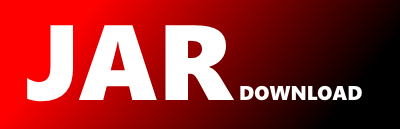
com.kintone.client.model.app.report.EveryQuarterPeriod Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model.app.report;
import java.time.LocalTime;
public class EveryQuarterPeriod implements PeriodicReportPeriod {
private static final String END_OF_MONTH = "END_OF_MONTH";
/**
* The months when the quarterly Periodic Report will be generated.
*/
private QuarterlyPattern pattern;
/**
* The day when the Periodic Report will be generated. The day is returned as an integer, ranging
* from 1 to 31, or set as "END_OF_MONTH".
*/
private String dayOfMonth;
/**
* The time when the Periodic Report will be generated.
*/
private LocalTime time;
/**
* {@inheritDoc}
*/
@Override
public IntervalType getEvery() {
return IntervalType.QUARTER;
}
/**
* Checks whether the day when the Periodic Report will be generated is set to "END_OF_MONTH".
*
* @return returns true if the day when the Periodic Report will be generated is set to
* "END_OF_MONTH".
*/
public boolean isEndOfMonth() {
return END_OF_MONTH.equals(dayOfMonth);
}
/**
* Gets the setting of day when the Periodic Report will be generated.
*
* @return the day when the Periodic Report will be generated. If the setting is not set or set as
* "END_OF_MONTH", the result is null.
*/
public Integer getDayOfMonth() {
if (dayOfMonth == null || isEndOfMonth()) {
return null;
}
return Integer.valueOf(dayOfMonth);
}
/**
* Sets the setting of day when the Periodic Report will be generated. To set the setting to
* "END_OF_MONTH", use {@link #setEndOfMonth()}.
*
* @param dayOfMonth the day of Month
* @return this object
*/
public EveryQuarterPeriod setDayOfMonth(int dayOfMonth) {
this.dayOfMonth = Integer.toString(dayOfMonth);
return this;
}
/**
* Sets the setting of day when the Periodic Report will be generated to "END_OF_MONTH".
*
* @return this object
*/
public EveryQuarterPeriod setEndOfMonth() {
this.dayOfMonth = END_OF_MONTH;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "EveryQuarterPeriod(pattern=" + this.getPattern() + ", dayOfMonth=" + this.getDayOfMonth() + ", time=" + this.getTime() + ")";
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof EveryQuarterPeriod)) return false;
final EveryQuarterPeriod other = (EveryQuarterPeriod) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$pattern = this.getPattern();
final java.lang.Object other$pattern = other.getPattern();
if (this$pattern == null ? other$pattern != null : !this$pattern.equals(other$pattern)) return false;
final java.lang.Object this$dayOfMonth = this.getDayOfMonth();
final java.lang.Object other$dayOfMonth = other.getDayOfMonth();
if (this$dayOfMonth == null ? other$dayOfMonth != null : !this$dayOfMonth.equals(other$dayOfMonth)) return false;
final java.lang.Object this$time = this.getTime();
final java.lang.Object other$time = other.getTime();
if (this$time == null ? other$time != null : !this$time.equals(other$time)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof EveryQuarterPeriod;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $pattern = this.getPattern();
result = result * PRIME + ($pattern == null ? 43 : $pattern.hashCode());
final java.lang.Object $dayOfMonth = this.getDayOfMonth();
result = result * PRIME + ($dayOfMonth == null ? 43 : $dayOfMonth.hashCode());
final java.lang.Object $time = this.getTime();
result = result * PRIME + ($time == null ? 43 : $time.hashCode());
return result;
}
/**
* The months when the quarterly Periodic Report will be generated.
*/
@java.lang.SuppressWarnings("all")
public QuarterlyPattern getPattern() {
return this.pattern;
}
/**
* The months when the quarterly Periodic Report will be generated.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public EveryQuarterPeriod setPattern(final QuarterlyPattern pattern) {
this.pattern = pattern;
return this;
}
/**
* The time when the Periodic Report will be generated.
*/
@java.lang.SuppressWarnings("all")
public LocalTime getTime() {
return this.time;
}
/**
* The time when the Periodic Report will be generated.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public EveryQuarterPeriod setTime(final LocalTime time) {
this.time = time;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy