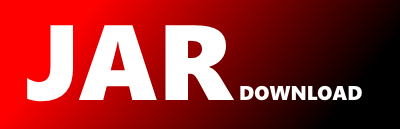
com.kintone.client.model.app.ReminderTiming Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model.app;
/**
* An object representing the settings of reminder timing.
*/
public class ReminderTiming {
/**
* The field code of the field used to determine the Reminder notification's timing.
*/
private String code;
/**
* The number of days after the {@link #code} filed value (date or datetime) when the Reminder
* notification is sent. A negative value indicates the number of days before the {@link #code}
* filed value.
*/
private Integer daysLater;
/**
* The number of hours after the {@link #code} filed value (datetime), shifted by daysLater, when
* the Reminder notification is sent. A negative value indicates the number of hours before the
* {@link #code} field value, shifted by daysLater.
*
* In the response of get reminder notification settings API, this parameter is returned only
* if the {@link #code} parameter is set to a Date and Time field and the "When" hours
* before/after option is configured (instead of the "At" time option).
*/
private Integer hoursLater;
/**
* The time when the Reminder notification is sent.
*
*
In the response of get reminder notification settings API, this parameter is returned if the
* {@link #code} parameter is set to a date field or the "At" time option is configured.
*/
private String time;
@java.lang.SuppressWarnings("all")
public ReminderTiming() {
}
/**
* The field code of the field used to determine the Reminder notification's timing.
*/
@java.lang.SuppressWarnings("all")
public String getCode() {
return this.code;
}
/**
* The number of days after the {@link #code} filed value (date or datetime) when the Reminder
* notification is sent. A negative value indicates the number of days before the {@link #code}
* filed value.
*/
@java.lang.SuppressWarnings("all")
public Integer getDaysLater() {
return this.daysLater;
}
/**
* The number of hours after the {@link #code} filed value (datetime), shifted by daysLater, when
* the Reminder notification is sent. A negative value indicates the number of hours before the
* {@link #code} field value, shifted by daysLater.
*
*
In the response of get reminder notification settings API, this parameter is returned only
* if the {@link #code} parameter is set to a Date and Time field and the "When" hours
* before/after option is configured (instead of the "At" time option).
*/
@java.lang.SuppressWarnings("all")
public Integer getHoursLater() {
return this.hoursLater;
}
/**
* The time when the Reminder notification is sent.
*
*
In the response of get reminder notification settings API, this parameter is returned if the
* {@link #code} parameter is set to a date field or the "At" time option is configured.
*/
@java.lang.SuppressWarnings("all")
public String getTime() {
return this.time;
}
/**
* The field code of the field used to determine the Reminder notification's timing.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ReminderTiming setCode(final String code) {
this.code = code;
return this;
}
/**
* The number of days after the {@link #code} filed value (date or datetime) when the Reminder
* notification is sent. A negative value indicates the number of days before the {@link #code}
* filed value.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ReminderTiming setDaysLater(final Integer daysLater) {
this.daysLater = daysLater;
return this;
}
/**
* The number of hours after the {@link #code} filed value (datetime), shifted by daysLater, when
* the Reminder notification is sent. A negative value indicates the number of hours before the
* {@link #code} field value, shifted by daysLater.
*
*
In the response of get reminder notification settings API, this parameter is returned only
* if the {@link #code} parameter is set to a Date and Time field and the "When" hours
* before/after option is configured (instead of the "At" time option).
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ReminderTiming setHoursLater(final Integer hoursLater) {
this.hoursLater = hoursLater;
return this;
}
/**
* The time when the Reminder notification is sent.
*
*
In the response of get reminder notification settings API, this parameter is returned if the
* {@link #code} parameter is set to a date field or the "At" time option is configured.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ReminderTiming setTime(final String time) {
this.time = time;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ReminderTiming)) return false;
final ReminderTiming other = (ReminderTiming) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$daysLater = this.getDaysLater();
final java.lang.Object other$daysLater = other.getDaysLater();
if (this$daysLater == null ? other$daysLater != null : !this$daysLater.equals(other$daysLater)) return false;
final java.lang.Object this$hoursLater = this.getHoursLater();
final java.lang.Object other$hoursLater = other.getHoursLater();
if (this$hoursLater == null ? other$hoursLater != null : !this$hoursLater.equals(other$hoursLater)) return false;
final java.lang.Object this$code = this.getCode();
final java.lang.Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final java.lang.Object this$time = this.getTime();
final java.lang.Object other$time = other.getTime();
if (this$time == null ? other$time != null : !this$time.equals(other$time)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ReminderTiming;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $daysLater = this.getDaysLater();
result = result * PRIME + ($daysLater == null ? 43 : $daysLater.hashCode());
final java.lang.Object $hoursLater = this.getHoursLater();
result = result * PRIME + ($hoursLater == null ? 43 : $hoursLater.hashCode());
final java.lang.Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final java.lang.Object $time = this.getTime();
result = result * PRIME + ($time == null ? 43 : $time.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "ReminderTiming(code=" + this.getCode() + ", daysLater=" + this.getDaysLater() + ", hoursLater=" + this.getHoursLater() + ", time=" + this.getTime() + ")";
}
}