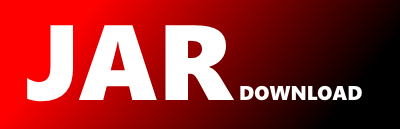
com.kncept.junit5.reporter.xml.Junit4DomReader Maven / Gradle / Ivy
package com.kncept.junit5.reporter.xml;
import static com.kncept.junit5.reporter.domain.TestCaseStatus.Errored;
import static com.kncept.junit5.reporter.domain.TestCaseStatus.Failed;
import static com.kncept.junit5.reporter.domain.TestCaseStatus.Passed;
import static com.kncept.junit5.reporter.domain.TestCaseStatus.Skipped;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
import com.kncept.junit5.reporter.domain.TestCase;
import com.kncept.junit5.reporter.domain.TestCaseStatus;
public class Junit4DomReader implements XMLTestResults {
private LinkedHashMap systemProperties = new LinkedHashMap<>();
private LinkedHashMap testsuiteProperties = new LinkedHashMap<>();
private List testcases = new ArrayList<>();
public Junit4DomReader(InputStream in) throws ParserConfigurationException, SAXException, IOException {
DocumentBuilder builder = DocumentBuilderFactory.newInstance().newDocumentBuilder();
Document doc = builder.parse(in);
NamedNodeMap testsuiteAttrs = doc.getDocumentElement().getAttributes();
if (testsuiteAttrs != null) for(int i = 0; i < testsuiteAttrs.getLength(); i++) {
Node attr = testsuiteAttrs.item(i);
testsuiteProperties.put(attr.getNodeName(), attr.getNodeValue());
}
NodeList nl = doc.getElementsByTagName("property");
for(int i = 0; i < nl.getLength(); i++) {
Node node = nl.item(i);
systemProperties.put(attr(node, "name"), attr(node, "value"));
}
nl = doc.getElementsByTagName("testcase");
for(int i = 0; i < nl.getLength(); i++) {
Node node = nl.item(i);
TestCaseStatus status = Passed; //default output is success.
String unsuccessfulMessage = null;
String stackTrace = null;
Node statusNode = child(node, "skipped");
//
if (statusNode != null) {
status = Skipped;
unsuccessfulMessage = attr(statusNode, "message");
stackTrace = statusNode.getTextContent();
}
statusNode = child(node, "failure");
// handleTextNode(Node node) {
List lines = new ArrayList<>();
if (node != null) {
NodeList nl = node.getChildNodes();
for(int i = 0; i < nl.getLength(); i++) try {
Node child = nl.item(i);
//switch to BufferedReader rather than string split to handle unix/windows cross platform rendering
BufferedReader bIn = new BufferedReader(new StringReader(child.getTextContent()));
String line = bIn.readLine();
while (line != null) {
lines.add(line);
line = bIn.readLine();
}
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return lines;
}
public LinkedHashMap systemProperties() {
return systemProperties;
}
public LinkedHashMap testsuiteProperties() {
return testsuiteProperties;
}
public List testcases() {
return testcases;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy