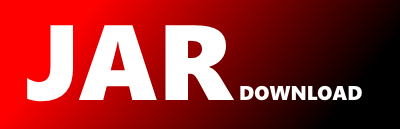
com.kintone.client.model.FileBody Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kintone-java-client Show documentation
Show all versions of kintone-java-client Show documentation
API client library for Kintone REST APIs on Java.
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model;
/**
* An object containing information of a saved file.
*/
public class FileBody {
/**
* The MIME type of the file.
*/
private String contentType;
/**
* The fileKey of the file.
*/
private String fileKey;
/**
* The file name of the file.
*/
private String name;
/**
* The byte size of the file.
*/
private Integer size;
@java.lang.SuppressWarnings("all")
public FileBody() {
}
/**
* The MIME type of the file.
*/
@java.lang.SuppressWarnings("all")
public String getContentType() {
return this.contentType;
}
/**
* The fileKey of the file.
*/
@java.lang.SuppressWarnings("all")
public String getFileKey() {
return this.fileKey;
}
/**
* The file name of the file.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* The byte size of the file.
*/
@java.lang.SuppressWarnings("all")
public Integer getSize() {
return this.size;
}
/**
* The MIME type of the file.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public FileBody setContentType(final String contentType) {
this.contentType = contentType;
return this;
}
/**
* The fileKey of the file.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public FileBody setFileKey(final String fileKey) {
this.fileKey = fileKey;
return this;
}
/**
* The file name of the file.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public FileBody setName(final String name) {
this.name = name;
return this;
}
/**
* The byte size of the file.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public FileBody setSize(final Integer size) {
this.size = size;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof FileBody)) return false;
final FileBody other = (FileBody) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$size = this.getSize();
final java.lang.Object other$size = other.getSize();
if (this$size == null ? other$size != null : !this$size.equals(other$size)) return false;
final java.lang.Object this$contentType = this.getContentType();
final java.lang.Object other$contentType = other.getContentType();
if (this$contentType == null ? other$contentType != null : !this$contentType.equals(other$contentType)) return false;
final java.lang.Object this$fileKey = this.getFileKey();
final java.lang.Object other$fileKey = other.getFileKey();
if (this$fileKey == null ? other$fileKey != null : !this$fileKey.equals(other$fileKey)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof FileBody;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $size = this.getSize();
result = result * PRIME + ($size == null ? 43 : $size.hashCode());
final java.lang.Object $contentType = this.getContentType();
result = result * PRIME + ($contentType == null ? 43 : $contentType.hashCode());
final java.lang.Object $fileKey = this.getFileKey();
result = result * PRIME + ($fileKey == null ? 43 : $fileKey.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "FileBody(contentType=" + this.getContentType() + ", fileKey=" + this.getFileKey() + ", name=" + this.getName() + ", size=" + this.getSize() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy