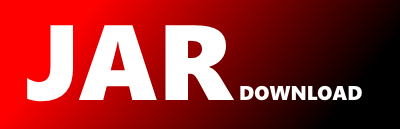
com.kintone.client.model.app.report.Report Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model.app.report;
import java.util.List;
/**
* An object containing settings of a Graph.
*/
public class Report {
/**
* The ID of the graph.
*
* This field is ignored for the request object of Update Graphs API.
*/
private Long id;
/**
* The chart type of the graph.
*/
private ChartType chartType;
/**
* The display mode of the graph.
*/
private ChartMode chartMode;
/**
* The name of the graph.
*/
private String name;
/**
* The order of the graph.
*/
private Long index;
/**
* An array of objects containing the "Group by" options.
*/
private List groups;
/**
* An array of objects containing the "Function" options.
*/
private List aggregations;
/**
* The filter condition in a query format.
*/
private String filterCond;
/**
* An array of objects containing the "Sort by" options.
*/
private List sorts;
/**
* An objects containing the "Periodic Reports" options.
*/
private PeriodicReport periodicReport;
@java.lang.SuppressWarnings("all")
public Report() {
}
/**
* The ID of the graph.
*
* This field is ignored for the request object of Update Graphs API.
*/
@java.lang.SuppressWarnings("all")
public Long getId() {
return this.id;
}
/**
* The chart type of the graph.
*/
@java.lang.SuppressWarnings("all")
public ChartType getChartType() {
return this.chartType;
}
/**
* The display mode of the graph.
*/
@java.lang.SuppressWarnings("all")
public ChartMode getChartMode() {
return this.chartMode;
}
/**
* The name of the graph.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* The order of the graph.
*/
@java.lang.SuppressWarnings("all")
public Long getIndex() {
return this.index;
}
/**
* An array of objects containing the "Group by" options.
*/
@java.lang.SuppressWarnings("all")
public List getGroups() {
return this.groups;
}
/**
* An array of objects containing the "Function" options.
*/
@java.lang.SuppressWarnings("all")
public List getAggregations() {
return this.aggregations;
}
/**
* The filter condition in a query format.
*/
@java.lang.SuppressWarnings("all")
public String getFilterCond() {
return this.filterCond;
}
/**
* An array of objects containing the "Sort by" options.
*/
@java.lang.SuppressWarnings("all")
public List getSorts() {
return this.sorts;
}
/**
* An objects containing the "Periodic Reports" options.
*/
@java.lang.SuppressWarnings("all")
public PeriodicReport getPeriodicReport() {
return this.periodicReport;
}
/**
* The ID of the graph.
*
* This field is ignored for the request object of Update Graphs API.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Report setId(final Long id) {
this.id = id;
return this;
}
/**
* The chart type of the graph.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Report setChartType(final ChartType chartType) {
this.chartType = chartType;
return this;
}
/**
* The display mode of the graph.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Report setChartMode(final ChartMode chartMode) {
this.chartMode = chartMode;
return this;
}
/**
* The name of the graph.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Report setName(final String name) {
this.name = name;
return this;
}
/**
* The order of the graph.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Report setIndex(final Long index) {
this.index = index;
return this;
}
/**
* An array of objects containing the "Group by" options.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Report setGroups(final List groups) {
this.groups = groups;
return this;
}
/**
* An array of objects containing the "Function" options.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Report setAggregations(final List aggregations) {
this.aggregations = aggregations;
return this;
}
/**
* The filter condition in a query format.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Report setFilterCond(final String filterCond) {
this.filterCond = filterCond;
return this;
}
/**
* An array of objects containing the "Sort by" options.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Report setSorts(final List sorts) {
this.sorts = sorts;
return this;
}
/**
* An objects containing the "Periodic Reports" options.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Report setPeriodicReport(final PeriodicReport periodicReport) {
this.periodicReport = periodicReport;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Report)) return false;
final Report other = (Report) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$index = this.getIndex();
final java.lang.Object other$index = other.getIndex();
if (this$index == null ? other$index != null : !this$index.equals(other$index)) return false;
final java.lang.Object this$chartType = this.getChartType();
final java.lang.Object other$chartType = other.getChartType();
if (this$chartType == null ? other$chartType != null : !this$chartType.equals(other$chartType)) return false;
final java.lang.Object this$chartMode = this.getChartMode();
final java.lang.Object other$chartMode = other.getChartMode();
if (this$chartMode == null ? other$chartMode != null : !this$chartMode.equals(other$chartMode)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$groups = this.getGroups();
final java.lang.Object other$groups = other.getGroups();
if (this$groups == null ? other$groups != null : !this$groups.equals(other$groups)) return false;
final java.lang.Object this$aggregations = this.getAggregations();
final java.lang.Object other$aggregations = other.getAggregations();
if (this$aggregations == null ? other$aggregations != null : !this$aggregations.equals(other$aggregations)) return false;
final java.lang.Object this$filterCond = this.getFilterCond();
final java.lang.Object other$filterCond = other.getFilterCond();
if (this$filterCond == null ? other$filterCond != null : !this$filterCond.equals(other$filterCond)) return false;
final java.lang.Object this$sorts = this.getSorts();
final java.lang.Object other$sorts = other.getSorts();
if (this$sorts == null ? other$sorts != null : !this$sorts.equals(other$sorts)) return false;
final java.lang.Object this$periodicReport = this.getPeriodicReport();
final java.lang.Object other$periodicReport = other.getPeriodicReport();
if (this$periodicReport == null ? other$periodicReport != null : !this$periodicReport.equals(other$periodicReport)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Report;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $index = this.getIndex();
result = result * PRIME + ($index == null ? 43 : $index.hashCode());
final java.lang.Object $chartType = this.getChartType();
result = result * PRIME + ($chartType == null ? 43 : $chartType.hashCode());
final java.lang.Object $chartMode = this.getChartMode();
result = result * PRIME + ($chartMode == null ? 43 : $chartMode.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $groups = this.getGroups();
result = result * PRIME + ($groups == null ? 43 : $groups.hashCode());
final java.lang.Object $aggregations = this.getAggregations();
result = result * PRIME + ($aggregations == null ? 43 : $aggregations.hashCode());
final java.lang.Object $filterCond = this.getFilterCond();
result = result * PRIME + ($filterCond == null ? 43 : $filterCond.hashCode());
final java.lang.Object $sorts = this.getSorts();
result = result * PRIME + ($sorts == null ? 43 : $sorts.hashCode());
final java.lang.Object $periodicReport = this.getPeriodicReport();
result = result * PRIME + ($periodicReport == null ? 43 : $periodicReport.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "Report(id=" + this.getId() + ", chartType=" + this.getChartType() + ", chartMode=" + this.getChartMode() + ", name=" + this.getName() + ", index=" + this.getIndex() + ", groups=" + this.getGroups() + ", aggregations=" + this.getAggregations() + ", filterCond=" + this.getFilterCond() + ", sorts=" + this.getSorts() + ", periodicReport=" + this.getPeriodicReport() + ")";
}
}