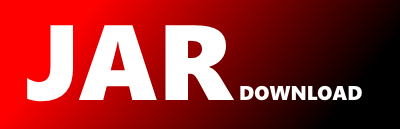
com.kintone.client.model.app.ReminderNotification Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model.app;
import java.util.List;
/**
* An object representing the reminder notification settings.
*/
public class ReminderNotification {
/**
* The notification subject.
*/
private String title;
/**
* The record's filter condition in query string format.
*/
private String filterCond;
/**
* An object containing the Reminder notification's timing.
*/
private ReminderTiming timing;
/**
* An array of objects containing the recipients of the Reminder Notification.
*/
private List targets;
@java.lang.SuppressWarnings("all")
public ReminderNotification() {
}
/**
* The notification subject.
*/
@java.lang.SuppressWarnings("all")
public String getTitle() {
return this.title;
}
/**
* The record's filter condition in query string format.
*/
@java.lang.SuppressWarnings("all")
public String getFilterCond() {
return this.filterCond;
}
/**
* An object containing the Reminder notification's timing.
*/
@java.lang.SuppressWarnings("all")
public ReminderTiming getTiming() {
return this.timing;
}
/**
* An array of objects containing the recipients of the Reminder Notification.
*/
@java.lang.SuppressWarnings("all")
public List getTargets() {
return this.targets;
}
/**
* The notification subject.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ReminderNotification setTitle(final String title) {
this.title = title;
return this;
}
/**
* The record's filter condition in query string format.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ReminderNotification setFilterCond(final String filterCond) {
this.filterCond = filterCond;
return this;
}
/**
* An object containing the Reminder notification's timing.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ReminderNotification setTiming(final ReminderTiming timing) {
this.timing = timing;
return this;
}
/**
* An array of objects containing the recipients of the Reminder Notification.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ReminderNotification setTargets(final List targets) {
this.targets = targets;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ReminderNotification)) return false;
final ReminderNotification other = (ReminderNotification) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$title = this.getTitle();
final java.lang.Object other$title = other.getTitle();
if (this$title == null ? other$title != null : !this$title.equals(other$title)) return false;
final java.lang.Object this$filterCond = this.getFilterCond();
final java.lang.Object other$filterCond = other.getFilterCond();
if (this$filterCond == null ? other$filterCond != null : !this$filterCond.equals(other$filterCond)) return false;
final java.lang.Object this$timing = this.getTiming();
final java.lang.Object other$timing = other.getTiming();
if (this$timing == null ? other$timing != null : !this$timing.equals(other$timing)) return false;
final java.lang.Object this$targets = this.getTargets();
final java.lang.Object other$targets = other.getTargets();
if (this$targets == null ? other$targets != null : !this$targets.equals(other$targets)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ReminderNotification;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $title = this.getTitle();
result = result * PRIME + ($title == null ? 43 : $title.hashCode());
final java.lang.Object $filterCond = this.getFilterCond();
result = result * PRIME + ($filterCond == null ? 43 : $filterCond.hashCode());
final java.lang.Object $timing = this.getTiming();
result = result * PRIME + ($timing == null ? 43 : $timing.hashCode());
final java.lang.Object $targets = this.getTargets();
result = result * PRIME + ($targets == null ? 43 : $targets.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "ReminderNotification(title=" + this.getTitle() + ", filterCond=" + this.getFilterCond() + ", timing=" + this.getTiming() + ", targets=" + this.getTargets() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy