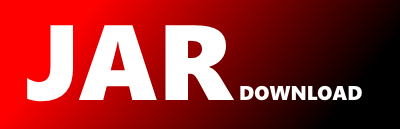
com.kolibrifx.common.collections.Maps Maven / Gradle / Ivy
/*
* Copyright (c) 2010-2017, KolibriFX AS. Licensed under the Apache License, version 2.0.
*/
package com.kolibrifx.common.collections;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.function.Supplier;
public final class Maps {
private Map map;
private Maps(final Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy