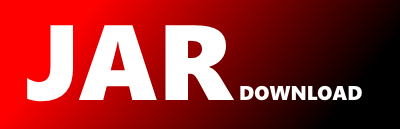
com.kintone.client.api.space.GetSpaceResponseBody Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.api.space;
import com.kintone.client.api.KintoneResponseBody;
import com.kintone.client.model.User;
import com.kintone.client.model.space.AttachedApp;
import com.kintone.client.model.space.CoverType;
import java.util.List;
/**
* A response object for Get Space API.
*/
public final class GetSpaceResponseBody implements KintoneResponseBody {
/**
* The Space ID.
*/
private final long id;
/**
* The name of the Space
*/
private final String name;
/**
* The Thread ID of the default thread that was created when the Space was made.
*/
private final long defaultThread;
/**
* The "Private" settings of the Space.
*/
private final boolean isPrivate;
/**
* An object containing information of the creator of the Space.
*/
private final User creator;
/**
* An object containing information of the updater of the Space.
*/
private final User modifier;
/**
* The image type of the Cover Photo.
*/
private final CoverType coverType;
/**
* The key of the Cover Photo.
*/
private final String coverKey;
/**
* The URL of the Cover Photo.
*/
private final String coverUrl;
/**
* The HTML of the Space body.
*/
private final String body;
/**
* A list of Apps that are in the thread.
*/
private final List attachedApps;
/**
* The number of members of the Space.
*/
private final long memberCount;
/**
* The "Enable multiple threads." setting.
*/
private final boolean useMultiThread;
/**
* The Guest Space setting.
*/
private final boolean isGuest;
/**
* The "Block users from joining or leaving the space and following or unfollowing the threads."
* setting.
*/
private final boolean fixedMember;
/**
* Whether the "Announcement" widget in the Space Portal page is shown.
*/
private final boolean showAnnouncement;
/**
* Whether the "Apps" widget in the Space Portal page is shown.
*/
private final boolean showAppList;
/**
* Whether the "People" widget in the Space Portal page is shown.
*/
private final boolean showMemberList;
/**
* Whether the "Threads" widget in the Space Portal page is shown.
*/
private final boolean showThreadList;
/**
* Whether the "Related Apps & Spaces" widget in the Space Portal page is shown.
*/
private final boolean showRelatedLinkList;
@java.beans.ConstructorProperties({"id", "name", "defaultThread", "isPrivate", "creator", "modifier", "coverType", "coverKey", "coverUrl", "body", "attachedApps", "memberCount", "useMultiThread", "isGuest", "fixedMember", "showAnnouncement", "showAppList", "showMemberList", "showThreadList", "showRelatedLinkList"})
@java.lang.SuppressWarnings("all")
public GetSpaceResponseBody(final long id, final String name, final long defaultThread, final boolean isPrivate, final User creator, final User modifier, final CoverType coverType, final String coverKey, final String coverUrl, final String body, final List attachedApps, final long memberCount, final boolean useMultiThread, final boolean isGuest, final boolean fixedMember, final boolean showAnnouncement, final boolean showAppList, final boolean showMemberList, final boolean showThreadList, final boolean showRelatedLinkList) {
this.id = id;
this.name = name;
this.defaultThread = defaultThread;
this.isPrivate = isPrivate;
this.creator = creator;
this.modifier = modifier;
this.coverType = coverType;
this.coverKey = coverKey;
this.coverUrl = coverUrl;
this.body = body;
this.attachedApps = attachedApps;
this.memberCount = memberCount;
this.useMultiThread = useMultiThread;
this.isGuest = isGuest;
this.fixedMember = fixedMember;
this.showAnnouncement = showAnnouncement;
this.showAppList = showAppList;
this.showMemberList = showMemberList;
this.showThreadList = showThreadList;
this.showRelatedLinkList = showRelatedLinkList;
}
/**
* The Space ID.
*/
@java.lang.SuppressWarnings("all")
public long getId() {
return this.id;
}
/**
* The name of the Space
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* The Thread ID of the default thread that was created when the Space was made.
*/
@java.lang.SuppressWarnings("all")
public long getDefaultThread() {
return this.defaultThread;
}
/**
* The "Private" settings of the Space.
*
* @return true if the Space is private.
*/
@java.lang.SuppressWarnings("all")
public boolean isPrivate() {
return this.isPrivate;
}
/**
* An object containing information of the creator of the Space.
*/
@java.lang.SuppressWarnings("all")
public User getCreator() {
return this.creator;
}
/**
* An object containing information of the updater of the Space.
*/
@java.lang.SuppressWarnings("all")
public User getModifier() {
return this.modifier;
}
/**
* The image type of the Cover Photo.
*/
@java.lang.SuppressWarnings("all")
public CoverType getCoverType() {
return this.coverType;
}
/**
* The key of the Cover Photo.
*/
@java.lang.SuppressWarnings("all")
public String getCoverKey() {
return this.coverKey;
}
/**
* The URL of the Cover Photo.
*/
@java.lang.SuppressWarnings("all")
public String getCoverUrl() {
return this.coverUrl;
}
/**
* The HTML of the Space body.
*/
@java.lang.SuppressWarnings("all")
public String getBody() {
return this.body;
}
/**
* A list of Apps that are in the thread.
*/
@java.lang.SuppressWarnings("all")
public List getAttachedApps() {
return this.attachedApps;
}
/**
* The number of members of the Space.
*/
@java.lang.SuppressWarnings("all")
public long getMemberCount() {
return this.memberCount;
}
/**
* The "Enable multiple threads." setting.
*
* @return true if the Space is a multi-threaded Space.
*/
@java.lang.SuppressWarnings("all")
public boolean isUseMultiThread() {
return this.useMultiThread;
}
/**
* The Guest Space setting.
*
* @return true if the Space is a Guest Space.
*/
@java.lang.SuppressWarnings("all")
public boolean isGuest() {
return this.isGuest;
}
/**
* The "Block users from joining or leaving the space and following or unfollowing the threads."
* setting.
*
* @return true if users cannot join/leave the Space or follow/unfollow threads.
*/
@java.lang.SuppressWarnings("all")
public boolean isFixedMember() {
return this.fixedMember;
}
/**
* Whether the "Announcement" widget in the Space Portal page is shown.
*
* @return true if the "Announcement" widget is shown.
*/
@java.lang.SuppressWarnings("all")
public boolean isShowAnnouncement() {
return this.showAnnouncement;
}
/**
* Whether the "Apps" widget in the Space Portal page is shown.
*
* @return true if the "Apps" widget is shown.
*/
@java.lang.SuppressWarnings("all")
public boolean isShowAppList() {
return this.showAppList;
}
/**
* Whether the "People" widget in the Space Portal page is shown.
*
* @return true if the "People" widget is shown.
*/
@java.lang.SuppressWarnings("all")
public boolean isShowMemberList() {
return this.showMemberList;
}
/**
* Whether the "Threads" widget in the Space Portal page is shown.
*
* @return true if the "Threads" widget is shown.
*/
@java.lang.SuppressWarnings("all")
public boolean isShowThreadList() {
return this.showThreadList;
}
/**
* Whether the "Related Apps & Spaces" widget in the Space Portal page is shown.
*
* @return true if the "Related Apps & Spaces" widget is shown.
*/
@java.lang.SuppressWarnings("all")
public boolean isShowRelatedLinkList() {
return this.showRelatedLinkList;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof GetSpaceResponseBody)) return false;
final GetSpaceResponseBody other = (GetSpaceResponseBody) o;
if (this.getId() != other.getId()) return false;
if (this.getDefaultThread() != other.getDefaultThread()) return false;
if (this.isPrivate() != other.isPrivate()) return false;
if (this.getMemberCount() != other.getMemberCount()) return false;
if (this.isUseMultiThread() != other.isUseMultiThread()) return false;
if (this.isGuest() != other.isGuest()) return false;
if (this.isFixedMember() != other.isFixedMember()) return false;
if (this.isShowAnnouncement() != other.isShowAnnouncement()) return false;
if (this.isShowAppList() != other.isShowAppList()) return false;
if (this.isShowMemberList() != other.isShowMemberList()) return false;
if (this.isShowThreadList() != other.isShowThreadList()) return false;
if (this.isShowRelatedLinkList() != other.isShowRelatedLinkList()) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$creator = this.getCreator();
final java.lang.Object other$creator = other.getCreator();
if (this$creator == null ? other$creator != null : !this$creator.equals(other$creator)) return false;
final java.lang.Object this$modifier = this.getModifier();
final java.lang.Object other$modifier = other.getModifier();
if (this$modifier == null ? other$modifier != null : !this$modifier.equals(other$modifier)) return false;
final java.lang.Object this$coverType = this.getCoverType();
final java.lang.Object other$coverType = other.getCoverType();
if (this$coverType == null ? other$coverType != null : !this$coverType.equals(other$coverType)) return false;
final java.lang.Object this$coverKey = this.getCoverKey();
final java.lang.Object other$coverKey = other.getCoverKey();
if (this$coverKey == null ? other$coverKey != null : !this$coverKey.equals(other$coverKey)) return false;
final java.lang.Object this$coverUrl = this.getCoverUrl();
final java.lang.Object other$coverUrl = other.getCoverUrl();
if (this$coverUrl == null ? other$coverUrl != null : !this$coverUrl.equals(other$coverUrl)) return false;
final java.lang.Object this$body = this.getBody();
final java.lang.Object other$body = other.getBody();
if (this$body == null ? other$body != null : !this$body.equals(other$body)) return false;
final java.lang.Object this$attachedApps = this.getAttachedApps();
final java.lang.Object other$attachedApps = other.getAttachedApps();
if (this$attachedApps == null ? other$attachedApps != null : !this$attachedApps.equals(other$attachedApps)) return false;
return true;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final long $id = this.getId();
result = result * PRIME + (int) ($id >>> 32 ^ $id);
final long $defaultThread = this.getDefaultThread();
result = result * PRIME + (int) ($defaultThread >>> 32 ^ $defaultThread);
result = result * PRIME + (this.isPrivate() ? 79 : 97);
final long $memberCount = this.getMemberCount();
result = result * PRIME + (int) ($memberCount >>> 32 ^ $memberCount);
result = result * PRIME + (this.isUseMultiThread() ? 79 : 97);
result = result * PRIME + (this.isGuest() ? 79 : 97);
result = result * PRIME + (this.isFixedMember() ? 79 : 97);
result = result * PRIME + (this.isShowAnnouncement() ? 79 : 97);
result = result * PRIME + (this.isShowAppList() ? 79 : 97);
result = result * PRIME + (this.isShowMemberList() ? 79 : 97);
result = result * PRIME + (this.isShowThreadList() ? 79 : 97);
result = result * PRIME + (this.isShowRelatedLinkList() ? 79 : 97);
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $creator = this.getCreator();
result = result * PRIME + ($creator == null ? 43 : $creator.hashCode());
final java.lang.Object $modifier = this.getModifier();
result = result * PRIME + ($modifier == null ? 43 : $modifier.hashCode());
final java.lang.Object $coverType = this.getCoverType();
result = result * PRIME + ($coverType == null ? 43 : $coverType.hashCode());
final java.lang.Object $coverKey = this.getCoverKey();
result = result * PRIME + ($coverKey == null ? 43 : $coverKey.hashCode());
final java.lang.Object $coverUrl = this.getCoverUrl();
result = result * PRIME + ($coverUrl == null ? 43 : $coverUrl.hashCode());
final java.lang.Object $body = this.getBody();
result = result * PRIME + ($body == null ? 43 : $body.hashCode());
final java.lang.Object $attachedApps = this.getAttachedApps();
result = result * PRIME + ($attachedApps == null ? 43 : $attachedApps.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "GetSpaceResponseBody(id=" + this.getId() + ", name=" + this.getName() + ", defaultThread=" + this.getDefaultThread() + ", isPrivate=" + this.isPrivate() + ", creator=" + this.getCreator() + ", modifier=" + this.getModifier() + ", coverType=" + this.getCoverType() + ", coverKey=" + this.getCoverKey() + ", coverUrl=" + this.getCoverUrl() + ", body=" + this.getBody() + ", attachedApps=" + this.getAttachedApps() + ", memberCount=" + this.getMemberCount() + ", useMultiThread=" + this.isUseMultiThread() + ", isGuest=" + this.isGuest() + ", fixedMember=" + this.isFixedMember() + ", showAnnouncement=" + this.isShowAnnouncement() + ", showAppList=" + this.isShowAppList() + ", showMemberList=" + this.isShowMemberList() + ", showThreadList=" + this.isShowThreadList() + ", showRelatedLinkList=" + this.isShowRelatedLinkList() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy