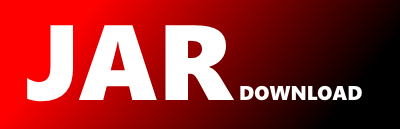
com.kintone.client.model.app.ProcessAction Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model.app;
/**
* Action settings information for getting and updating the Process Management Settings.
*/
public class ProcessAction {
/**
* The Action name.
*/
private String name;
/**
* The status before taking action.
*/
private String from;
/**
* The status after taking action.
*/
private String to;
/**
* The branch criteria of the action.
*/
private String filterCond;
@java.lang.SuppressWarnings("all")
public ProcessAction() {
}
/**
* The Action name.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* The status before taking action.
*/
@java.lang.SuppressWarnings("all")
public String getFrom() {
return this.from;
}
/**
* The status after taking action.
*/
@java.lang.SuppressWarnings("all")
public String getTo() {
return this.to;
}
/**
* The branch criteria of the action.
*/
@java.lang.SuppressWarnings("all")
public String getFilterCond() {
return this.filterCond;
}
/**
* The Action name.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ProcessAction setName(final String name) {
this.name = name;
return this;
}
/**
* The status before taking action.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ProcessAction setFrom(final String from) {
this.from = from;
return this;
}
/**
* The status after taking action.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ProcessAction setTo(final String to) {
this.to = to;
return this;
}
/**
* The branch criteria of the action.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ProcessAction setFilterCond(final String filterCond) {
this.filterCond = filterCond;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ProcessAction)) return false;
final ProcessAction other = (ProcessAction) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$from = this.getFrom();
final java.lang.Object other$from = other.getFrom();
if (this$from == null ? other$from != null : !this$from.equals(other$from)) return false;
final java.lang.Object this$to = this.getTo();
final java.lang.Object other$to = other.getTo();
if (this$to == null ? other$to != null : !this$to.equals(other$to)) return false;
final java.lang.Object this$filterCond = this.getFilterCond();
final java.lang.Object other$filterCond = other.getFilterCond();
if (this$filterCond == null ? other$filterCond != null : !this$filterCond.equals(other$filterCond)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ProcessAction;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $from = this.getFrom();
result = result * PRIME + ($from == null ? 43 : $from.hashCode());
final java.lang.Object $to = this.getTo();
result = result * PRIME + ($to == null ? 43 : $to.hashCode());
final java.lang.Object $filterCond = this.getFilterCond();
result = result * PRIME + ($filterCond == null ? 43 : $filterCond.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "ProcessAction(name=" + this.getName() + ", from=" + this.getFrom() + ", to=" + this.getTo() + ", filterCond=" + this.getFilterCond() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy