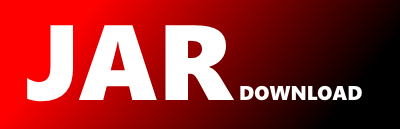
com.kintone.client.model.app.ProcessEntity Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model.app;
import com.kintone.client.model.Entity;
/**
* An entity in Assignee settings information.
*/
public class ProcessEntity {
/**
* An object containing user data of the Assignees.
*/
private Entity entity;
/**
* The "Include affiliated departments" settings of the department.
*/
private Boolean includeSubs;
@java.lang.SuppressWarnings("all")
public ProcessEntity() {
}
/**
* An object containing user data of the Assignees.
*/
@java.lang.SuppressWarnings("all")
public Entity getEntity() {
return this.entity;
}
/**
* The "Include affiliated departments" settings of the department.
*
* @return true if affiliated departments are included as Assignees
*/
@java.lang.SuppressWarnings("all")
public Boolean getIncludeSubs() {
return this.includeSubs;
}
/**
* An object containing user data of the Assignees.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ProcessEntity setEntity(final Entity entity) {
this.entity = entity;
return this;
}
/**
* The "Include affiliated departments" settings of the department.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ProcessEntity setIncludeSubs(final Boolean includeSubs) {
this.includeSubs = includeSubs;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ProcessEntity)) return false;
final ProcessEntity other = (ProcessEntity) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$includeSubs = this.getIncludeSubs();
final java.lang.Object other$includeSubs = other.getIncludeSubs();
if (this$includeSubs == null ? other$includeSubs != null : !this$includeSubs.equals(other$includeSubs)) return false;
final java.lang.Object this$entity = this.getEntity();
final java.lang.Object other$entity = other.getEntity();
if (this$entity == null ? other$entity != null : !this$entity.equals(other$entity)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ProcessEntity;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $includeSubs = this.getIncludeSubs();
result = result * PRIME + ($includeSubs == null ? 43 : $includeSubs.hashCode());
final java.lang.Object $entity = this.getEntity();
result = result * PRIME + ($entity == null ? 43 : $entity.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "ProcessEntity(entity=" + this.getEntity() + ", includeSubs=" + this.getIncludeSubs() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy