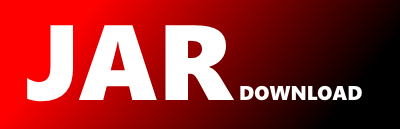
com.kintone.client.model.app.field.CalcFieldProperty Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model.app.field;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.kintone.client.model.record.FieldType;
/**
* An object containing the properties of a Calculated field for getting and setting the field
* settings.
*/
@JsonIgnoreProperties(value = "type", allowGetters = true)
public class CalcFieldProperty implements FieldProperty {
/**
* The field code of the field.
*/
private String code;
/**
* The field name.
*/
private String label;
/**
* The "Hide field name" option.
*/
private Boolean noLabel;
/**
* The "Required field" option.
*/
private Boolean required;
/**
* The formula expression used in the field.
*/
private String expression;
/**
* The display format for fields with calculations
*/
private DisplayFormat format;
/**
* The number of decimal places to display for the field.
*/
private Long displayScale;
/**
* The "Hide formula" settings for the field.
*/
private Boolean hideExpression;
/**
* The currency settings of the field.
*/
private String unit;
/**
* The display position of the currency.
*/
private UnitPosition unitPosition;
/**
* {@inheritDoc}
*/
@Override
public FieldType getType() {
return FieldType.CALC;
}
@java.lang.SuppressWarnings("all")
public CalcFieldProperty() {
}
/**
* The field code of the field.
*/
@java.lang.SuppressWarnings("all")
public String getCode() {
return this.code;
}
/**
* The field name.
*/
@java.lang.SuppressWarnings("all")
public String getLabel() {
return this.label;
}
/**
* The "Hide field name" option.
*
* @return true if the field's name will be hidden
*/
@java.lang.SuppressWarnings("all")
public Boolean getNoLabel() {
return this.noLabel;
}
/**
* The "Required field" option.
*
* @return true if the field will be a required field.
*/
@java.lang.SuppressWarnings("all")
public Boolean getRequired() {
return this.required;
}
/**
* The formula expression used in the field.
*/
@java.lang.SuppressWarnings("all")
public String getExpression() {
return this.expression;
}
/**
* The display format for fields with calculations
*/
@java.lang.SuppressWarnings("all")
public DisplayFormat getFormat() {
return this.format;
}
/**
* The number of decimal places to display for the field.
*/
@java.lang.SuppressWarnings("all")
public Long getDisplayScale() {
return this.displayScale;
}
/**
* The "Hide formula" settings for the field.
*
* @return true if the formula will be hidden.
*/
@java.lang.SuppressWarnings("all")
public Boolean getHideExpression() {
return this.hideExpression;
}
/**
* The currency settings of the field.
*/
@java.lang.SuppressWarnings("all")
public String getUnit() {
return this.unit;
}
/**
* The display position of the currency.
*/
@java.lang.SuppressWarnings("all")
public UnitPosition getUnitPosition() {
return this.unitPosition;
}
/**
* The field code of the field.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public CalcFieldProperty setCode(final String code) {
this.code = code;
return this;
}
/**
* The field name.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public CalcFieldProperty setLabel(final String label) {
this.label = label;
return this;
}
/**
* The "Hide field name" option.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public CalcFieldProperty setNoLabel(final Boolean noLabel) {
this.noLabel = noLabel;
return this;
}
/**
* The "Required field" option.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public CalcFieldProperty setRequired(final Boolean required) {
this.required = required;
return this;
}
/**
* The formula expression used in the field.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public CalcFieldProperty setExpression(final String expression) {
this.expression = expression;
return this;
}
/**
* The display format for fields with calculations
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public CalcFieldProperty setFormat(final DisplayFormat format) {
this.format = format;
return this;
}
/**
* The number of decimal places to display for the field.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public CalcFieldProperty setDisplayScale(final Long displayScale) {
this.displayScale = displayScale;
return this;
}
/**
* The "Hide formula" settings for the field.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public CalcFieldProperty setHideExpression(final Boolean hideExpression) {
this.hideExpression = hideExpression;
return this;
}
/**
* The currency settings of the field.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public CalcFieldProperty setUnit(final String unit) {
this.unit = unit;
return this;
}
/**
* The display position of the currency.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public CalcFieldProperty setUnitPosition(final UnitPosition unitPosition) {
this.unitPosition = unitPosition;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof CalcFieldProperty)) return false;
final CalcFieldProperty other = (CalcFieldProperty) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$noLabel = this.getNoLabel();
final java.lang.Object other$noLabel = other.getNoLabel();
if (this$noLabel == null ? other$noLabel != null : !this$noLabel.equals(other$noLabel)) return false;
final java.lang.Object this$required = this.getRequired();
final java.lang.Object other$required = other.getRequired();
if (this$required == null ? other$required != null : !this$required.equals(other$required)) return false;
final java.lang.Object this$displayScale = this.getDisplayScale();
final java.lang.Object other$displayScale = other.getDisplayScale();
if (this$displayScale == null ? other$displayScale != null : !this$displayScale.equals(other$displayScale)) return false;
final java.lang.Object this$hideExpression = this.getHideExpression();
final java.lang.Object other$hideExpression = other.getHideExpression();
if (this$hideExpression == null ? other$hideExpression != null : !this$hideExpression.equals(other$hideExpression)) return false;
final java.lang.Object this$code = this.getCode();
final java.lang.Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final java.lang.Object this$label = this.getLabel();
final java.lang.Object other$label = other.getLabel();
if (this$label == null ? other$label != null : !this$label.equals(other$label)) return false;
final java.lang.Object this$expression = this.getExpression();
final java.lang.Object other$expression = other.getExpression();
if (this$expression == null ? other$expression != null : !this$expression.equals(other$expression)) return false;
final java.lang.Object this$format = this.getFormat();
final java.lang.Object other$format = other.getFormat();
if (this$format == null ? other$format != null : !this$format.equals(other$format)) return false;
final java.lang.Object this$unit = this.getUnit();
final java.lang.Object other$unit = other.getUnit();
if (this$unit == null ? other$unit != null : !this$unit.equals(other$unit)) return false;
final java.lang.Object this$unitPosition = this.getUnitPosition();
final java.lang.Object other$unitPosition = other.getUnitPosition();
if (this$unitPosition == null ? other$unitPosition != null : !this$unitPosition.equals(other$unitPosition)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof CalcFieldProperty;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $noLabel = this.getNoLabel();
result = result * PRIME + ($noLabel == null ? 43 : $noLabel.hashCode());
final java.lang.Object $required = this.getRequired();
result = result * PRIME + ($required == null ? 43 : $required.hashCode());
final java.lang.Object $displayScale = this.getDisplayScale();
result = result * PRIME + ($displayScale == null ? 43 : $displayScale.hashCode());
final java.lang.Object $hideExpression = this.getHideExpression();
result = result * PRIME + ($hideExpression == null ? 43 : $hideExpression.hashCode());
final java.lang.Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final java.lang.Object $label = this.getLabel();
result = result * PRIME + ($label == null ? 43 : $label.hashCode());
final java.lang.Object $expression = this.getExpression();
result = result * PRIME + ($expression == null ? 43 : $expression.hashCode());
final java.lang.Object $format = this.getFormat();
result = result * PRIME + ($format == null ? 43 : $format.hashCode());
final java.lang.Object $unit = this.getUnit();
result = result * PRIME + ($unit == null ? 43 : $unit.hashCode());
final java.lang.Object $unitPosition = this.getUnitPosition();
result = result * PRIME + ($unitPosition == null ? 43 : $unitPosition.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "CalcFieldProperty(code=" + this.getCode() + ", label=" + this.getLabel() + ", noLabel=" + this.getNoLabel() + ", required=" + this.getRequired() + ", expression=" + this.getExpression() + ", format=" + this.getFormat() + ", displayScale=" + this.getDisplayScale() + ", hideExpression=" + this.getHideExpression() + ", unit=" + this.getUnit() + ", unitPosition=" + this.getUnitPosition() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy