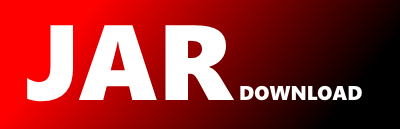
com.kintone.client.model.Organization Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model;
import java.beans.ConstructorProperties;
/**
* An object representing a Department value.
*/
public final class Organization {
/**
* The name of department.
*/
private final String name;
/**
* The code of department.
*/
private final String code;
/**
* Constructor to create a department value used for editing Department Selection fields. When
* adding or updating values of Department Selection field, the "name" parameter will be ignored.
* This constructor only sets the "code" field while leaves the "name" field empty.
*
* @param code the code of the department.
*/
public Organization(String code) {
this("", code);
}
@ConstructorProperties({"name", "code"})
public Organization(String name, String code) {
this.name = name;
this.code = code;
}
/**
* The name of department.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* The code of department.
*/
@java.lang.SuppressWarnings("all")
public String getCode() {
return this.code;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Organization)) return false;
final Organization other = (Organization) o;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$code = this.getCode();
final java.lang.Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
return true;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "Organization(name=" + this.getName() + ", code=" + this.getCode() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy