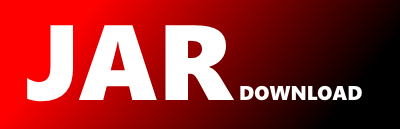
com.kintone.client.api.space.UpdateThreadRequest Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.api.space;
import com.kintone.client.api.KintoneRequest;
/**
* A request object for Update Thread API.
*/
public class UpdateThreadRequest implements KintoneRequest {
/**
* The Thread ID (required).
*/
private Long id;
/**
* The new name of the Thread (optional). The name will not be updated if this parameter is null.
*/
private String name;
/**
* The contents of the Thread body (optional). If set to null, leaves this setting unchanged.
*/
private String body;
@java.lang.SuppressWarnings("all")
public UpdateThreadRequest() {
}
/**
* The Thread ID (required).
*/
@java.lang.SuppressWarnings("all")
public Long getId() {
return this.id;
}
/**
* The new name of the Thread (optional). The name will not be updated if this parameter is null.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* The contents of the Thread body (optional). If set to null, leaves this setting unchanged.
*/
@java.lang.SuppressWarnings("all")
public String getBody() {
return this.body;
}
/**
* The Thread ID (required).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public UpdateThreadRequest setId(final Long id) {
this.id = id;
return this;
}
/**
* The new name of the Thread (optional). The name will not be updated if this parameter is null.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public UpdateThreadRequest setName(final String name) {
this.name = name;
return this;
}
/**
* The contents of the Thread body (optional). If set to null, leaves this setting unchanged.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public UpdateThreadRequest setBody(final String body) {
this.body = body;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof UpdateThreadRequest)) return false;
final UpdateThreadRequest other = (UpdateThreadRequest) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$body = this.getBody();
final java.lang.Object other$body = other.getBody();
if (this$body == null ? other$body != null : !this$body.equals(other$body)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof UpdateThreadRequest;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $body = this.getBody();
result = result * PRIME + ($body == null ? 43 : $body.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "UpdateThreadRequest(id=" + this.getId() + ", name=" + this.getName() + ", body=" + this.getBody() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy