setIsPrivate(final Boolean isPrivate) {
this.isPrivate = isPrivate;
return this;
}
/**
* true to make the Space as a Guest Space (optional).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public AddSpaceFromTemplateRequest setIsGuest(final Boolean isGuest) {
this.isGuest = isGuest;
return this;
}
/**
* If true, users will not be able to join/leave the Space or follow/unfollow threads (optional).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public AddSpaceFromTemplateRequest setFixedMember(final Boolean fixedMember) {
this.fixedMember = fixedMember;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof AddSpaceFromTemplateRequest)) return false;
final AddSpaceFromTemplateRequest other = (AddSpaceFromTemplateRequest) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$isPrivate = this.getIsPrivate();
final java.lang.Object other$isPrivate = other.getIsPrivate();
if (this$isPrivate == null ? other$isPrivate != null : !this$isPrivate.equals(other$isPrivate)) return false;
final java.lang.Object this$isGuest = this.getIsGuest();
final java.lang.Object other$isGuest = other.getIsGuest();
if (this$isGuest == null ? other$isGuest != null : !this$isGuest.equals(other$isGuest)) return false;
final java.lang.Object this$fixedMember = this.getFixedMember();
final java.lang.Object other$fixedMember = other.getFixedMember();
if (this$fixedMember == null ? other$fixedMember != null : !this$fixedMember.equals(other$fixedMember)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$members = this.getMembers();
final java.lang.Object other$members = other.getMembers();
if (this$members == null ? other$members != null : !this$members.equals(other$members)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof AddSpaceFromTemplateRequest;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $isPrivate = this.getIsPrivate();
result = result * PRIME + ($isPrivate == null ? 43 : $isPrivate.hashCode());
final java.lang.Object $isGuest = this.getIsGuest();
result = result * PRIME + ($isGuest == null ? 43 : $isGuest.hashCode());
final java.lang.Object $fixedMember = this.getFixedMember();
result = result * PRIME + ($fixedMember == null ? 43 : $fixedMember.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $members = this.getMembers();
result = result * PRIME + ($members == null ? 43 : $members.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "AddSpaceFromTemplateRequest(id=" + this.getId() + ", name=" + this.getName() + ", members=" + this.getMembers() + ", isPrivate=" + this.getIsPrivate() + ", isGuest=" + this.getIsGuest() + ", fixedMember=" + this.getFixedMember() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy