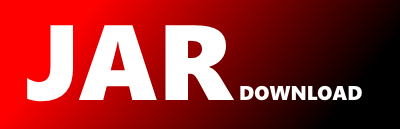
com.kintone.client.model.app.View Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kintone-java-client Show documentation
Show all versions of kintone-java-client Show documentation
API client library for Kintone REST APIs on Java.
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model.app;
import java.util.List;
/**
* An object containing settings of an App view.
*/
public class View {
/**
* The View ID.
*/
private Long id;
/**
* The type of View.
*/
private ViewType type;
/**
* The type of the built-in View.
*/
private BuiltinType builtinType;
/**
* The name of the View.
*/
private String name;
/**
* Used for List Views.
*/
private List fields;
/**
* The filter condition in a query format.
*/
private String filterCond;
/**
* The sort order in a query format.
*/
private String sort;
/**
* The display order of the View, in the list of views, specified with a number.
*/
private Long index;
/**
* Used for Calendar views.
*/
private String title;
/**
* Used for Calendar Views.
*/
private String date;
/**
* Used for Custom Views.
*/
private String html;
/**
* The scope of where the view is displayed.
*/
private Device device;
/**
* The pagination settings. Specify one of the following:
*/
private Boolean pager;
@java.lang.SuppressWarnings("all")
public View() {
}
/**
* The View ID.
*/
@java.lang.SuppressWarnings("all")
public Long getId() {
return this.id;
}
/**
* The type of View.
*/
@java.lang.SuppressWarnings("all")
public ViewType getType() {
return this.type;
}
/**
* The type of the built-in View.
*/
@java.lang.SuppressWarnings("all")
public BuiltinType getBuiltinType() {
return this.builtinType;
}
/**
* The name of the View.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* Used for List Views.
*/
@java.lang.SuppressWarnings("all")
public List getFields() {
return this.fields;
}
/**
* The filter condition in a query format.
*/
@java.lang.SuppressWarnings("all")
public String getFilterCond() {
return this.filterCond;
}
/**
* The sort order in a query format.
*/
@java.lang.SuppressWarnings("all")
public String getSort() {
return this.sort;
}
/**
* The display order of the View, in the list of views, specified with a number.
*/
@java.lang.SuppressWarnings("all")
public Long getIndex() {
return this.index;
}
/**
* Used for Calendar views.
*/
@java.lang.SuppressWarnings("all")
public String getTitle() {
return this.title;
}
/**
* Used for Calendar Views.
*/
@java.lang.SuppressWarnings("all")
public String getDate() {
return this.date;
}
/**
* Used for Custom Views.
*/
@java.lang.SuppressWarnings("all")
public String getHtml() {
return this.html;
}
/**
* The scope of where the view is displayed.
*/
@java.lang.SuppressWarnings("all")
public Device getDevice() {
return this.device;
}
/**
* The pagination settings. Specify one of the following:
*
* @return true if enable (default)
*/
@java.lang.SuppressWarnings("all")
public Boolean getPager() {
return this.pager;
}
/**
* The View ID.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setId(final Long id) {
this.id = id;
return this;
}
/**
* The type of View.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setType(final ViewType type) {
this.type = type;
return this;
}
/**
* The type of the built-in View.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setBuiltinType(final BuiltinType builtinType) {
this.builtinType = builtinType;
return this;
}
/**
* The name of the View.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setName(final String name) {
this.name = name;
return this;
}
/**
* Used for List Views.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setFields(final List fields) {
this.fields = fields;
return this;
}
/**
* The filter condition in a query format.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setFilterCond(final String filterCond) {
this.filterCond = filterCond;
return this;
}
/**
* The sort order in a query format.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setSort(final String sort) {
this.sort = sort;
return this;
}
/**
* The display order of the View, in the list of views, specified with a number.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setIndex(final Long index) {
this.index = index;
return this;
}
/**
* Used for Calendar views.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setTitle(final String title) {
this.title = title;
return this;
}
/**
* Used for Calendar Views.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setDate(final String date) {
this.date = date;
return this;
}
/**
* Used for Custom Views.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setHtml(final String html) {
this.html = html;
return this;
}
/**
* The scope of where the view is displayed.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setDevice(final Device device) {
this.device = device;
return this;
}
/**
* The pagination settings. Specify one of the following:
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public View setPager(final Boolean pager) {
this.pager = pager;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof View)) return false;
final View other = (View) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$index = this.getIndex();
final java.lang.Object other$index = other.getIndex();
if (this$index == null ? other$index != null : !this$index.equals(other$index)) return false;
final java.lang.Object this$pager = this.getPager();
final java.lang.Object other$pager = other.getPager();
if (this$pager == null ? other$pager != null : !this$pager.equals(other$pager)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$builtinType = this.getBuiltinType();
final java.lang.Object other$builtinType = other.getBuiltinType();
if (this$builtinType == null ? other$builtinType != null : !this$builtinType.equals(other$builtinType)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$fields = this.getFields();
final java.lang.Object other$fields = other.getFields();
if (this$fields == null ? other$fields != null : !this$fields.equals(other$fields)) return false;
final java.lang.Object this$filterCond = this.getFilterCond();
final java.lang.Object other$filterCond = other.getFilterCond();
if (this$filterCond == null ? other$filterCond != null : !this$filterCond.equals(other$filterCond)) return false;
final java.lang.Object this$sort = this.getSort();
final java.lang.Object other$sort = other.getSort();
if (this$sort == null ? other$sort != null : !this$sort.equals(other$sort)) return false;
final java.lang.Object this$title = this.getTitle();
final java.lang.Object other$title = other.getTitle();
if (this$title == null ? other$title != null : !this$title.equals(other$title)) return false;
final java.lang.Object this$date = this.getDate();
final java.lang.Object other$date = other.getDate();
if (this$date == null ? other$date != null : !this$date.equals(other$date)) return false;
final java.lang.Object this$html = this.getHtml();
final java.lang.Object other$html = other.getHtml();
if (this$html == null ? other$html != null : !this$html.equals(other$html)) return false;
final java.lang.Object this$device = this.getDevice();
final java.lang.Object other$device = other.getDevice();
if (this$device == null ? other$device != null : !this$device.equals(other$device)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof View;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $index = this.getIndex();
result = result * PRIME + ($index == null ? 43 : $index.hashCode());
final java.lang.Object $pager = this.getPager();
result = result * PRIME + ($pager == null ? 43 : $pager.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $builtinType = this.getBuiltinType();
result = result * PRIME + ($builtinType == null ? 43 : $builtinType.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $fields = this.getFields();
result = result * PRIME + ($fields == null ? 43 : $fields.hashCode());
final java.lang.Object $filterCond = this.getFilterCond();
result = result * PRIME + ($filterCond == null ? 43 : $filterCond.hashCode());
final java.lang.Object $sort = this.getSort();
result = result * PRIME + ($sort == null ? 43 : $sort.hashCode());
final java.lang.Object $title = this.getTitle();
result = result * PRIME + ($title == null ? 43 : $title.hashCode());
final java.lang.Object $date = this.getDate();
result = result * PRIME + ($date == null ? 43 : $date.hashCode());
final java.lang.Object $html = this.getHtml();
result = result * PRIME + ($html == null ? 43 : $html.hashCode());
final java.lang.Object $device = this.getDevice();
result = result * PRIME + ($device == null ? 43 : $device.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "View(id=" + this.getId() + ", type=" + this.getType() + ", builtinType=" + this.getBuiltinType() + ", name=" + this.getName() + ", fields=" + this.getFields() + ", filterCond=" + this.getFilterCond() + ", sort=" + this.getSort() + ", index=" + this.getIndex() + ", title=" + this.getTitle() + ", date=" + this.getDate() + ", html=" + this.getHtml() + ", device=" + this.getDevice() + ", pager=" + this.getPager() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy