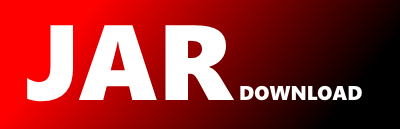
com.kintone.client.model.space.ThreadComment Maven / Gradle / Ivy
// Generated by delombok at Fri Jan 21 13:34:53 JST 2022
package com.kintone.client.model.space;
import com.kintone.client.model.Entity;
import java.util.List;
/**
* An object containing thread comment settings to be posted using Add Thread Comment API.
*/
public class ThreadComment {
/**
* The comment contents.
*/
private String text;
/**
* A list of mentioned users, groups or departments, that notify other kintone users.
*/
private List mentions;
/**
* A list of attachment files.
*/
private List files;
@java.lang.SuppressWarnings("all")
public ThreadComment() {
}
/**
* The comment contents.
*/
@java.lang.SuppressWarnings("all")
public String getText() {
return this.text;
}
/**
* A list of mentioned users, groups or departments, that notify other kintone users.
*/
@java.lang.SuppressWarnings("all")
public List getMentions() {
return this.mentions;
}
/**
* A list of attachment files.
*/
@java.lang.SuppressWarnings("all")
public List getFiles() {
return this.files;
}
/**
* The comment contents.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ThreadComment setText(final String text) {
this.text = text;
return this;
}
/**
* A list of mentioned users, groups or departments, that notify other kintone users.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ThreadComment setMentions(final List mentions) {
this.mentions = mentions;
return this;
}
/**
* A list of attachment files.
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public ThreadComment setFiles(final List files) {
this.files = files;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ThreadComment)) return false;
final ThreadComment other = (ThreadComment) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$text = this.getText();
final java.lang.Object other$text = other.getText();
if (this$text == null ? other$text != null : !this$text.equals(other$text)) return false;
final java.lang.Object this$mentions = this.getMentions();
final java.lang.Object other$mentions = other.getMentions();
if (this$mentions == null ? other$mentions != null : !this$mentions.equals(other$mentions)) return false;
final java.lang.Object this$files = this.getFiles();
final java.lang.Object other$files = other.getFiles();
if (this$files == null ? other$files != null : !this$files.equals(other$files)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ThreadComment;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $text = this.getText();
result = result * PRIME + ($text == null ? 43 : $text.hashCode());
final java.lang.Object $mentions = this.getMentions();
result = result * PRIME + ($mentions == null ? 43 : $mentions.hashCode());
final java.lang.Object $files = this.getFiles();
result = result * PRIME + ($files == null ? 43 : $files.hashCode());
return result;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "ThreadComment(text=" + this.getText() + ", mentions=" + this.getMentions() + ", files=" + this.getFiles() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy