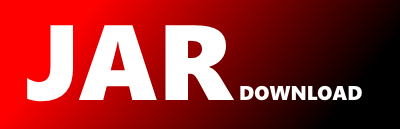
com.kintone.client.model.space.AddedSpaceMember Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model.space;
import com.kintone.client.model.Entity;
/**
* A Space member information retrieved by Get Space Members API.
*/
public final class AddedSpaceMember {
/**
* The entity information of the Space member.
*/
private final Entity entity;
/**
* The Space Admin settings of the Space member
*/
private final boolean isAdmin;
/**
* The "Include Affiliated Departments" setting of the Department Space Member.
*/
private final boolean includeSubs;
/**
* If the Space Member is added as a User or not.
*/
private final boolean isImplicit;
@java.beans.ConstructorProperties({"entity", "isAdmin", "includeSubs", "isImplicit"})
@java.lang.SuppressWarnings("all")
public AddedSpaceMember(final Entity entity, final boolean isAdmin, final boolean includeSubs, final boolean isImplicit) {
this.entity = entity;
this.isAdmin = isAdmin;
this.includeSubs = includeSubs;
this.isImplicit = isImplicit;
}
/**
* The entity information of the Space member.
*/
@java.lang.SuppressWarnings("all")
public Entity getEntity() {
return this.entity;
}
/**
* The Space Admin settings of the Space member
*
* @return true if the Space Member is the Space Administrator.
*/
@java.lang.SuppressWarnings("all")
public boolean isAdmin() {
return this.isAdmin;
}
/**
* The "Include Affiliated Departments" setting of the Department Space Member.
*/
@java.lang.SuppressWarnings("all")
public boolean isIncludeSubs() {
return this.includeSubs;
}
/**
* If the Space Member is added as a User or not.
*/
@java.lang.SuppressWarnings("all")
public boolean isImplicit() {
return this.isImplicit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof AddedSpaceMember)) return false;
final AddedSpaceMember other = (AddedSpaceMember) o;
if (this.isAdmin() != other.isAdmin()) return false;
if (this.isIncludeSubs() != other.isIncludeSubs()) return false;
if (this.isImplicit() != other.isImplicit()) return false;
final java.lang.Object this$entity = this.getEntity();
final java.lang.Object other$entity = other.getEntity();
if (this$entity == null ? other$entity != null : !this$entity.equals(other$entity)) return false;
return true;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + (this.isAdmin() ? 79 : 97);
result = result * PRIME + (this.isIncludeSubs() ? 79 : 97);
result = result * PRIME + (this.isImplicit() ? 79 : 97);
final java.lang.Object $entity = this.getEntity();
result = result * PRIME + ($entity == null ? 43 : $entity.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "AddedSpaceMember(entity=" + this.getEntity() + ", isAdmin=" + this.isAdmin() + ", includeSubs=" + this.isIncludeSubs() + ", isImplicit=" + this.isImplicit() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy