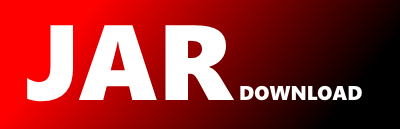
com.kintone.client.model.app.field.GroupFieldProperty Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model.app.field;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.kintone.client.model.record.FieldType;
/**
* An object containing the properties of a Group field for getting and setting the field settings.
*/
@JsonIgnoreProperties(value = "type", allowGetters = true)
public class GroupFieldProperty implements FieldProperty {
/**
* The field code of the field.
*/
private String code;
/**
* The field name.
*/
private String label;
/**
* The "Hide field name" option.
*/
private Boolean noLabel;
/**
* The "Show fields in this group" option.
*/
private Boolean openGroup;
/**
* {@inheritDoc}
*/
@Override
public FieldType getType() {
return FieldType.GROUP;
}
@java.lang.SuppressWarnings("all")
public GroupFieldProperty() {
}
/**
* The field code of the field.
*/
@java.lang.SuppressWarnings("all")
public String getCode() {
return this.code;
}
/**
* The field name.
*/
@java.lang.SuppressWarnings("all")
public String getLabel() {
return this.label;
}
/**
* The "Hide field name" option.
*
* @return true if the field's name will be hidden
*/
@java.lang.SuppressWarnings("all")
public Boolean getNoLabel() {
return this.noLabel;
}
/**
* The "Show fields in this group" option.
*
* @return true if the Group field will be displayed open by default.
*/
@java.lang.SuppressWarnings("all")
public Boolean getOpenGroup() {
return this.openGroup;
}
/**
* The field code of the field.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GroupFieldProperty setCode(final String code) {
this.code = code;
return this;
}
/**
* The field name.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GroupFieldProperty setLabel(final String label) {
this.label = label;
return this;
}
/**
* The "Hide field name" option.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GroupFieldProperty setNoLabel(final Boolean noLabel) {
this.noLabel = noLabel;
return this;
}
/**
* The "Show fields in this group" option.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GroupFieldProperty setOpenGroup(final Boolean openGroup) {
this.openGroup = openGroup;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof GroupFieldProperty)) return false;
final GroupFieldProperty other = (GroupFieldProperty) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$noLabel = this.getNoLabel();
final java.lang.Object other$noLabel = other.getNoLabel();
if (this$noLabel == null ? other$noLabel != null : !this$noLabel.equals(other$noLabel)) return false;
final java.lang.Object this$openGroup = this.getOpenGroup();
final java.lang.Object other$openGroup = other.getOpenGroup();
if (this$openGroup == null ? other$openGroup != null : !this$openGroup.equals(other$openGroup)) return false;
final java.lang.Object this$code = this.getCode();
final java.lang.Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final java.lang.Object this$label = this.getLabel();
final java.lang.Object other$label = other.getLabel();
if (this$label == null ? other$label != null : !this$label.equals(other$label)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof GroupFieldProperty;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $noLabel = this.getNoLabel();
result = result * PRIME + ($noLabel == null ? 43 : $noLabel.hashCode());
final java.lang.Object $openGroup = this.getOpenGroup();
result = result * PRIME + ($openGroup == null ? 43 : $openGroup.hashCode());
final java.lang.Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final java.lang.Object $label = this.getLabel();
result = result * PRIME + ($label == null ? 43 : $label.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "GroupFieldProperty(code=" + this.getCode() + ", label=" + this.getLabel() + ", noLabel=" + this.getNoLabel() + ", openGroup=" + this.getOpenGroup() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy